Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial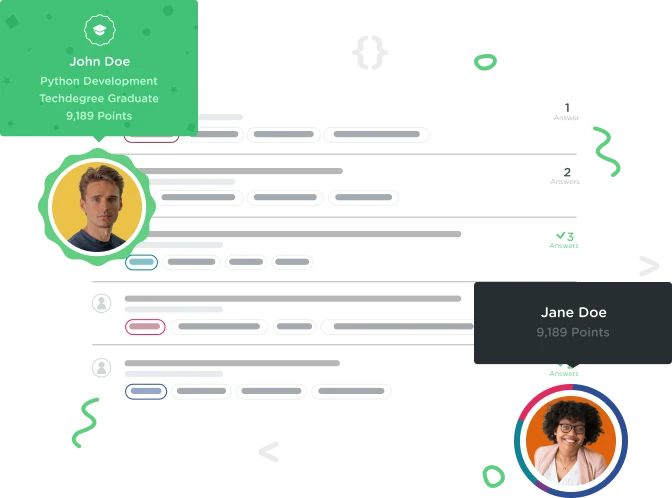

Steve Muthig
4,225 PointsJavaScript Code Challenge ???
Modify the Car's Prototype to have "wheels" set to 4
<head> <title> JavaScript Foundations: Objects</title> <style> html { background: #FAFAFA; font-family: sans-serif; } </style> </head> <body> <h1>JavaScript Foundations</h1> <h2>Objects: Prototypes</h2> <script>
var carPrototype = {
model: "generic",
currentGear: 0,
increaseGear: function() {
this.currentGear ++;
},
decreaseGear: function() {
this.currentGear--;
}
}
function Car(model) {
this.model = model;
}
Car.prototype = carPrototype; {
wheels = new Car ("4");
}
</script>
</body> </html> '''
6 Answers

Russell Wood
12,088 PointsI believe that you just need to add a wheels property to the carPrototype and set a default value of 4 like so:
''' ... decreaseGear: function() { this.currentGear--; }, wheels: 4 }'''

Russell Wood
12,088 PointsIt doesn't matter. You can declare a property after a method (in this case "decreaseGear") because in the end, they are both properties. Just be sure to put a "," after the closing "}" of the function to continue with the property declaration. However, what you have works just fine. Sometimes order is a matter of preference. Of course, I'm on this site because I'm not an expert either, just speaking from experience.

Mike Healy
2,451 PointsAh, I misread your code. It looked like wheels: 4 was inside the decreaseGear() method at first glance. That will work, but probably a lot less clear than grouping with the other properties at the top of the prototype.

Mike Healy
2,451 PointsHow about this? Passing 4 to the constructor will set that as the Model property.
var carPrototype = {
model: "generic",
wheels: 4,
currentGear: 0,
....

Russell Wood
12,088 PointsYep, I'm not quite clear on how I can make it look better because I've tried the markdown syntax and it generally looks as confusing either way.

Mike Healy
2,451 PointsIf you wanted to get the syntax highlighting use backticks (beside the 1) not single quotes You can specify a language after the opening backticks
var muchSyntax = true;
$highlight = 'wow';

Russell Wood
12,088 PointsAwesome! I've been using the wrong ones this entire time. Look at me I'm on top of the :
var hello = "Hello World!!!"

Mike Healy
2,451 PointsBtw, they have to start after a blank line

Russell Wood
12,088 PointsSo close, but thanks for the tips.
Mike Healy
2,451 PointsMike Healy
2,451 PointsI think that'd give a syntax error. You're in the method scope, so this.wheels = 4 is probably what you meant, but using the decreaseGear function isn't a good place for that anyway. That method may never be called. The prototype still needs a default number of wheels.