Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial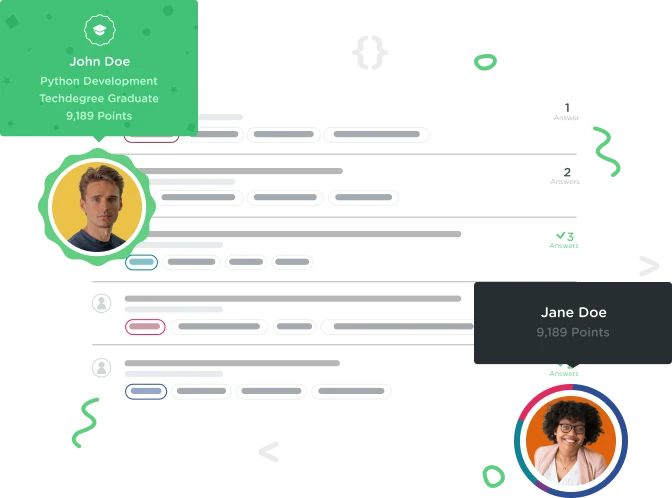

Sean Poore
5,365 PointsJavaScript Add Class on Click Help
Hello! I'm attempting to make a responsive navbar via a menu button and when said button is clicked a containing div appears with the navigation links. In order to do so when this element is clicked, I want the element to have the class 'toggled' added to it.
Here is my html:
<div class="menu-container">
<i class="fas fa-bars"></i>
</div>
Here's the JavaScript:
$('fas.fa-bars').on('click', function() {
$(this).addClass("toggled");
});
Could someone please help me out and let me know how to do this?!
5 Answers

Tony Idehen
8,060 PointsHi Sean, here is my made up lines of codes to display when clicked and closes when X is clicked. Case in point, one $(document).ready(function(){ } maybe enough for all your click events. Let me know if this is of any help, I am learning just like you. Good luck with your project.
<!--My made up style-->
<style>
.toggled {
position: relative;
width: 200px;
height: 200px;
background-color: beige;
border: 2px solid black;
}
h1 {
text-align: right;
font-size: 26px;
cursor: pointer;
right: 10px;
}
.close-menu {
position: absolute;
width: 25px;
height: 25px;
top: 0;
right: 10px;
}
</style>
</head>
<body>
<!--main-container with 2 div inside-->
<div class="main-container">
<div class="fbars">
<i class="fa fa-bars" style="font-size:36px; color:red"></i>
</div>
<div class="menu">
<h1 class="close-menu">X</h1>
</div>
</div>
<script>
$("h1").hide(); // hide the X
$(document).ready(function(){ // you can use this line for more event, no piont repeating it in this file
// add toggle and display X
$(".fbars").click(function() {
$("div.menu").addClass("toggled");
$("h1").show(); // Display the X
}); // end of display/toggle
// close hide X and remove class toggle
$(".close-menu").click(function() {
$("div.menu").removeClass("toggled");
$(this).hide(); // hide the X
}); //End of remove/hide
// Put next code/click event under the same $(document).ready().
}); //End of ready()
</script>

Tony Idehen
8,060 Points1) Get the class name you will be adding to 2) Since only 1 class, use 0 as index 3) Add it to the classList. Then check to see if it is there(DOM) with DevTools
<div class="menu-container">
<i class="fas fa-bars">Here</i>
</div>
<script>
var menuContainer = document.getElementsByClassName("menu-container");
menuContainer[0].addEventListener('click', function() {
menuContainer[0].classList.add("toggled");
});
</script>

Tony Idehen
8,060 PointsThis worked for me. You can interchange the div and i, depends on where you want to place your new class.
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("i").click(function() {
$("i").addClass("toggled");
});
});
</script>
</head>
<body>
<div class="menu-container">
<i class="fas fa-bars" style="font-size:36px"></i>
</div>
</body>
</html>

Tony Idehen
8,060 PointsThis worked too.
<script>
$(document).ready(function(){
$(this).click(function() {
$("i").addClass("toggled");
});
});
</script>
<div class="menu-container">
<i class="fas fa-bars" style="font-size:36px"></i>
</div>

Sean Poore
5,365 PointsHello! I really appreciate the help, that also worked for me to make the menu pop up, now I'm working on making the menu disappear when clicking the X but I'm also having trouble with this.
Here's my html:
<div class="menu-container">
<i class="fas fa-bars"></i>
<div class="menu">
<h1 class="close-menu">X</h1>
</div>
</div>
And here's my JavaScript:
$(document).ready(function(){
$(".menu-container").click(function() {
$(".menu").addClass("toggled");
});
});
$(document).ready(function(){
$(".close-menu").click(function() {
$(".menu").removeClass("toggled");
});
});
The portion of the JS that makes the menu appears works however the portion that makes the menu disappear again doesn't.

Tony Idehen
8,060 PointsTry this and group your div the way you want, because you are adding a class/div you have to target the element
You can also target any specific class in an element as follows:
1) If in div you can use $("div.menu")
2) If in h3 you can use $("h3.menu")
3) If in p (paragraph) you can use $("p.menu")
etc etc......
<div class="fbars">
<i class="fa fa-bars" style="font-size:36px; color:red"></i>
</div>
<div class="menu">
<h1 class="close-menu">X</h1>
</div>
<script>
$(document).ready(function(){
$(".fbars").click(function() {
$("div.menu").addClass("toggled");
});
$(".close-menu").click(function() {
$("div.menu").removeClass("toggled");
});
});
</script>

Tony Idehen
8,060 Points <div class="main-container">
<div class="fbars">
<i class="fa fa-bars" style="font-size:36px; color:red"></i>
</div>
<div class="menu">
<h1 class="close-menu">X</h1>
</div>
</div>
<script>
$(document).ready(function(){
$(".fbars").click(function() {
$("div.menu").addClass("toggled");
});
$(".close-menu").click(function() {
$("div.menu").removeClass("toggled");
});
});
</script>

Sean Poore
5,365 PointsThank you Tony for all the help, i've now got this menu working! And yes, Instead of the hard coded <h1>X</h1> I have indeed changed my code to another font-awesome close button which looks much better.
Anyway, I appreciate all the help, thank you!

Tony Idehen
8,060 PointsHi Sean, you are welcome. I am glad it was useful to you.
Tony Idehen
8,060 PointsTony Idehen
8,060 PointsSean, I prefer the hard coding you are doing, but have you check font awesome or Bootstrap for close button to customize?