Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial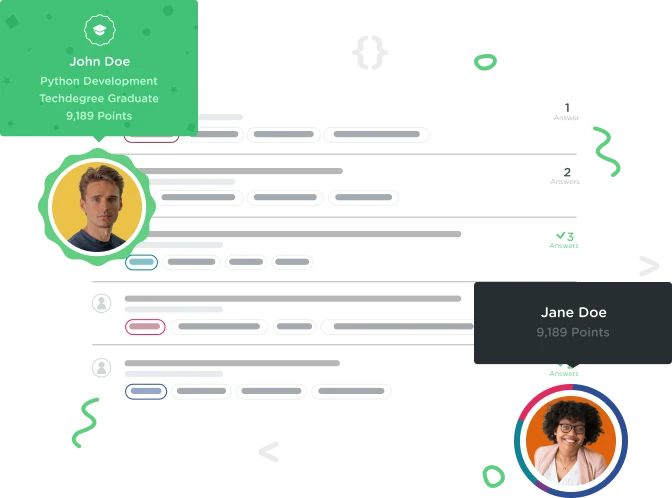

Malcolm Mutambanengwe
4,205 Pointsjava.lang.NullPointerException (Look around MealActivity.java line 16)
What does java.lang.NullPointerException mean and how can I make the background color green?
import android.os.Bundle;
import android.widget.TextView;
public class MealActivity extends Activity {
private RelativeLayout mRelativeLayout;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_meal);
TextView foodLabel = (TextView) findViewById(R.id.foodTextView);
TextView drinkLabel = (TextView) findViewById(R.id.drinkTextView);
RelativeLayout mealLayout = (RelativeLayout) findViewById(R.id.mealLayout);
mRelativeLayout.setBackgroundColor(Color.GREEN);
}
}
2 Answers
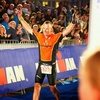
Steve Hunter
57,712 PointsHi Malcolm,
Technically, what Gunjeet has posted is the reason for your NullPointerException. However, you need to reverse the variable names to pass the challenge as the challenge provides you with a RelativeLayout
called mealLayout
. The test is expecting the background colour of a variable called mealLayout
to equal Color.GREEN
.
You need to add one line only:
public void onCreate(Bundle savedInstanceState) {
// provided code
.
.
RelativeLayout mealLayout = (RelativeLayout) findViewById(R.id.mealLayout); // provided
// your line below
mealLayout.setBackgroundColor(Color.GREEN);
}
I hope that helps,
Steve.
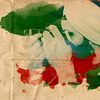
Gunjeet Hattar
14,483 PointsHi Malcom,
Let me try and help you out on this.
So if you look closely the relativeLayout has already been defined at the top with the name mRelativeLayout
private RelativeLayout mRelativeLayout;
Then at the bottom you're trying to define it agin using a different name called mealLayout
RelativeLayout mealLayout = (RelativeLayout) findViewById(R.id.mealLayout);
Therefore when you call this line
mRelativeLayout.setBackgroundColor(Color.GREEN);
the compiler gets confused because you've initialised mealLayout and no mRelativeLayout. Since mRelativeLayout has not been initialised but only declared it gives you a null pointer exception
Therefore as a solution
RelativeLayout mealLayout = (RelativeLayout) findViewById(R.id.mealLayout);
//instead of this this line type
mRelativeLayout = (RelativeLayout) findViewById(R.id.mealLayout);
// now when you call
mRelativeLayout.setBackgroundColor(Color.GREEN);
// it will refer to the correct relative layout
Hoep that helps. Feel free to post again if you need any help

Malcolm Mutambanengwe
4,205 PointsThanks Gunjeet. It's making sense, I finally got it. thanks. Learning programming is like learning a new language, literary.
Malcolm Mutambanengwe
4,205 PointsMalcolm Mutambanengwe
4,205 PointsHi Steve. finally understood what was needed. Thanks for your help. The coding force is strong in you, jedi Steve.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsA challenging language, Android is.
