Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial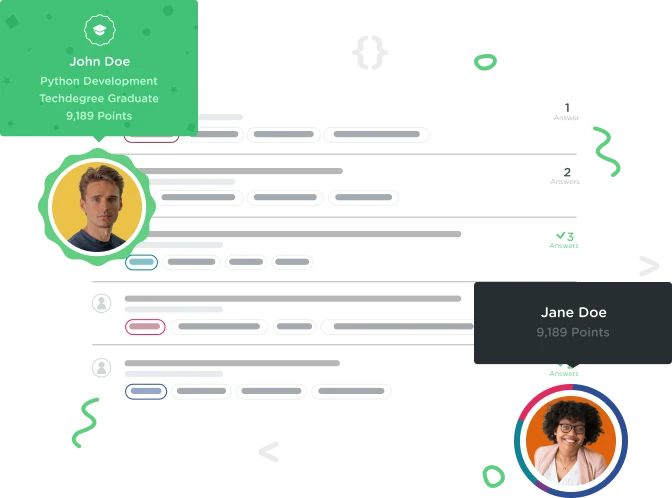
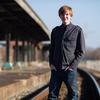
Jeffrey Green
1,733 PointsJava Tester bugged on "Add Tags to a Course" code challenge under the "Java Data Structures" course.
If you're going through the Java Data Structures course, you come across the second code challenge under the "MVP" section named "Add Tags to a Course." The first task asks you to initialize the member set of tags in the constructor. The problem though is if you add another parameter for the constructor to accept a list or set of "tags" to initialize the set with, the Java Tester throws up an error because it's trying to use a single parameter. And if you try to initialize the set of "tags" without a parameter by simply using ".add()" to the set it will give you a null pointer exception. I've even tried method signatures and created a second constructor that accepts two, while leaving the first constructor alone, and the task will ask if I've initialized the set of "tags." I believe the Java Tester is not performing the correct tests or something is bugged with the code challenge and task.
package com.example.model;
import java.util.List;
import java.util.Set;
import java.util.HashSet;
public class Course {
private String mTitle;
private Set<String> mTags;
public Course(String title, List<String> tags) {
mTitle = title;
// TODO: initialize the set mTags
mTags = new HashSet<String>(tags);
}
public Course(String title) {
mTitle = title;
mTags.add("New Tag");
}
public void addTag(String tag) {
// TODO: add the tag
}
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
}
public boolean hasTag(String tag) {
// TODO: Return whether or not the tag has been added
return false;
}
public String getTitle() {
return mTitle;
}
}
1 Answer

Seth Kroger
56,413 PointsAdding the tags to the course is going to be a subsequent step. You're not going to change the signature of the constructor, you're only going to initialize an empty set of tags.
Jeffrey Green
1,733 PointsJeffrey Green
1,733 PointsOkay, so that works, I guess the issue I was having was why I would initialize it to be empty when I would accept a list of tags in the constructor to begin with.