Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial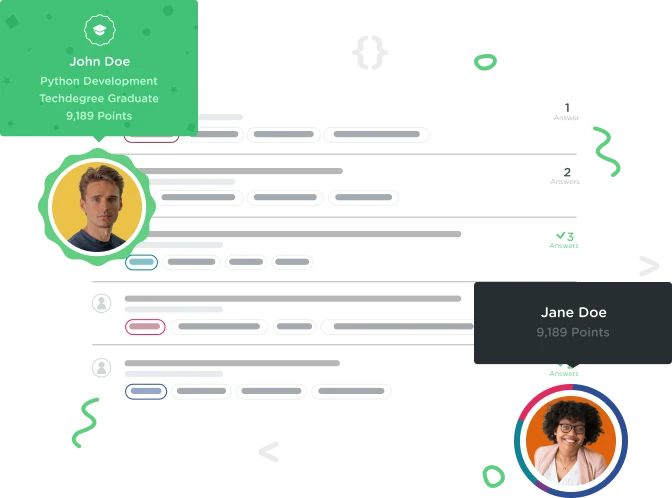
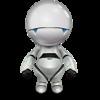
Joshua Montmeny
Courses Plus Student 6,135 PointsJavaScript Conditional Statement Basics
I'm working on building the Ultimate Quiz Challenge in the JavaScript Basics course. I've built a code block that runs the way I want it to, except for one glitch, here's the code:
var answerOne = true;
var guessOne = prompt( 'Who wrote the book For Whom the Bell Tolls: A. Edgar Allen Poe B. Ernest Hemingway C. Dave Eggers? (Please chose A, B or C).' );
if ( guessOne.toUpperCase() === "B" ) {
answerOne;
} else if ( guessOne.toUpperCase() === "C" || guessOne.toUpperCase() === "A" ) {
answerOne = false;
}
if ( answerOne ) {
console.log("correct");
} else {
console.log("incorrect");
}
So, the if/else statement seem to work fine, logging the appropriate response to the console; here's the problem though: If I type ANYTHING other than a, b, or c the answer isn't rejected but instead logs as 'correct' to the console!
How come? I thought the '===' would force the program to ignore anything that wasn't one of those three answers. What am I missing?
Thanks in advance!
2 Answers
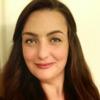
Jennifer Nordell
Treehouse TeacherJoshua Montmeny Hi there! First, you're doing great. I've reworked your code so that it works, but I'm going to go through why yours doesn't currently work. Here's the working version:
var answerOne = false;
var guessOne = prompt( 'Who wrote the book For Whom the Bell Tolls: A. Edgar Allen Poe B. Ernest Hemingway C. Dave Eggers? (Please chose A, B or C).' );
if ( guessOne.toUpperCase() === "B" ) {
answerOne = true;
} else {
answerOne = false;
}
if ( answerOne ) {
console.log("correct");
} else {
console.log("incorrect");
}
Your code starts with the assumption that the answer is true. And you're checking for A, B, and C... but nothing else. Which means Z will use true. And, thus, you get a correct result when using anything besides B or C. My code starts with the assumption that the answer is false. Then I check to see if the answer is equal to B. If it's not... I mark answerOne as false.
You have a line in there that just says answerOne; Now, I'm guessing that you believe that assigns true to answerOne, but that's not the case. We can use if(answerOne) to determine if the value is true. But if you want to set a value to true you have to explicitly assign it the value of true as I did in my code above. Hope this helps!
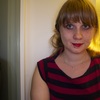
Karolin Rafalski
11,368 PointsTo elaborate on Jennifer's point. The if statement works like so: if (the thing in here is true or truthy){ do stuff in these curly braces }
Beyond the booleans true and false, Javascript has things that are truthy and falsely. Some falsely things are: 0 null undefined ""
Many many things in js are truthy. Any string that is not empty will by truthy, that includes "false", "0" and " ".
So when you have :
if ( answerOne ) {}
it is testing whether or not answerOne is truthy/true, and if it is either , it will go into the code inside your if statement.