Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial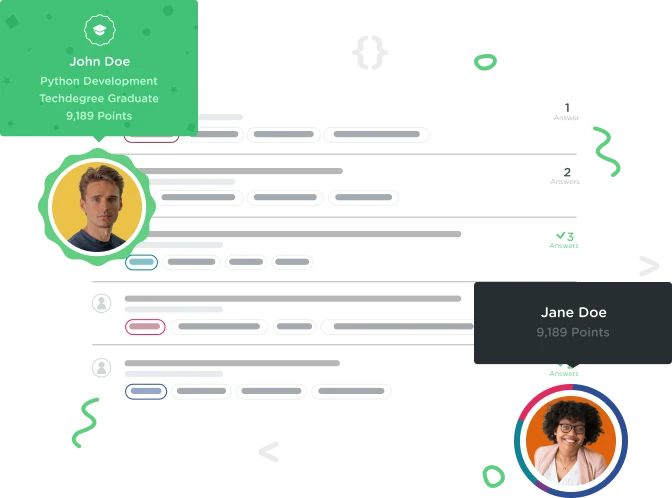
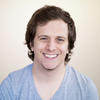
Patrick Bell
Treehouse TeacherJavaScript and the DOM | Selecting by Id | let vs. const
Why in the code challenge for Selecting by Id in JavaScript and the DOM are we using let instead of const? In the video before this code challenge we used const. So when the challenge asked me to use let instead, it really confused me. What's the difference between the two? Why the change?
// In previous video we wrote the code 'const button = document.getElementById('sayPhrase');'
let button = document.getElementById('sayPhrase');
let input;
button.addEventListener('click', () => {
alert(input.value);
});
<!DOCTYPE html>
<html>
<head>
<title>Phrase Sayer</title>
</head>
<body>
<p><input type="text" id="phraseText"></p>
<p><button id="sayPhrase">Say Phrase</button></p>
<script src="js/app.js"></script>
</body>
</html>
2 Answers
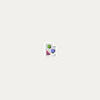
doesitmatter
12,885 PointsThis is because a constant variable without an initial value is not allowed, so the code of the challenge would be incorrect if it was:
const buttonA;
const buttonB;
This is why they use let instead, so that they can write:
let buttonA;
let buttonB;
without it giving an error, because the let variable does not have to be initialized upon creation.
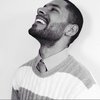
Michael Liendo
15,326 PointsVariables with the const keyword can't be modified, but down below we're modifying the button by adding the event listener. So in the previous challenge, if you were just grabbing the button, then it makes sense to make it a const, but once you start manipulating it, it should be let.
As an aside, and not to confuse you: When it comes to objects, a lot of what I just said is considered "best-practice".
Consider the following code:
const a = 3
a + 1; //not allowed
const b = {
name: "Michael"
}
b.name = "Mike" // allowed
but
const c = {
name:"Mitch"
}
//not allowed, since I'm not simply accessing the existing value and updating it, but rather creating a new object literal.
c = {
name: "Mick"
}
The difference is that, when it comes to objects, since they're pass by reference, their values can be updated, but the object itself can't be replaced in memory by anything else.
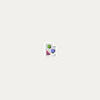
doesitmatter
12,885 PointsVariables with the const keyword can't be modified, but down below we're modifying the button by adding the event listener.
not really true, adding an event listener does not modify the value stored in const, watch the video before the code challenge, then you will see Guil use const while still adding an event listener
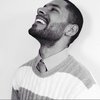
Michael Liendo
15,326 PointsI was noting it as a best practice when using it with objects (dot-syntax). Though, perhaps my examples were unclear. I appreciate the feedback
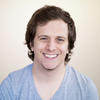
Patrick Bell
Treehouse TeacherThank you for all the feedback First and Michael, I think I get it now! I also think it might help if I take the prerequisites for this course! :)
Michael Liendo
15,326 PointsMichael Liendo
15,326 Pointsgood point. Missed that one.