Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial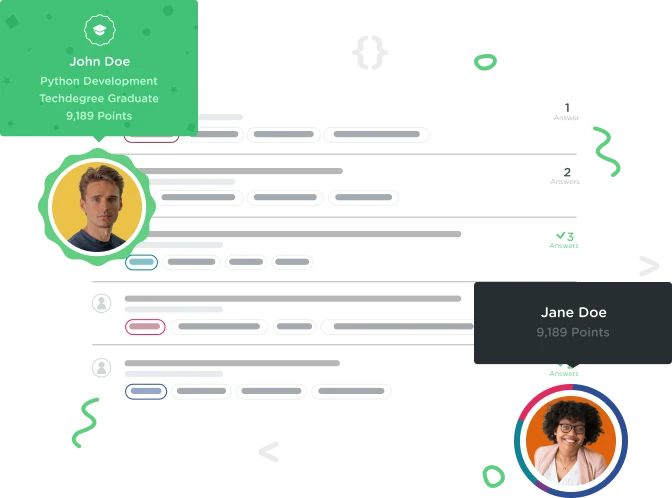

Samuel Fortunato
20,229 PointsJavaScript Array Iteration Methods Last Exercise = Stumped
The last exercise in this course has got me.
const customers = [
{
name: "Tyrone",
personal: {
age: 33,
hobbies: ["Bicycling", "Camping"]
}
},
{
name: "Elizabeth",
personal: {
age: 25,
hobbies: ["Guitar", "Reading", "Gardening"]
}
},
{
name: "Penny",
personal: {
age: 36,
hobbies: ["Comics", "Chess", "Legos"]
}
}
];
let hobbies;
// hobbies should be: ["Bicycling", "Camping", "Guitar", "Reading", "Gardening", "Comics", "Chess", "Legos"]
// Write your code below
This is what I have so far:
hobbies = customers
.reduce((hobbiesList, customer) => customer.personal.hobbies.map(hobby => hobby));
Not working. Don't get it. :(
1 Answer
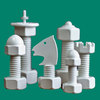
Steven Parker
231,275 PointsYou seem to be heading in the right direction. Here's a few hints:
- that "map" function isn't doing anything, you can remove it entirely
- you might need to give "reduce" an additional argument for an initial value
- the function needs to use both arguments
- the function should do something that combines two arrays together into one
Samuel Fortunato
20,229 PointsSamuel Fortunato
20,229 PointsOk, I've gotten farther. My code is now this:
And my output is now this, according to the code challenge:
"Bicycling,CampingGuitar,Reading,GardeningComics,Chess,Legos"
Seems like I just have to get each array item individually and not grab the whole thing? Why isn't reduce() grabbing each array item individually? I thought that's what it did?
Steven Parker
231,275 PointsSteven Parker
231,275 PointsYou're really close now! You're successfully combining all the individual hobbies into a string, so now you only need to change it so that the combination is another array. Hint: the "spread" operator ("...") might be useful.