Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial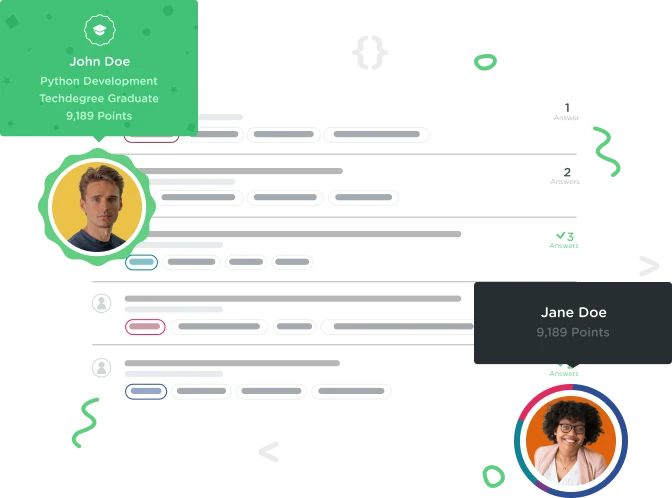

Joseph M.
2,057 PointsJavaScript and the DOM
The task requires that I assign the listItems to a collection, where the collection contains items on a list with the id 'rainbow. when I try to check the code I get: Bummer: works now.
var listItems = document.querySelector('#rainbow').children;
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i <colors.length; i++) {
listItems[i].style.color = colors[i];
}
<!DOCTYPE html>
<html>
<head>
<title>Rainbow!</title>
</head>
<body>
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
3 Answers
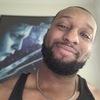
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsHi Joseph M,
So there are two issues for you to look at.
One. You've changed some of the code that was given to you. The for loop should read colors.length, not listItems.length.
Two. document.getElementById() does not return a collection. It returns a single item. I would suggest using the querySelectorAll method. document.querySelectorAll()
. It uses CSS queries to determine which elements to return, and it does return a collection.
You can read about this particular method at https://developer.mozilla.org/en-US/. Just search for querySelectorAll
Hope this helps. Feel free to reach back out if I haven't explained this well enough.

Joseph M.
2,057 PointsThanks Brandon White . I did change back to colors.length and also used document.SelectorAll('#rainbow'). Getting the error: Bummer: There was an error with your code: TypeError: 'undefined' is not an object (evaluating 'listItems[i].style')
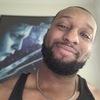
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsHi Joseph,
SelectorAll is not a method. The method I'm suggesting you use is querySelectorAll. It's very important in any programming language to spell things correctly (casing matters).
Also, you're not looking to return the ul element, you're wanting the list elements. The selector that would get you that would be #rainbow li. There are many ways to do this, I'm sure, but using the querySelectorAll method was simply the first one that popped into my mind.
That said, you may want to research CSS selectors. They are very important with respect to traversing and manipulating the DOM.

Joseph M.
2,057 Pointssorry for that, in the workspaces I typed it correctly as document.querySelectorAll('#rainbow'), but still get the above error.

Joseph M.
2,057 Pointsfound the answer! the task requires that the coloring is iterated through the <li> of the html which is the children of the ID rainbow. So I did var listItems = document.querySelector('#rainbow').children; at the top (line 1) and it worked. any other way to look at it?
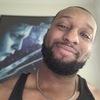
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsNice job, Joseph.
Thatβs a great choice that you made. As far as other ways to look at it, Iβll point you to the one I proposed in my earlier message, which was: var listItems = document.querySelectorAll(β#rainbow liβ)
, as this would also return the correct collection of items.
Also, in this case the only li elements in the html doc are the ones you need, so you should be able to use document.querySelectorAll(βliβ), or document.getElementsByTagName(βliβ).