Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial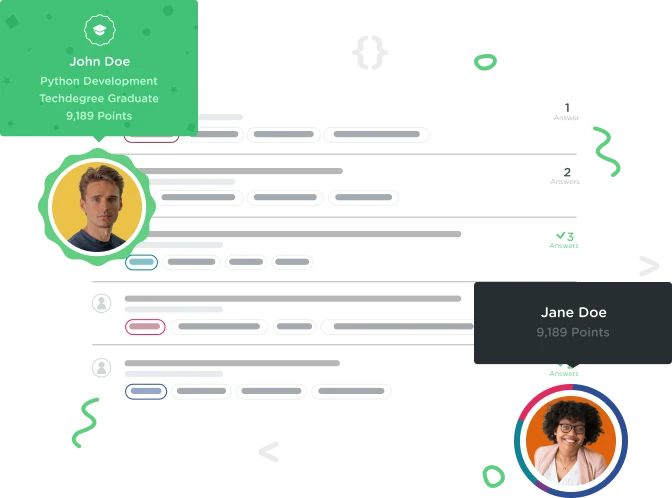
williamrossi
6,232 PointsJavascript FAQ section - toggle + to -
Hi all, I have created a FAQ section for a website I am making. When only the questions are shown upon load, when a question is clicked the answer is revealed and hidden when clicked for the second time.
I have appended a "+" to the end of each question, when this particular question is clicked I want the "+" to change to a "-"
I have tried a few ways of doing this but I am finding it hard to do. I already have a toggle in there which is showing the answer once clicked.
Thanks in advance.
3 Answers
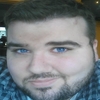
Marcus Parsons
15,719 PointsHey williamrossi, I made up a solution for you. I changed a couple things in the markup and re-vamped the jQuery you had.
1) I added a class of "answer" to all of the answers in your FAQ. It's good to use a class selector instead of a specific element selector, because if you decide that those specific elements should be divs or unordered lists or something else, you won't have to go back into the CSS to change it every single time you make a change.
2) I changed the appended span into a button. Spans can be clicked, but they have to be changed to block (or inline-block) level elements. You'll just have to do as Petros suggested and change its hover state to show a pointer for the cursor. That is why I changed it to a button so that it stands out, and you can tell that you can click on it. You can change the CSS on the button though to make them more to your needs if you don't like the standard button look.
3) The click function you had was operating on the section, not the specific paragraph that was being clicked. The click function is now displaying the correct paragraph. Also, you have to add the "parent()" function to the "next('p')" function because when you append an element to another element, the appended element becomes a child of that element. The "next()" function only selects sibling elements; therefore, there would be no next paragraph to select without using the "parent()" function to go back up to the paragraph in question (pun pun pun) because the next paragraph that we need to select is a sibling of the appended button's parent, not of the button itself.
You can copy this straight into a Workspaces HTML file and check it out.
<!DOCTYPE html>
<html>
<head>
<title>Toggle</title>
<style>
.question {
color: #009fdb;
display: block;
font-size: 20px;
margin: 15px 0 5px;
width: 100%;
}
.answer {
display: none;
}
</style>
</head>
<body>
<section class="faqs sections">
<h1>Frequently asked Questions</h1>
<p class="question">How long have you been involved in the fitness industry?</p>
<p class="answer">I have been a fully qualified personal trainer and involved in the fitness industry since 2005. I have worked in various gyms, including those on Cruise Ships and also worked internationally, in Australia, in particular, Melbourne.</p>
<p class="question">Who are your typical trainees?</p>
<p class="answer">I have trained everybody. Older, younger, fit and unfit. I have trained people with conditions starting with A all the way to Z.</p>
<p class="question">Who is your best success story?</p>
<p class="answer">I have many, many success stories. The one in particular that normally comes to mind when I get asked this is when I was working on cruise ships. I trained a lady who was around the age of 80. She walked with a hunched back, had absolutely no heel-toe action in her walk and used a stick. After a few posture pointers, and exercises to help bring her shoulders back and encourage a heel-toe action in her walk, she never used her walking stick again after that first session.</p>
<p class="question">What is your approach to training people?</p>
<p class="answer">Everything is goal dependent. It is important to also understand that everyone is an individual. Often people compare themselves to friends of theirs, or other people in the gym, but try to focus on your own goals and journey through fitness. Once that is understood, the exercise part I design is for that individual, but it normally follows a few parameters. Included in the workout are functional exercises within the ability of the individual. You will work hard to ensure a progressive overload, i.e. increasingly difficult workouts to ensure results. Combined with healthy nutrition, this will guarantee goals are achieved.</p>
<p class="question">What equipment do you use?</p>
<p class="answer">Anything and everything. This will include the following where possible: bodyweight, kettlebells, medicine balls, TRX suspension, battle rope, gymnastic rings, bosu, foam rollers, dumbbells, barbells, machine weights, and boxing gloves and pads. Anything for variety, function and fun! If you particularly like a specific piece of gym equipment we can always try to focus around that, so that you enjoy the session that little bit more!</p>
<p class="question">What are your qualifications?</p>
<p class="answer">Level 3 personal trainer, Sport and Exercise Science BSc with honours, Mat Pilates and Pilates on the ball, Spinning, Boxercise, Fitball trainer and I am fully insured.</p>
<p class="question">How do I get rid of my gut/bingo wings/fat in general?</p>
<p class="answer">I’ve included this in the FAQ because I get asked it so frequently. You can’t simply decide where to lose fat. Your body burns fat where it sees fit, depending on age, hormones and gender. To achieve that alluring 6 pack, there is always muscle there, but generally underneath a layer of fat. The key to a reduction in body fat lies within nutrition. On top of that, cardiovascular combined with resistance work is a great way to reduce body fat and tone/increase muscle mass. This helps to show through the muscles underneath and make them more prominent. There are other, not so straight forward ways where results may be seen faster!</p>
<p class="question">Finally, what made you get into personal training?</p>
<p class="answer">I have always been sporty; my main sport being basketball. I have also intermittently participated in rock climbing, jiu jitsu, karate and kick boxing. I fell naturally into this role, enjoying the social side of personal training and teaching classes.</p>
</section>
<script src="http://code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript" charset="utf-8">
$('.question').append('<button class="plus">+</button>');
$('.plus').click(function() {
$(this).parent().next('p').slideToggle('fast').css('display', 'block');
});
</script>
</body>
</html>

