Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial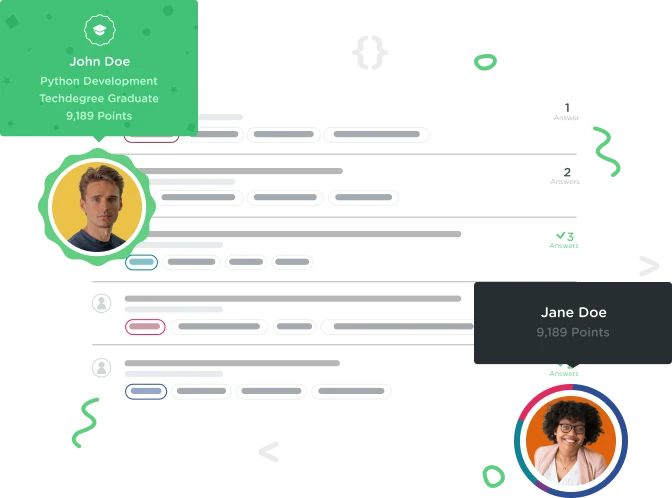
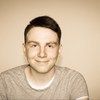
Sarunas Medeikis
1,141 PointsJavaScript assigning value by a reference, thus changing the passed value itself ( Eloquent Javascript) , need help..
Hello, i am in a pinch, I don't get a bit of code and how it was done. I tried reading about it, and I advanced to the point where I understand that It is passed as a reference, but apart that, I still don't know how this code works, it is from Eloquent javascript 4.2 exercise. I managed to do the first part of the exercise on my own quite easily. It is different than tutorial answer showed . My version is : This is how I did first part.
```function reverseArray(array){ var reversedArray =[]; for(var i = 0; i<array.length; i++){ reversedArray.unshift(array[i]); } return reversedArray; }
Now.. The second part, asks me to reverseArrayInPlace .. Which I find confusing, as i can't use the same method as I used before. As I wasn't capable of changing the value from parameters, I looked at solution and got puzzled. The solution for second part is :
```function reverseArrayInPlace(array) {
for (var i = 0; i < Math.floor(array.length / 2); i++) {
var old = array[i];
array[i] = array[array.length - 1 - i];
array[array.length - 1 - i] = old;
}
return array;
}
What i don't understand is : why we divide array.length /2 ? and why we do array[i] = array[array.length -1 -i]; why we need to substract 1 and i ? I am very confused with this part of code, can someone help me out ? I am very confused now.
Why do we need return array; ? If we are changing the array itself, why do we need to return it ?
I would be really pleased if someone helped me to understand it ! As I think it's important to get that part.
ADDED CODE TO JSFIDDLE: https://jsfiddle.net/31voprq1/ This is the place where i test code : http://eloquentjavascript.net/code/#4.2
1 Answer

Cristian Altin
12,165 PointsBasically reverseArrayInPlace swaps values between: 1) first and last 2) second and second to last 3) so on until it reaches half the array
In the example of [1,2,3,4,5] these are the steps: [5,2,3,4,1] (first and last swapped) [5,4,3,2,1] (second and second to last swapped)
If the array has an odd number of elements (like the example above) the middle element will stay there else it will swap the two in the middle and end. Since an odd number will lead to a non int value Math.floor is used on that half value to make the iteration stop at the right index both for odd and even element length of the array.
You understand now why it stops at halfway. If it were to go all the way to the end it would restore the initial array making the whole function useless (it would reverse the reversed array).
Almost forgot your other question. Since arrays start at index 0 while the length tells the number of elements you need to subtract 1 to reach the last element. Consider an array with 1 element. Its index is 0 while the array.length gives 1. To get to that element you need length-1 which is 1-1 = 0 and it's the correct index indeed. Now for the -i part it's simple, it's what makes you reach the second to last, third to last and so on.
Lastly the return of the array is indeed redundant in my opinion since it is already been modified.
Sarunas Medeikis
1,141 PointsSarunas Medeikis
1,141 PointsI think I understand it now, I knew that to reach last item in array we need to do array.length - 1, just got lost with the -i so i thought that maybe i misunderstood something. So If I understand it correctly now, first we assigned var old so we can store the changed array to the array that was sent in.Then we used array[i] = array[ array.length -1 -i]; to reach the last element and set it's value to array[i] ? Then we used array[array.length -1 -i] = old. to save the value to the variable old, which will store it in the array ? ( var old = array[i] ) ? did i understood it correctly ?
Cristian Altin
12,165 PointsCristian Altin
12,165 PointsThe old variable is used just to temporarily store a value while swapping values. If there was no old variable you would do something bad like this on the first iteration: [5,2,3,4,5]
This is because it would copy the last value into first position OVERWRITING the value 1 with value 5 so that when it looks at the value to copy into last position now there's a 5 and you got a bug in your code having lost value 1. The value in the current position has to be first stored to old so that once it gets overwritten it can still be put into the final destination.