Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial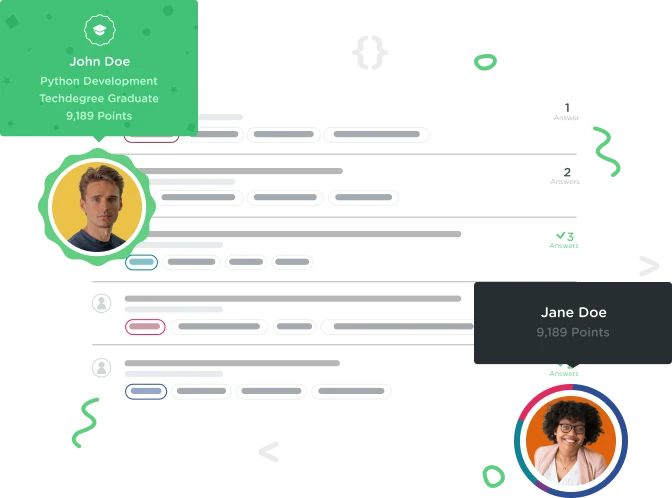
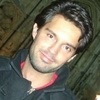
Benjamin Singh
15,248 PointsJavaScript function challenge
Hi all!
I just want to know what I am doing wrong here. The challenge is:
"Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in."
This is my code:
function arrayCounter (array) {
if (typeof array === 'array') {
return array.length;
}
if (typeof array === 'string' || typeof array === 'number' || typeof array === 'undefined') {
return 0;
}
}
The error message is:"Bummer! If I pass in an array in to 'arrayCounter' of '1,2,3' I get undefined and not the number 3."
The code looks ok for me :)
8 Answers
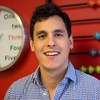
Nick Ocampo
15,661 PointsJavaScript doesn't recognize "array" as a valid value for the typeof operator. You're actually looking for "object" as your result. I would also use an else clause for that last part. I tried this in the console and it worked:
function arrayCounter (array) {
if (typeof array === 'object') {
return array.length;
}
else {
return 0;
}
}
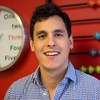
Nick Ocampo
15,661 PointsThanks for pointing that out! I completely agree with your answer, very cool!
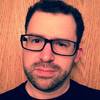
Chris Shaffer
12,030 PointsThe Challenge in question is terribly explained, horribly irrelevant to the point in the course and uses a method not previously defined in JavaScript Foundations.
Combined with the terrible teaching for this particular course, this poorly worded question has me almost pulling my hair out.
I've never been so frustrated with Treehouse courses until I started this track by Jim Hoskins. He jumps all over, doesn't explain parts of what he's doing or why and then fails to provide (more than once) the methods needed to complete the challenges.
It's enough for me to call it quits with TH's Javascript courses. Everything else has been great, but this is ridiculous.

Chris Konchalski
5,914 PointsAgreed... it seems they at one point re-created the JS course and used a mashup of older courses, nothing is fluid

Paul Rangel
4,935 PointsCompletely agree. The JavaScript courses with Hoskins are absolutely brutal.

David Forero
5,492 PointsI am wondering if there is a way to complete the challenge with the code provided in the Javascript Foundations course only. If I am correct that is the main purpose of these challenges.
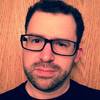
Chris Shaffer
12,030 Points@Chris Upjohn Your answer may be technically the best but is not the best answer in the context of the challenge because it uses the Object method which hasn't been taught at this point in JavaScript Foundations, at least as it appears in the Front End track.
More importantly, @David Forero points out the obvious: people are confused and coming to the forums due to a lack of consistency in this JS Foundations track.
Learning code in a haphazard method of "you're doing it wrong do this instead" bucks the trend of TH teaching in a methodical way where the core concepts are learned first.
So while you're answer is interesting, for those of us at this point in the track, it's also wrong.
Note that I also responded to you on another thread saying basically the same thing.