Petros Sordinas
16,181 PointsHi,
Update your javascript with something like this:
$('.faqs .question').append('<span class="plus">+</span>');
$('.faqs .question').click(function() {
$(this).next('p').slideToggle('fast');
question = $(this);
if (question.children().hasClass('plus'))
$(this).find('.plus').replaceWith('<span class="minus">-</span>');
else
$(this).find('.minus').replaceWith('<span class="plus">+</span>');
});
If the clicked object has a class of plus, replace the class with the minus class else replace with plus class. Hope it makes sense.
Petros
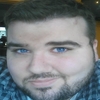
Marcus Parsons
15,719 PointsThe problem with using a span like this is that when the user gets on to the page, they won't know that you are supposed to click the plus sign because there is no indication that it actually does anything. People are used to seeing their mouse cursor change when you hover over an element that you can interact with.
If you replaced this with an anchor link and pass in the "event" object to the click function, then use event.preventDefault()
, this would be a nice solution. I like it!

Petros Sordinas
16,181 PointsYou are right Marcus. I just gave him a solution based on the code williamrossi posted in his fiddle without changing any html or css, as he may have had other reasons for using spans.
For example, he could do this in his stylesheet:
.question:hover {
cursor: pointer;
}
That way, when the user hovers over the questions, the cursor will change...
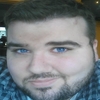
Marcus Parsons
15,719 PointsHaha Petros, thank you for reminding me of that! I totally forgot about being able to use that. I legitimately, 100% forgot about that. hahaha

Petros Sordinas
16,181 Points:) You should see what I keep forgetting...
williamrossi
6,232 PointsThanks for the responses guys, nice work!