Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial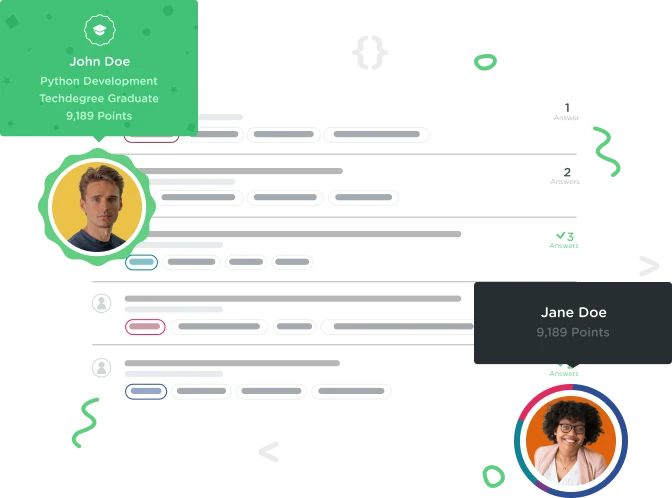
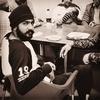
Sandeep Singh
6,083 Pointsjavascript 'for' loop
what are the uses of, for loops as ' for(key in person){ } ' like structure with objects in javascript
1 Answer
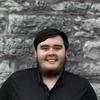
Michael Hulet
47,913 PointsA for in
loop iterates over every key in an object and gives it to you. Consider this code:
const names = {
first: "Michael",
middle: "David",
last: "Hulet"
}
for(const position in names){
console.log(`Your ${position} name is ${names[position]}.`);
}
This is the console output of that script:
Your first name is Michael.
Your middle name is David.
Your last name is Hulet.
It helps you operate on all the keys/values in an object without knowing what they are head of time. Note that if you use a for in
loop on an array, it will give you all the indexes in the array, but it will also still give you the length
property, so you shouldn't use a for in
loop to iterate over an array, unless that's the functionality you want. ECMAScript 2015 added a different loop to iterate over arrays: for of
. This will give you all the values that are marked as iterable in an object. For arrays, that's just the values of an array, like this:
const numbers = [1, 2, 3];
for(const number in numbers){
console.log(number * 2);
}
The console output of that script is:
2
4
6