Chris Shaw
26,676 PointsI fully understand and in context it's not correct but I supplied the code as a way of showing better ways to achieve the same result without falling into one of JavaScript's nasty inheritance traps, however in saying that the code I used in question is all fairly straight forward and can be understood in one day of reading the documentation over at MDN.
I in no way am forcing anyone to use this code while going through the course, I simply improved the response to the question which is fairly vague to begin with as it offers no real hints as to what code you could write and receive an success message.
Note that I also responded to you on another thread saying basically the same thing.
My mind has been on and off for the past few days so I've more than likely just missed your reply, thanks for the reply though as I think these are the types of discussions that need to happen in order for new comers to get up front assistance rather than endlessly walking the wonders of the internet which can be painful.
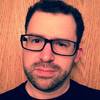
Chris Shaffer
12,030 PointsIt'd be very nice if we could get an answer from the instructor as to what the intended answer is. I copied in some code
var myArray = [1,2, "bob", this]
function arrayCounter (example) {
if (typeof example === 'string'){
return 0;
}
if (typeof example === 'number'){
return 0;
}
if (typeof example === 'undefined'){
return 0;
}
return example.length;
};
arrayCounter(myArray);
to pass, rather than bang my head against the wall trying to figure a way to write the code with "known" JS vs. Mr Upjohn's answer or Nick Ocampo's answer.
They're both straight-forward, but the problem is that we don't need the ANSWER we need to understand the concept.
I'm going to give up on expecting Treehouse to explain this further as I think I'll continue to get the 10 ways to do it that don't apply in context, which misses the entire point that it's hard to learn when you're frustrated and then someone comes along and says, "do it this way" but you have no idea why you do it that way and it's NOT the way you've been taught.
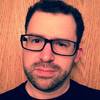
Chris Shaffer
12,030 PointsMaybe @Jim Hoskins could give us a real answer?
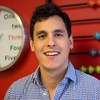
Nick Ocampo
15,661 PointsHey Chris, I've been busy working on a project all week but here's how this whole thing works:
First, you're declaring a variable called 'MyArray' and storing an array inside of. The array has 4 values; two are numbers, one is a string and the other is a variable--but that doesn't really matter for the purpose of this exercise. They could be anything, what matters is that it is the variable is storing an array of values.
Let's skip the function and go straight to the last line where the function actually gets called. By writing the name of a function that has been declared elsewhere (in this case, in the statement right before), you're effectively calling the function (in other words, asking the program to execute that function for you). The function passes one parameter in between the parenthesis. In your case, you are passing the variable 'MyArray' that you declared earlier. Therefore, the function will execute and use the variable 'MyArray' as its only parameter.
Now we're ready to talk about what's happening in the function when it gets called. When you write 'function arrayCounter(example) {}' you are declaring a new function, telling the program that it's called 'arrayCounter' and telling it that it will accept one parameter in the parenthesis. You are calling it 'example' here, but you could call it whatever you want, as long as you use the same name when you use it in between the curly braces.
Between the curly braces is where you tell the program what you want to happen when the function gets executed. In this case, you have three conditional statements that ask if the parameter you pass when calling the function (in your case, that will be the 'myArray' variable) is a string, a number or undefined. If any of these is true, the function will return a value of 0. If the program runs into an If statement that is not true, it just skips it. So in your case, it will go to the first statement asking if it's a string. Your variable 'myArray' is an array, so it will skip that if statement and ignore the part where it tells it to return 0. Then it will go to the next asking if it's a number. It's a variable, so it will ignore that as well. Same thing for the last if statement asking if it's an undefined value. Lastly, after all the if statements, there is a line that says 'return example.lenght'. This will return the length of whatever you ran through the arrayCounter() function. This line will execute only if it managed to skip all the if statements. So basically, what your code is doing is checking if the variable you passed when you called arrayCounter was a number, if not, check if it's a string, if not, check if it's undefined. If none of those are true, then give me the length of what you have.
I should point out that all of this is covered in those videos. It's not my obligation or anyone else's to give you the answers to your questions. We're just doing it to help others out that are learning just like us. Next time, try to have a better attitude when you have a problem. Rewatch the videos, look things up online and try things out yourself. I agree that that particular question was tricky, but to say it was horribly irrelevant seems a little out of place coming from a guy that is doing his first JavaScript challenge. Put your best attitude to learning and you'll find the answers.
Best of luck.
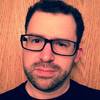
Chris Shaffer
12,030 Points1) Never asked you for an answer, but input is appreciated.
2) I'm not the only person who thinks the Jim Hoskins' courses are horribly explained.
3) Please go take the actual JS Fundamentals course, up to and including this particular question. You will find the videos that lead to this do not actually properly explain the methods that YOU and Chris Upjohn gave. I'm not saying your answers are wrong; they are out of the context of what has been taught in the course up to this point. This was my only criticism of you or your answer, and it stands, I don't apologize and if you don't understand why that affects people's ability to learn, maybe you should avoid answering questions.
My attitude is indicative of the lack of quality instruction in these particular videos. Needless to say, I'm looking elsewhere for continuing with JS until I see something at Treehouse that is worth my money related to JS. Maybe once I up my JS game at Code School or somewhere else, I'll return.
Up to this point, as I stated before, Treehouse has been fairly solid in terms of instruction, the challenges have been in sync with the instruction and the material has overall been educational.
I don't feel as though I've learned much, more so struggled to survive, Jim Hoskins' courses and ultimately and most importantly: I haven't learned anything I didn't already know from Codecademy, which is sad.
I've suspended my account and moved over to Code School to give it a whirl. I'm sure I'll be back to TH, but probably not for any course where I see Hoskins' name attached.
Benjamin Singh
15,248 PointsBenjamin Singh
15,248 PointsThank you for the quick response!
Chris Shaw
26,676 PointsChris Shaw
26,676 PointsHi Nick,
Checking the type of the value against an object is a valid answer but it's not the best one, I explained why in my answer to this question in another thread.
https://teamtreehouse.com/forum/stuck-on-stage-5-functions-quiz#show-answer-630332