Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial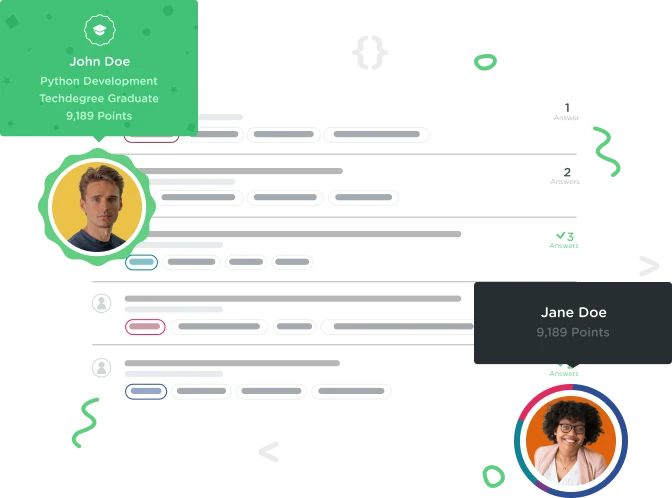
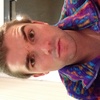
Garan Guillory
Courses Plus Student 19,291 PointsJavaScript Foundations / Stage 1: Variables / Shadowing Quiz
var person = "Jim";
function whosGotTheFunc() { var person = "Andrew"; }
person = "Nick"; whosGotTheFunc(); console.log(person);
Nick
var person = "Jim";
function whosGotTheFunc() { person = "Andrew"; }
person = "Nick"; whosGotTheFunc(); console.log(person);
Andrew
Why does the console log out "Nick" in the first example and not the second? Doesn't the (person = "Nick";) change the global variable "person" on both occasions?
8 Answers
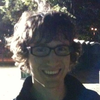
Ben Rubin
Courses Plus Student 14,658 PointsIn the first example, the function says var person = "Andrew"
. When you use the keyword var
you're creating a new variable. You now have two person
variables; one in global scope and one whose scope is limited to the function whosGotTheFunc
. When you set person = "Andrew"
inside of whosGotTheFunc
, you're accessing the local person
variable, so the change you make doesn't affect the global person
variable.
In the second example, the function doesn't use var
, so it doesn't declare its own variable. Since there is no local variable named person
, the statement person = "Andrew"
is referencing the global variable person
. So when you call whosGotTheFunc
, you're changing the global person
variable from "Nick" to "Andrew".
If a variable that is declared inside of a function has the same name as a global variable, you are "shadowing" the global variable. In this situation, any references to the variable use the local variable instead of the global variable.
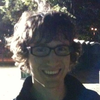
Ben Rubin
Courses Plus Student 14,658 PointsYou are correct in saying that console.log
will log the latest value of person
. However, keep in mind that whosGotTheFunc
is updating person
. Once you call whosGotTheFunc
in example 2, that's updating the value of the global variable person
to "Andrew".
Keep in mind that the function doesn't actually do anything until you call it. Just declaring the function and its body doesn't change any variables until you actually call the function.
Here's the order in which the variables get set in example 1:
- Global variable
person
is set to "Jim" - Global variable
person
is set to "Nick" - Local variable
person
withinwhosGotTheFunc
is set to "Andrew" (from the function call) - Log the global variable
person
, which is "Nick"
And in example 2:
- Global variable
person
is set to "Jim" - Global variable
person
is set to "Nick" - Global variable
person
is set to "Andrew" (from the function call) - Log the global variable
person
, which is "Andrew"
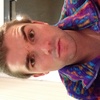
Garan Guillory
Courses Plus Student 19,291 PointsThanks for taking time out of your day to clarify this for me. Here is where I am confused:
person = "Nick";
WhosGotTheFunc();
console.log(person);
The above code calls the function WhosGotTheFunc and then logs the variable person. Shouldn't the console log the latest "update" to the variable person, which is "Nick" ? (I am talking about the second example here. I understand that the var person = "Andrew" is untouchable because it is defined inside of a function.) Does the function have precedent? Why is "Andrew" logged when person = "Nick" is defined afterwords? Sorry, I've read this email 100 times and re-watched the video on numerous occasions, and the second example is still not clear.
Do you see where I am confused? Thanks in advance.
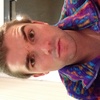
Garan Guillory
Courses Plus Student 19,291 PointsYou're good. Thanks Ben, you clarified it all.

adam costa
456 Points+1 Thanks Ben, your explanations really helped.

Nick Stoneman
10,093 Points+1 thanks too. Your answer helped.

kieron kirkland
3,074 Points+1 that's really helps, especially understanding how the variables change depending on when the function is called
Jeff Froustet
16,015 Points+1. "Keep in mind that the function doesn't actually do anything until you call it." That's what did it for me.