Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial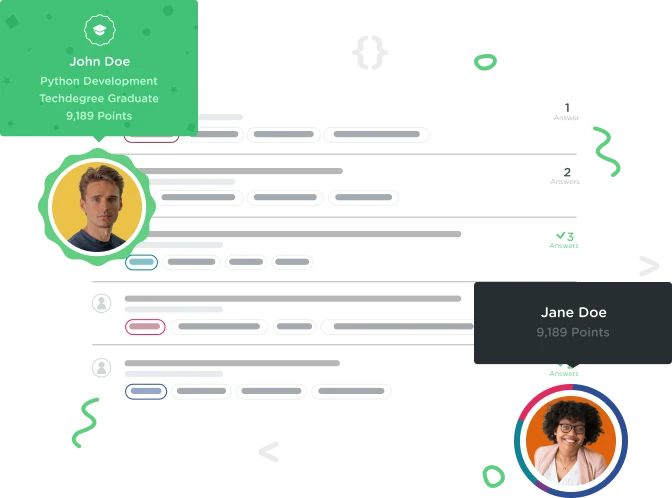
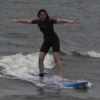
julieseif
11,063 PointsJavascript Basics Improving The Random Number Guessing Game Help Needed
I'm trying to figure out why, when I run my code, I am not getting the chance to input a second guess when I guess incorrectly.
I thank you for your help in advance
Here is the original video for reference: https://teamtreehouse.com/library/javascript-basics/making-decisions-with-conditional-statements/improving-the-random-number-guessing-game
Here is my code:
var correctGuess = false;
var randomNumber = Math.floor(Math.random() * 6 ) + 1;
var guess = prompt('I am thinking of a number between 1 and 6. What is it?');
if (parseInt(guess) === randomNumber ) {
correctGuess = true;
} else if (parseInt(guess) > randomNumber ) {
// is the guess lower than the random number?
var guessMore = prompt('Try again. The number I am thinking of is more than' + guess);
// we let the player know it is incorrect
if (parseInt(guessMore) === randomNumber {
correctGuess = true;
// this tests if the second guess is correct, and sets the value to true, because the user has gotten it right.
}
else if (parseInt(guess) < randomNumber ) {
//is the guess higher than the random number?
var guessMore = prompt('Try again. The number I am thinking of is less than' + guess);
// we let the player know it is incorrect
if (parseInt(guessMore) === randomNumber {
correctGuess = true;
// this tests if the second guess is correct, and sets the value to true, because the user has gotten it right.
}
if ( correctGuess ) {
// we test the value of this variable and print the appropriate message
document.write('<p>You guessed the number!</p>');
} else {
document.write('<p>Sorry. The number was ' + randomNumber + '.</p>');
}
2 Answers

Eric Garnick
6,991 PointsFor one thing, you're missing closing parentheses in lines 11 and 22:
11 if (parseInt(guessMore) === randomNumber {
22 if (parseInt(guessMore) === randomNumber {
And then you are missing closing braces for those same if statements. Adding these closing parentheses and braces makes the code run, but you also have your greater than and less than tests backwards.
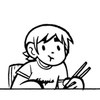
Hugo Buenrostro
10,620 PointsTry with this correction...
var correctGuess = false; var randomNumber = Math.floor(Math.random() * 6 ) + 1; var guess = prompt("I am thinking of a number between 1 and 6. What is it?");
if(parseInt(guess) === randomNumber ) { correctGuess = true; } else if(parseInt(guess) > randomNumber) { var guessMore = prompt("Try again. The number I am thinking of is more than" + guess);
if(parseInt(guessMore) === randomNumber) {
correctGuess = true;
} else if (parseInt(guess) < randomNumber) {
var guessMore = prompt("Try again. The number I am thinking of is less than");
}
if (parseInt(guessMore) === randomNumber) {
correctGuess = true;
}
}
if ( correctGuess ) { document.write("<p>You guessed the number!</p>"); } else { document.write("<p>Sorry. The number was " + randomNumber + ".</p>"); }
Enjoy. :)