Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial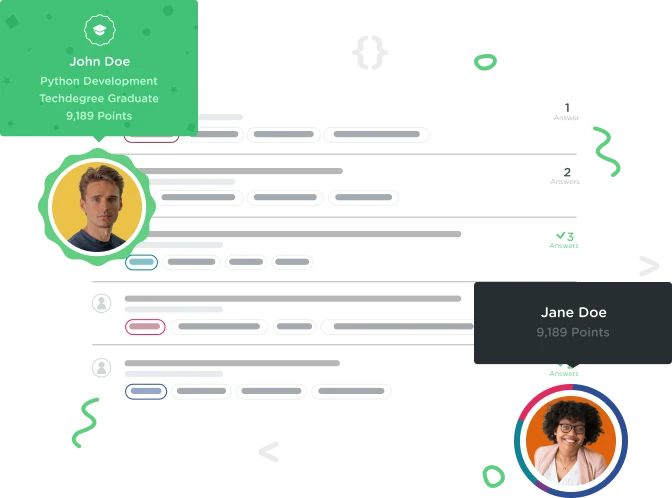

Justyna Leżuch
10,534 PointsJavaScript and the DOM Challenge Task
Hi, do you know how solve this problem?
In the listener that has been added to the section element, ensure that the text input elements are the only children that trigger the background-changing behavior.
{SOLVED}
let section = document.getElementsByTagName('section')[0];
section.addEventListener('click', (e) => {
e.target.style.backgroundColor = 'rgb(255, 255, 0)';
});
8 Answers
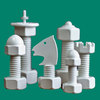
Steven Parker
231,236 Points
You could just add a check and avoid the change for any undesired types:
section.addEventListener('click', (e) => {
if (e.target.tagName != "INPUT") return;
e.target.style.backgroundColor = 'rgb(255, 255, 0)';
});
The nested ternary solution suggested by simhub seems a bit cryptic, and operates on the first child of the target instead of the actual target. Plus the handler doesn't need to return a value.
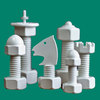
Steven Parker
231,236 PointsYour second solution should also work, you just forgot to capitalize the tag name "INPUT".
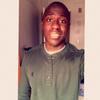
Allan Oloo
8,054 PointsHey can you explain this part let section = document.getElementsByTagName('section')[0];
I dont understand why include [0] next to that dom property?

josefina terrera
16,506 Pointswhy did you add "return;"?
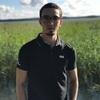
Yazan Alhalabi
10,483 Pointssection.addEventListener('click', (e) => { if (e.target.tagName == 'INPUT') { e.target.style.backgroundColor = 'rgb(255, 255, 0)'; } });

johnny louifils
Full Stack JavaScript Techdegree Graduate 18,926 Pointsmy question is why do we need to put the "!" before the equal sign in the if conditional statement
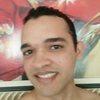
Rafael silva
23,877 PointsThis is the best way to organize this code because ,if you want to call the function, you need to indetify and use if statement and compare, if is equal the is true and this will work, I hope help .
section.addEventListener('click', (e) => { if (e.target.tagName == "INPUT") { e.target.style.backgroundColor = 'rgb(255, 255, 0)'; } });

Nickolas Fuentes
14,016 PointsI had all of this code down but dont understand why it was a 'INPUT' and not 'input' ? there wasnt a .toUpperCase added anywhere?

Sean May
9,145 PointsNickolas Fuentes it has to be 'INPUT' because the event.target.tagName
returns the names of the tags as uppercase
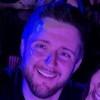
Sam Allen
7,176 PointsSuch a tedious and tricky exercise! This worked for me (commented out the default code that came with the challenge for before/after comparison's sake):
let section = document.getElementsByTagName('section')[0];
// section.addEventListener('click', (e) => {
// e.target.style.backgroundColor = 'rgb(255, 255, 0)';
// });
section.addEventListener('click', (e) => {
if (event.target.tagName == "INPUT") {
event.target.style.backgroundColor = 'rgb(255, 255, 0)';
}
});
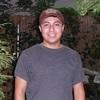
Brian Ramon
6,322 PointsThanks Sam Allen. I don't know why my code wouldn't work, I had tried it numerous times, even typed it in exactly as yours, but with no success. Then I simply copy pasted your code into the editor and it worked. I compared it many many times before and it wouldn't work. Scratching my head!
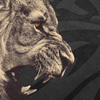
Hosam Darwish
14,309 Pointslet section = document.getElementsByTagName('section')[0];
section.addEventListener('click', (e) => { if (e.target.tagName != "INPUT") return; e.target.style.backgroundColor = 'rgb(255, 255, 0)'; });
With this you will pass the test.
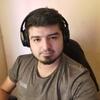
Rodrigo Valladares
9,708 Pointsbut that is correct but why ?? " != "

Clare Rayner
2,583 PointsThis question really beat me... i was almost there! just like so many other DOM questions.. i dont understand why it didnt work for me when i changed all the 'e's to 'event'....and why did we need to use return? was this really so different from the example that was shown in the previous video? because i tried to apply the same formula and it didnt work:
(not answer to forum solution) listDiv.addEventListener('mouseover',(event) => { if(event.target.tagName =='LI') { event.target.textContent=event.target.textContent.tpUpperCase(); } });
can someone explain why we needed two sets of {} here and not in the other solution?

Warren Fung
4,268 Pointsi think it didn't work since you put 'mouseover', while the question uses 'click'. your first set of {} is for the if statement and the second one is for the arrow function. the naming of the event variable doesn't matter.
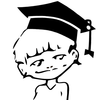
simhub
26,544 Pointshi justyna,
you could try something like this:
section.addEventListener('click', (e) => {
return (e.target.children.length != 0) ? (e.target.children[0].tagName === "INPUT") ? e.target.children[0].style.backgroundColor = 'rgb(255, 255, 0)' : void 0 : void 0;
});

Justyna Leżuch
10,534 PointsUnfortunately it does not work :(
I try to do something like :
let section = document.getElementsByTagName('section')[0];
section.addEventListener('click', (e) => {
if(e.target.tagName == "input") {
e.target.style.backgroundColor = 'rgb(255, 255, 0)'; }
});

Justyna Leżuch
10,534 PointsThank you guys !!

roman rivkin
1,689 PointsHi guys, still didnt get why it's ------> ('section')[0]; index 0 ??? (why index zero) thx.
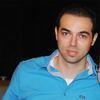
Ezra Siton
12,644 Pointsroman rivkin. The index starts from zero
The getElementsByTagName() method returns a collection of all elements in the document with the specified tag name, as a NodeList object.
The NodeList object represents a collection of nodes. The nodes can be accessed by index numbers. The index starts at 0 (w3c)
W3C https://www.w3schools.com/Jsref/met_document_getelementsbytagname.asp
Tommy Gebru
30,164 PointsTommy Gebru
30,164 PointsWere you able to solve this problem?