Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial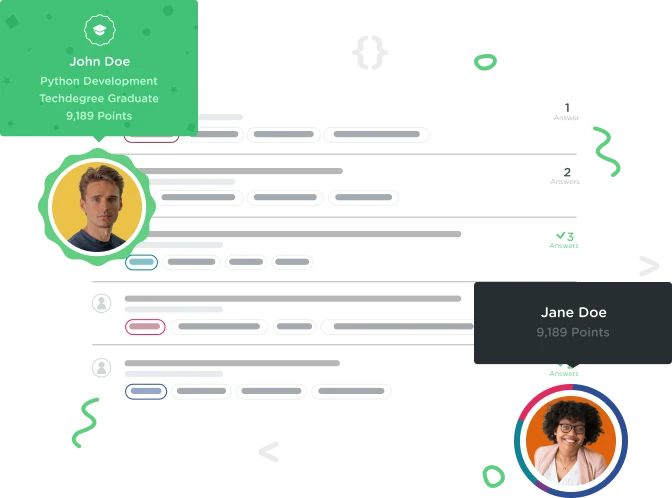

Cameron Maciel
1,433 PointsJavascript
ok so i have created these variables, now what i want to create is if the input of small + medium + large + x-large + 2x-large+ 3x-large + 4x-large is <= 30 then these prices apply
how create this "if Statement"
this will be different for every qty, so the next ones would be <=49, <= 99, <= 199, <=499, <=999
Each of these will have a different price for each color
here is my code
var shirtsPrice = 3;
var 1230perOneColorPrice = 5.50;
var 1230perTwoColorPrice = 8.18;
var 3649perOneColorPrice = 4.48;
var 3649perTwoColorPrice = 5.33;
var 3649perThreeColorPrice = 6.18;
var 5099perOneColorPrice = 3.05;
var 5099perTwoColorPrice = 3.43;
var 5099perThreeColorPrice = 3.90;
var 5099perFourColorPrice = 4.23;
var 100199perOneColorPrice = 2.15;
var 100199perTwoColorPrice = 2.43;
var 100199perThreeColorPrice = 2.78;
var 100199perFourColorPrice = 3.13;
var 200499perOneColorPrice = 1.68;
var 200499perTwoColorPrice = 1.93;
var 200499perThreeColorPrice = 2.18;
var 200499perFourColorPrice = 2.43;
var 500999perOneColorPrice = 1.33;
var 500999perTwoColorPrice = 1.53;
var 500999perThreeColorPrice = 1.73;
var 500999perFourColorPrice = 1.93;
var inputNum_of_Small = $('#Small').val();
var inputNum_of_Medium = $('#Medium').val();
var inputNum_of_Large = $('#Large').val();
var inputNum_of_X-Large = $('#X-Large').val();
var inputNum_of_2X-Large = $('#2X-Large').val();
var inputNum_of_3X-Large = $('#3X-Large').val();
var inputNum_of_4X-Large = $('#4X-Large').val();
var inputNum_of_FColor = $('#colorsOnFront').val();
var inputNum_of_BColor = $('#colorsOnBack').val();
2 Answers
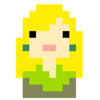
Alexandria Wagner
7,038 PointsHi Cameron,
An if statement in JavaScript works very similarly to writing a normal sentence. For example, "If I am ordering a total of 30 shirts or less, the cost of a shirt would be $5. Otherwise, the cost would be $4," would look like:
if(totalShirts <= 30){
var shirtCost = 5;
} else {
var shirtCost = 4;
}
Notice that else is used for my "otherwise" situation. You can read more information about if...else statements on the Mozilla Developer Network.
Now, that if statement in itself doesn't help you very much. You need to communicate what you would like done with the information. Notice that I used "totalShirts" as an argument for the if statement above. You can define the totalShirts variable and write an instruction to log the shirt cost to the console for you to use. This would look like:
var totalShirts = ;
console.log(shirtCost);
In this case, if I wrote var totalShirts = 12 and called the console.log function on shirtCost, it would return 4, as specified above.
Please note that you cannot start a JavaScript variable name with a numeral. Variable names must start with a letter, underscore (_), or dollar sign ($). More information about variable declaration can be found here, again on the MDN.
I hope this information helps you! -Alex

Petar Popovic
Courses Plus Student 23,421 PointsI don't know is this some kind of an advanced technique and I did't came to that stage yet, but as I know you can't start a variable with number
Cameron Maciel
1,433 PointsCameron Maciel
1,433 PointsAwesome thanks that help out some. But may I ask if I order 30 shirts that need a two color print on the front. The price is $3 plus $8.18 for the two color print
But the printing Price will decrease as the number of shirts order goes up
Alexandria Wagner
7,038 PointsAlexandria Wagner
7,038 PointsIt's possible to write a crazy amount of if statements to specify exactly what you want for a huge number of potential scenarios (for example, ordering less than 30 small shirts with one color, ordering less than 30 small shirts with two colors, ordering less than 30 medium shirts with one color, etc.), BUT this is not the most efficient way to solve your problem.
For the sake of argument, I'll roll with it, but I think you'll find easier and more efficient solutions once you get to the modules on functions and loops.
Let's use an example where we define how much a plain shirt costs ($3), an arbitrary total number of shirts ordered (12, in this case), and another arbitrary total number of colors used (2, in this case) to specify the additional print cost. In my example, I will be using a whole number ($8) for the additional print cost, because JavaScript uses 64-bit floating point representation. We can nest if statements for specificity.
JavaScript has some really neat built-in features, like logical operators which can be used to specify more than one thing in a given argument. The code above can be written as follows, using the logical AND operator:
I'm sure you can see how you would end up writing mass amounts of if statements at this rate.
Hopefully, that makes a little more sense. -Alex
Cameron Maciel
1,433 PointsCameron Maciel
1,433 Pointswow thank you so much Alex you were such a big help with this project. Thank you soooooo much. And yes i do see how this can and will be a pain doing it this way.