Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial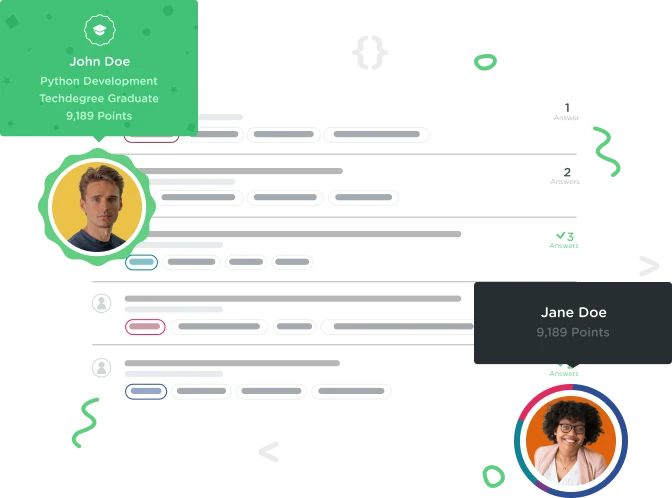
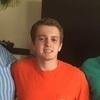
Tyler Lowrey
14,538 Pointsjava.lang.OutOfMemoryError: Java heap space error with Teamwork challenge
I am encountering an error that I have been unable to successfully fix while trying to complete the code challenge following the "Teamwork" video.
The error stack trace I receive is the following:
Exception in thread "main" java.lang.OutOfMemoryError: Java heap space
at java.util.Arrays.copyOf(Arrays.java:3236)
at java.io.ByteArrayOutputStream.grow(ByteArrayOutputStream.java:118)
at java.io.ByteArrayOutputStream.ensureCapacity(ByteArrayOutputStream.java:93)
at java.io.ByteArrayOutputStream.write(ByteArrayOutputStream.java:153)
at java.io.PrintStream.write(PrintStream.java:480)
at sun.nio.cs.StreamEncoder.writeBytes(StreamEncoder.java:221)
at sun.nio.cs.StreamEncoder.implFlushBuffer(StreamEncoder.java:291)
at sun.nio.cs.StreamEncoder.flushBuffer(StreamEncoder.java:104)
at java.io.OutputStreamWriter.flushBuffer(OutputStreamWriter.java:185)
at java.io.PrintStream.write(PrintStream.java:527)
at java.io.PrintStream.print(PrintStream.java:669)
at java.io.PrintStream.println(PrintStream.java:806)
at com.teamtreehouse.Prompter.promptForWord(Prompter.java:108)
at com.teamtreehouse.Prompter.promptForWords(Prompter.java:88)
at com.teamtreehouse.Prompter.run(Prompter.java:46)
at com.teamtreehouse.Main.main(Main.java:16)
at JavaTester.run(JavaTester.java:77)
at JavaTester.main(JavaTester.java:39)
I suspect that the issue is in the part of my code here:
(From Prompter.java)
public String promptForWord(String phrase) throws IOException
{
boolean censored = false;
String replacement;
do
{
System.out.println(String.format("Enter a replacement for %s: ", phrase);
replacement = mReader.readLine();
if (mCensoredWords.contains(replacement))
{
System.out.println("That is a censored word! Please enter an uncensored word.");
censored = true;
}
} while (censored);
return replacement;
}
Specifically where "replacement = mReader.readLine();". I suspect that what is happening is that too much data is being read in by the Java tests, however I am unsure and I am not sure how to adapt my code to run successfully for the code challenge. The code works fine on my local machine. The complete code for the challenge is below.
Main.java
package com.teamtreehouse;
public class Main
{
public static void main(String[] args)
{
Prompter prompter = new Prompter();
String story = prompter.promptForTemplateString();
Template tmpl = new Template(story);
prompter.run(tmpl);
}
}
Prompter.java
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter
{
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter()
{
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords()
{
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try
{
words = Files.readAllLines(file);
}
catch (IOException e)
{
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl)
{
List<String> results = null;
try
{
results = promptForWords(tmpl);
}
catch (IOException e)
{
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
String result = tmpl.render(results);
System.out.printf("Your TreeStory:%n%n%s", result);
}
public String promptForTemplateString()
{
System.out.println("Please enter the template (__word__):");
try
{
return mReader.readLine();
}
catch (IOException e)
{
e.printStackTrace();
}
return "";
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException
{
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders())
{
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) throws IOException
{
boolean censored = false;
String replacement;
do
{
System.out.println("Enter a replacement for " + phrase + ":");
replacement = mReader.readLine();
if (mCensoredWords.contains(replacement))
{
System.out.println("That is a censored word! Please enter an uncensored word.");
censored = true;
}
} while (censored);
return replacement;
}
}
Template.java (Unedited from what was provided)
package com.teamtreehouse;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Template
{
/**
* The string format that is waiting to receive values
*/
private String mCompiled;
private List<String> mPlaceholders;
/**
* @param text A template with double underscored surrounded placeholders. eg: Hello __name__!
*/
public Template(String text)
{
// Match on double underscore surrounded words, like __name__ or __proper noun__
Pattern pattern = Pattern.compile("__([^__]+)__");
Matcher matcher = pattern.matcher(text);
mPlaceholders = new ArrayList<String>();
while (matcher.find())
{
String label = matcher.group(1);
mPlaceholders.add(label);
}
mCompiled = matcher.replaceAll("%s");
}
/**
* @return Ordered names of placeholders in the template
*/
public List<String> getPlaceHolders()
{
return mPlaceholders;
}
/**
* Given a list of values, replaces the fill in the blanks in order.
*
* @param values The replacements for the fill in the blank
* @return The filled out TreeStory
*/
public String render(List<String> values)
{
// String.format accepts the templates and Object... (a variable amount of objects)
return String.format(mCompiled, values.toArray());
}
}
Any help with this issue would be appreciated.
1 Answer
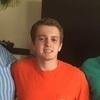
Tyler Lowrey
14,538 PointsThe issue was actually the line preceding (and another line) the
replacement = mReader.readLine();
The real issue was both of the the "println"s
System.out.println(String.format("Enter a replacement for %s: ", phrase);
and
System.out.println("That is a censored word! Please enter an uncensored word.");
I altered my code by moving the first print statement outside of the loop and altering the censor code to work without printing an error statement, as such:
public String promptForWord(String phrase) throws IOException
{
boolean censored = false;
String replacement = "";
System.out.println("Enter a replacement for " + phrase + ":");
do
{
replacement = mReader.readLine();
if (!mCensoredWords.contains(replacement))
{
censored = false;
}
else
{
censored = true;
}
} while (censored);
return replacement;
}
I think that the memory allocated to the VM that runs the tests for this challenge is insufficient (But I'm unfamiliar with exactly how the challenges work, so I could be wrong; I could've just been providing an inefficient solution).