Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial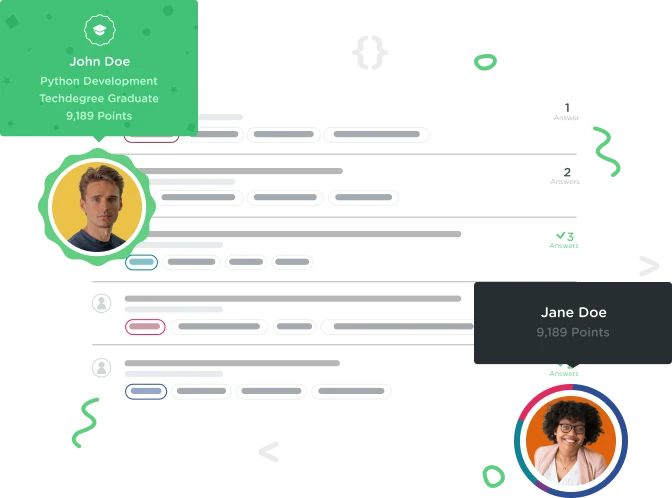
Darrin Banfield
8,244 PointsJavaScript functions challenge
create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in. I have: function arrayCounter (array) { if (typeof array === 'string', 'number', 'undefined') { return 0; } return array.length; }; What am I doing wrong?
4 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Darrin,
The syntax of the language doesn't allow what you're trying to do. You are treating 'undefined', 'number', 'string' as a kind of list and you are trying to see if the typeof array
is any of those things. Javascript doesn't have a construct like that to my knowledge. Other languages like Python will let you do something like that with slightly different syntax.
For this problem you actually have to compare to each type individually and you can combine then with the logical OR operator. Which is two pipe characters ||
function arrayCounter (array) {
if (typeof array === 'undefined' || typeof array === 'string' || typeof array === 'number') {
return 0;
}
return array.length;
}
As others have pointed out in other posts, this function is not fool proof. For example, if you passed in a function as a parameter then it's going to try to return the length of that function.
For the time being, this is what they wanted you to come up with though.
I hope that clears it up for you.
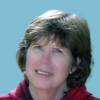
Joannie Johnson
7,997 PointsThanks for posting this - it works!

Jason Anello
Courses Plus Student 94,610 PointsThis is revised code that is more in line with what is taught in the video. I'm not sure if the logical OR operator was covered up to this point. Please leave a comment if you do remember it being covered.
The single if
statement can be rewritten as 3 simpler if statements that are familiar from the video.
function arrayCounter (array) {
if (typeof array === 'undefined') {
return 0;
}
if (typeof array === 'string') {
return 0;
}
if (typeof array === 'number') {
return 0;
}
return array.length;
}
So we check for each of the 3 types in separate if
statement and return 0
if any are true. Otherwise, we return the length. It requires a little more code and there is some repetitiveness vs. the OR operator but this should be more familiar from watching the video.
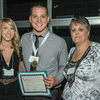
Matthew Ludwigs
11,197 PointsOkay so I think I have it. Or at this worked for me:
function arrayCounter(a) {
if (typeof a === "object" && a.length > 0){
return a.length;
}
else if (typeof a === "string", "number","undefined") {
return 0;
}
else {
return "This function is jacked up";
}
}
I believe the first typeof statement makes sure the array that is passed to the function is different then the other types of data that could be pass through, and checks the length to see if the array is longer then 0. I know if you just had a.length > 0 and passed it a string, this method would still work and now your function is not returning 0, but actually returning the length of the string. When you put typeof a === "object" a string will no longer be true and the function will move to the next statement to evaluate it.
To test the way typeof works I wrote:
x = "bob"
console.log(typeof x)
and the console gave me "string." This can be strange(at least to me at first) because JavaScript does have a string object. I hope this helped.
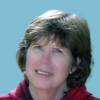
Joannie Johnson
7,997 PointsYou will have my eternal gratitude if this works! I only wish I knew what the || is about? It's yet another new thing for me.
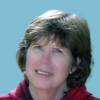
Joannie Johnson
7,997 PointsTAKE A BOW -- it worked! But I don't understand why it works. This seems to have very little to do with the lecture. Does the instructor make up the challenge tests, or does some other party do it? I really wonder some time.
Anyway, thanks. I really appreciate seeing an answer that works.

Jason Anello
Courses Plus Student 94,610 PointsI reviewed the video and you're correct. I'm going to add a comment to my answer with revised code that will be more in line with what is taught. I skimmed over all the stages and I'm not sure if the OR operator was ever covered. I can't remember from when I completed this.
The ||
is the logical OR operator. You can combine boolean expressions with this and if any of the expressions are true, then the entire thing is true. In the code we're checking if it's undefined
OR a string
OR a number
. So if any of those things are true the entire if
condition is true and code for the if
will be executed. In this case, return 0
Adam Duffield
30,494 PointsAdam Duffield
30,494 PointsTo add to this, I'm also stuck with Javascript on the exact same issue,I'm 100% sure all my coding is right but it keeps saying that the function "arrayCounter" has not been declared despite that being the easiest part to do!