Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial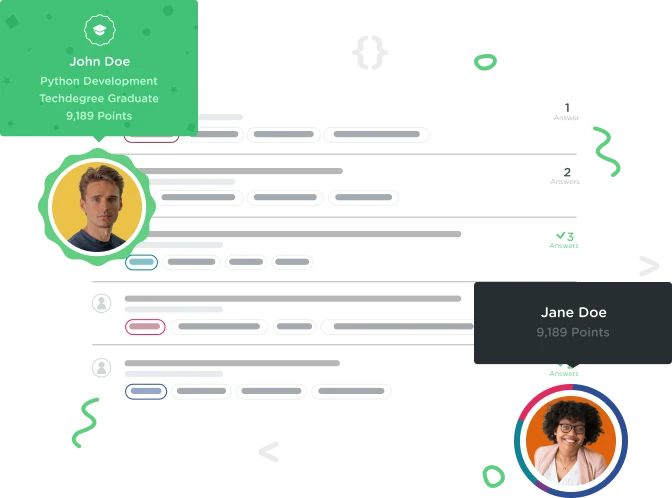
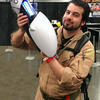
John Grillo
30,241 PointsJava Wrap-up part 4---compiler error with forum
I'm attempting to solve the problems with regard to main.java in part 4 of the java wrap up, but can't quite figure out what the compiler is trying to tell me. It says something wrong with the Forum section, but I can't quite see what the problem is.
Thank you.
public class Forum {
private String topic;
// TODO: add a constructor that accepts a topic and sets the private field topic
public String forum(String topic) {
this.topic = topic;
}
public String getTopic() {
return topic;
}
// Uncomment this when you are prompted to do so
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
//
}
public class User {
// TODO: add private fields for firstName and lastName
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
// TODO: set and add the private fields
this.firstName = firstName;
this.lastName = lastName;
}
// TODO: add getters for firstName and lastName
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
}
public class ForumPost {
private User author;
private String title;
private String description;
// TODO: add a constructor that accepts the author, title and description
public ForumPost(User author, String title, String description) {
this.Author = author;
this.title = title;
this.Description = description;
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
// Uncomment this when prompted
Forum forum = new Forum("Java");
// TODO: pass in the first name and last name that are in the args parameter
User author = new User(args[0], args[1]);
// TODO: initialize the forum post with the user created above and a title and description of your choice
ForumPost post = new ForumPost(author, "my first post--how to learn Java", "Best way to learn Java?");
forum.addPost(post);
}
}
3 Answers

Jake Goodman
3,381 PointsJava is case-sensitive. You wrote this in ForumPost.java
this.Author = author;
this.title = title;
this.Description = description;
but it should be:
this.author = author;
this.title = title;
this.description = description;
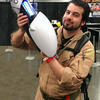
John Grillo
30,241 PointsGrrr....It didn't copy/paste! This is the error message. ./Main.java:11: error: constructor Forum in class Forum cannot be applied to given types; Forum forum = new Forum("Java"); ^ required: no arguments found: String reason: actual and formal argument lists differ in length Note: JavaTester.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. 1 error
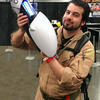
John Grillo
30,241 PointsThank you! Caught and updated. But for some reason, it's claiming the "new forum" argument is off. I've updated my original post.
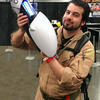
John Grillo
30,241 PointsThe error message in question:
./Main.java:11: error: constructor Forum in class Forum cannot be applied to given types;
Forum forum = new Forum("Java");
^
required: no arguments
found: String
reason: actual and formal argument lists differ in length
Note: JavaTester.java uses unchecked or unsafe operations.
Note: Recompile with -Xlint:unchecked for details.
1 error

Ben Reynolds
35,170 PointsIn forum.java you've set up your constructor like this:
public String forum(String topic)
This is how you'd set up a regular method, but constructors don't have return types (the "string" part).
- Remove "String" from the constructor
- Capitalize Forum to match the class name

Ben Reynolds
35,170 PointsClarification, just the first "String" can be removed from that line; the second one in the parameters before "topic" is correct.
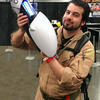
John Grillo
30,241 PointsSo wait, like this:
public Forum(String topic) {
this.topic = topic;
return topic; [ <-- update:this line needed to be removed. ]
}
Why remove the first "String"? I thought a datatype was needed for each constructor?

Ben Reynolds
35,170 PointsRemove the return statement and you should be good to go. Since there's no return type in the constructor, no need for the return statement.

Ben Reynolds
35,170 PointsWhy remove the first "String"? I thought a datatype was needed for each constructor?
Nope, constructors are a special type of method that are only used to create an instance of a class and set some default values if you want it to, such as the topic variable in this case (instantiation is the process that's kicked off when you use the "new" keyword). That's why the line with the "new" keyword was throwing the error, it didn't know what to do when it jumped to the constructor and saw a return type there.
Ben Reynolds
35,170 PointsBen Reynolds
35,170 PointsDid you modify any code from an earlier step? I think i see what's going on but task 1 wouldn't have passed if I'm right. Can you also share the text of the error message? That will help narrow it down. Thanks!