Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial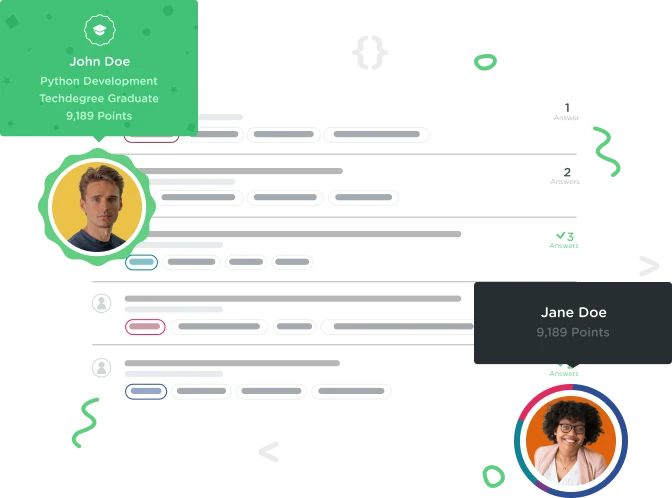

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsJavaScript and the DOM, Unit Testing
JavaScript and the DOM, Unit Testing
Task : 1.First, Write a function called Greet(name) that takes an argument called name, and returns a simple greeting message. For example, if the name is “Elizabeth” the function should return “Hello, Elizabeth”.
2.Next, handle null values by introducing a default. For example, when the name parameter is null, then the method should return the string “Hello there!”.
3.Next, add shouting. When “name” is all uppercase, then the method should shout back to the user. For example, when the name is "JOSE" then the method should return the string "HELLO JOSE!".
4..Next, Handle two names as input. If the name parameter is an array containing two names, then both names should be greeted. For example, if the input parameter is [‘Jose’,’Pep’], the method should return the string: “Hello, Jose, Pep”.
5.Finally, Handle an arbitrary number of names as input. If the name parameter is an array of multiple names, all names must be greeted. For example, if the input parameter is [‘Alex’,’Arsene’,’Jose’,’Zidane’], the method should return the string: “Hello, Alex, Arsene, Jose, Zidane”
JS CODE:
// Unit tests to cover the greet() function // To run these tests, make sure you're in the folder containing this file // run the command: npm install to setup the testing framework // Thn run the command: npm run test // The tests should run successfully
import greet from './greetings'; describe('test greet()', function () { it('should greet a name', function () { expect(greet('Elizabeth')).toEqual('Hello, Elizabeth'); }); it('should handle null value', function () { expect(greet()).toEqual('Hello there!'); }); it('should handle shouting', function () { expect(greet('JOSE')).toEqual('HELLO JOSE!'); }); it('should handle 2 names', function () { expect(greet(['Jose', 'Pep'])).toEqual('Hello, Jose, Pep'); }); it('should handle multiple names', function () { expect(greet(['Alex', 'Arsene', 'Jose', 'Zidane'])).toEqual( 'Hello, Alex, Arsene, Jose, Zidane' ); }); }); My answer”Any Hints, comments and suggestions are very much welcome, I have a couple of questions I tried to answer please correct me if I am wrong, thank you in advance for your help.” export default function greet(name) { if (name === null || name === undefined) return 'Hello there!'; if (typeof name === typeof []) { if (name.length > 0) { let names = ''; name.forEach((elm) => { names += ', ' + elm; }); return 'Hello' + names; } return 'Hello there!'; } if (name === name.toUpperCase()) { return 'HELLO ' + name + '!'; } return 'Hello, ' + name; }
SNAP SHOT: https://w.trhou.se/7ywk0t0lnp
Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsKarl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsThis is what i have done so far, any comments, hints and or suggestions are very welcome and appreciated, please feel free to correct me if i am wrong, thank you in advance for all your help.