Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial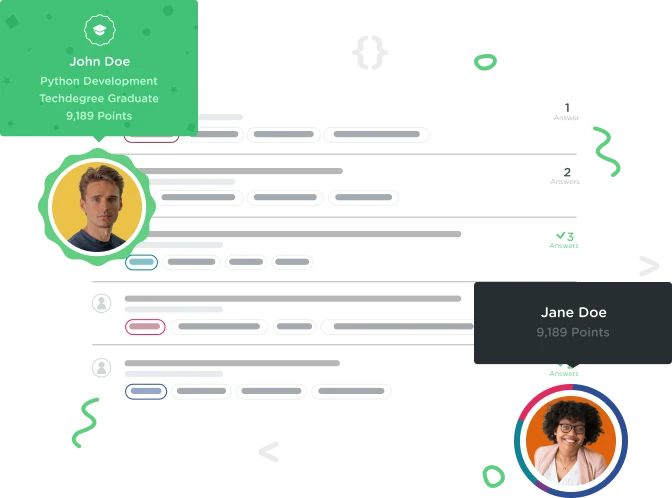
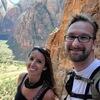
Jeremy Castanza
12,081 PointsJavascript - Difference between ParseInt and Number functions.
Hello,
I'm trying to understand the difference of when I would use Number and when I would use ParseInt in Javascript. If anyone can give me some guidance and clarity on this, I'd appreciate it.
Thanks, Jeremy
2 Answers
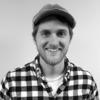
Eric Stermer
9,499 PointsParseInt is meant to change a string value that is a number into an actual number value.
console.log(parseInt("3")); // output will be the number 3 not a string
So this would come in handy when you are looking to use numbers gathered from an input value from the user in a form to do math, like in a calculator. So the number coming in a a string "37820" and to do math on it you need it to be a number 37820 and parseInt() will do that.
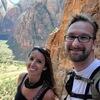
Jeremy Castanza
12,081 PointsThanks for the additional clarification, Eric.
So, is it fair to say that Number() is more robust and ParseInt() would largely be used for initial user input?
Thanks again, Jeremy

Stuart Elmore
84 PointsAs a rule of thumb, you shouldn't construct new base level classes (Number
/ String
/ Array
) etc. Good answer for that here: http://stackoverflow.com/a/1273936 - essentially the base levels are primitives
, as opposed to the class-level versions (i.e. new Number();
). Class level can be overwritten, so for example I could write this:
function Number (num){
return parseInt(num, 10) * 10;
}
Which would modify the Number
method:
new Number(10); // -> 100
new Number('5'); // -> 50
When creating a number, you should just define it as:
var myNum = 5;
const myForeverNum = 6;
let myLocalNum = 7;
However, if you're converting a string into a number (for example: when parsing an XHR request), you should always use parseInt
as then you can explicitly set the radix
level - this sets the radix (or base) of the number. This is very important too, since parseInt
will otherwise try and guess the radix to provide according to what you give it.
To demonstrate this:
parseInt('8') // -> 8
parseInt('08') // -> 8 in modern browsers (ECMAScript 5 removes octal interpretation), 0 in IE8
parseInt('0e0'); // -> 0
parseInt('0e8'); // -> 0
Mozilla recommends still using the radix even in ECMAScript 5 environments because:
Many implementations have not adopted this behavior as of 2013, and because older browsers must be supported, always specify a radix.
Rule of thumb
Always use the radix parameter in parseInt
:
parseInt(10, 10); // -> Base-10 = 10
parseInt(10, 2); // -> Binary = 2
parseInt(10, 8); // -> Octal = 8
parseInt(10, 16); // -> Hexadecimal = 16
parseInt('5fcf80', 16); // -> Treehouse's green colour becomes 6279040
// to get it back...
(6279040).toString(16); // -> '5fcf80'
Jeremy Castanza
12,081 PointsJeremy Castanza
12,081 PointsThanks Eric. I understand that aspect.
I'm just trying to figure out when a programmer would do the following:
console.log(parseInt("3"));
versus
console.log(Number("3"));
Eric Stermer
9,499 PointsEric Stermer
9,499 PointsSorry, Number() would be if you are looking to convert Native Objects of different kinds to a number instead of just a string. So parseInt() would only be for numbers in the format with "" around them, but Number() you can convert a boolean, a date, etc. Granted deciding between which one to use is up to you as a developer. Most of the time a parseInt() will be enough. hope this clarification helps