Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial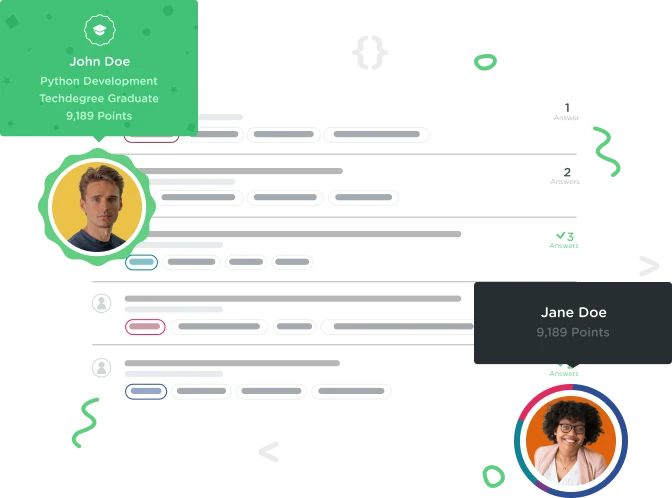

John Saad
20,199 PointsJavaFX Question
Hello I'm having some trouble with setting the initial date for the DatePicker control in JavaFX. (This has not been reviewed in the class but I am trying to build something myself)
When I code everything in main it works fine:
VBox vbox = new VBox(20);
Scene scene = new Scene(vbox, 400, 400);
primaryStage.setScene(scene);
DatePicker startDatePicker = new DatePicker();
startDatePicker.setValue(LocalDate.now());
vbox.getChildren().add(startDatePicker);
but the trouble starts when I try to separate the layout and use the controller. I cannot bind text to the DatePicker in the .fxml file so I try to set it in the controller by doing the following:
public class Controller {
@FXML
private DatePicker datePicker;
public Controller() {
datePicker.setValue(LocalDate.now());
}
}
(Only relevant code is shown in both instances)
However, this does not work and I have been trying to make sense of it all by reading https://docs.oracle.com/javase/8/javafx/api/javafx/scene/control/DatePicker.html but am having not luck.
A push in the right direction would be greatly appreciated!!

John Saad
20,199 PointsThe issue I am having is that the datepicker control is empty (no initial date) because there is no initialized value. Basically, I do not know how to initialize the value of datepicker using the controller, but I know how to do it in main.
I'd like to do it in the controller because all of my other controls are in the .fxml file and I don't want to have to code everything in main.
Does my question make sense?

Seth Kroger
56,413 PointsI asked about the FXML because I think it could be relevant. What you posted for Java code looks like it should work so I wondering if the issue is in the FXML code instead.
4 Answers

Seth Kroger
56,413 PointsOK, I've been trying it out myself and the issue I get is the dread NullPointerException for the DatePicker. From what I've been able to piece together, you need to use the Initializable interface to set up a default date, not the constructor.
import javafx.fxml.FXML;
import javafx.fxml.Initializable;
import javafx.scene.control.DatePicker;
// ...other imports... yay for IDEs!
public class Controller implements Initializable {
@FXML private DatePicker pickADate;
@Override
public void initialize(URL location, ResourceBundle resources) {
pickADate.setValue(LocalDate.now().plusDays(3));
}
}

Seth Kroger
56,413 PointsIt can also be simplified in newer versions of JavaFX by the @FXML annotation on a method named initialize():
public class Controller {
@FXML private DatePicker pickADate;
@FXML
public void initialize() {
pickADate.setValue(LocalDate.now().plusDays(3));
}
}
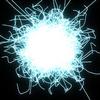
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey There, I had to do something like this recently, if the variable has already been set you can use the setConverter method and pass the time into the argument. Here's the two things I found online.
This is to set it upon initialization of the variable (I found this to be the easiest.)
Creating upon intialization of variable
The below uses the set Converter method to format a current date in if the variable has already been delcared Setting if variable has already been set and declared

John Saad
20,199 PointsThanks for the response Rob.
I saw the link you provided before you responded as I was doing some researching.
public class PickerDemo extends Application {
@Override public void start(Stage stage) {
final DatePicker datePicker = new DatePicker(LocalDate.now());
datePicker.setOnAction(event -> {
LocalDate date = datePicker.getValue();
System.out.println("Selected date: " + date);
});
stage.setScene(
new Scene(datePicker)
);
stage.show();
}
public static void main(String[] args) { launch(args); }
}
This does not help because this initializes datepicker in the main application instead of using the controller. I would prefer not to do it this way because all of my other controls, labels, text boxes are declared in the .fxml file.
I try to set the datePicker variable in the controller constructor as shown in the original question but it does not work for some reason. Do I need to override a class or something? I think I'm missing something...
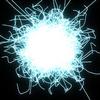
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHi John,
Unfortunately LocalDate object has no default constructor, so there's no way to set the value in FXML. This is true for a lot of items in JavaFX, they have to be set in your controller or application, then they can be arranged in the FXML.
However, the example shown to set it in the app then move it around in your FXML should have worked. Is it just populating correctly? What exactly is it doing.

John Saad
20,199 PointsThanks Seth that worked great!
Seth Kroger
56,413 PointsSeth Kroger
56,413 PointsWhat about your FXML file, and what sort of issue are you having? Is it not rendering, or not setting the correct date?