Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial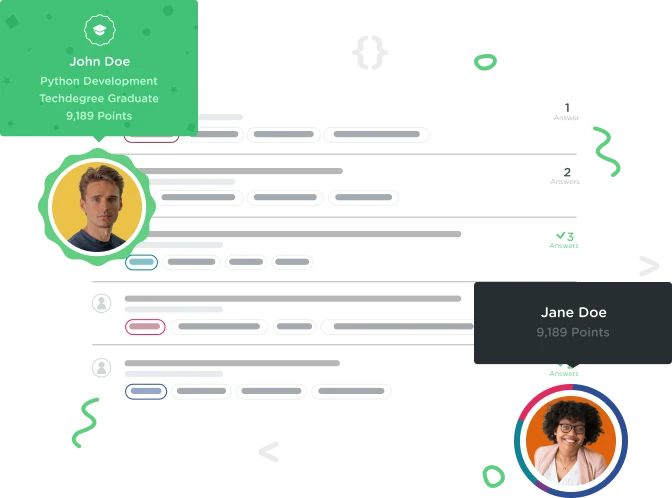
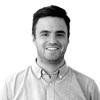
Christopher Sukovich
13,382 PointsJavascript Foundations / Variables / Basics: Code Challenge
Challenge 1 of 3: Move the script tag where it'll get executed after the page loads.
<!DOCTYPE html>
<html lang="en">
<head>
<title> JavaScript Foundations: Variables</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
<script src="myscript.js"></script>
</head>
<body>
<h1 id="title">JavaScript Foundations</h1>
<h2>Variables: Basics</h2>
<div id="container">
This is a div with the id of "container"
</div>
</body>
</html>
I'm assuming all I need to do is move the <script>
tag to the bottom of the body, just before it closes, but when I do, I receive this error:
Bummer! There was an error with your code: TypeError: 'null' is not an object (evaluating 'container.style')
Here is the example js:
/* JavaScript Foundations: Variables */
var bgColor = "";
var textColor = "";
var container = document.getElementById('title');
container.style.background = bgColor;
container.style.color = textColor;
Is there an error in the example code?
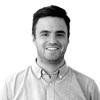
Christopher Sukovich
13,382 PointsTwiz T - That's what I did, but I just wanted to provide the example code as is - the error pops up anyway, and I'm thinking it's something in the example js.
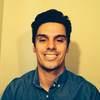
Twiz T
12,588 Pointsahh thanks for fixing the formatting. It doesn't look like bgColor and textColor variables are being set to anything which explains the error.
But yeah you're right if thats how the challenge engine was loaded is seems to be a mistake; maybe just fill in colors for the 2 variables for the sake of passing the first task and submit a ticket to treehouse saying the example.js was being loaded without any values for those 2 variables.
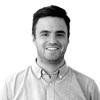
Christopher Sukovich
13,382 PointsThanks Twiz T ! Still getting the error, unfortunately - even with variables filled in.
Jim Hoskins , any ideas?
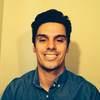
Twiz T
12,588 PointsSounds like a bug. I just went to that challenge and this worked for me.
<!DOCTYPE html>
<html lang="en">
<head>
<title> JavaScript Foundations: Variables</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1 id="title">JavaScript Foundations</h1>
<h2>Variables: Basics</h2>
<div id="container">
This is a div with the id of "container"
</div>
<script src="myscript.js"></script>
</body>
</html>
and the myscript.js file
/* JavaScript Foundations: Variables */
var bgColor = "";
var textColor = "";
var container = document.getElementById('title');
container.style.background = bgColor;
container.style.color = textColor;
Try reloading the challenge in a different browser session?
3 Answers
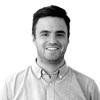
Christopher Sukovich
13,382 PointsTwiz T it ended up being a browser issue with Safari - works in Chrome, thanks!

Jason Anello
Courses Plus Student 94,610 PointsHi Christopher,
For task 1 you should not be doing anything yet with the js file.
The challenge wants you to simply move the script
tag which loads the js file, <script src="myscript.js"></script>
, from the head
section to right before the closing body tag </body>
<script src="myscript.js"></script>
</body>
</html>

Jason Anello
Courses Plus Student 94,610 PointsDid you by chance put it there but also left it in the head
section? That does produce the error you're getting. If you have it in both places.
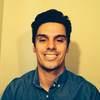
Twiz T
12,588 PointsGah of all the things to have to think about!
Twiz T
12,588 PointsTwiz T
12,588 PointsI think you need to move the script before the closing body tag