Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial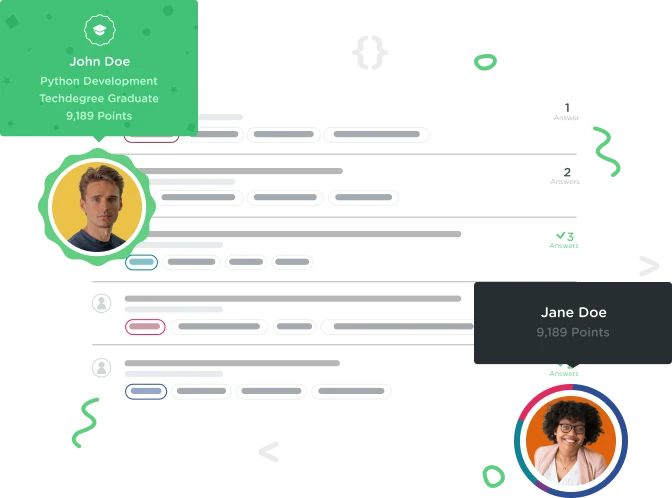
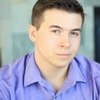
Anthony Williams
10,813 PointsJavaScript Functions first code challenge
I am trying to determine if the array name itself is being looked at as a the variable name or the contents of the array. If it is being looked at as the contents of the array, how do you check if the functions input is a string, number, or undefined without looking at the contents within the array?
link to the code challenge: http://teamtreehouse.com/library/return-values
function arrayCounter(a){
if (typeof a === "string", 4, 'undefined'){
return 0;}
return a.length;
}
``` ???
If possible, please explain. Thanks : )
3 Answers

notf0und
11,940 PointsYou'll need separate "typeof" checks for each type.
if (typeof a === "string" || typeof a === "number" || typeof a === "undefined")
Should work. If any of those are true, that will return 0. Otherwise, return the length of the array.

Michael Miller
5,747 PointsI believe the best answer is to use the instanceof operator. For example:
if(array instanceof Array){
return array.length;
}else{
return 0;
}
This will return 0 for anything other than an array, and return array.length if it is an array.
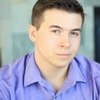
Anthony Williams
10,813 PointsThanks Mike. Crazy how many ways you can solve a problem. I did notice with the "typeof" operator it recognizes the array as "object". "Array" is a key word in JS right? so when declared with "instanceof" it can differentiate between different object types?
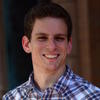
Jeff Lange
8,788 PointsExcellent solution! Unfortunately, if you're expecting to be able to solve the challenge only with what you've been taught in the preceding videos (like myself), then you feel dismayed that they never taught you the "instanceof" method.

Michael Miller
5,747 PointsGood questions Anthony. I had to look this up, but I believe the best answer to your original question is to use Array.isArray(object). The typeof operator can only return one of seven types (undefined, number, string, boolean, symbol, function, object). It cannot return what type of object it is, only whether it is or isn't one. My original suggestion, instanceof, will work most of the time since it checks to see if the object compared is in it's prototype chain. In other words is myArray an instance of the Array object. From what I've read there are some loopholes which could make instanceof return a bad result. (Don't ask me to explain them I don't really understand what they were saying). Array.isArray() is supposed to be a bullet proof way of testing whether an object is an array or not. I hope this all makes sense.
If you're interested check out these articles:
Determining with absolute accuracy whether or not a JavaScript object is an array
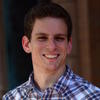
Jeff Lange
8,788 PointsGood answer again!
I wish they had taught us the stuff in the videos they are expecting us to be able to solve...sheesh. :D

Michael Miller
5,747 PointsAgreed. I think instanceof was very briefly mentioned, but I think they should teach us how and where to look for more information when we're stuck.
Anthony Williams
10,813 PointsAnthony Williams
10,813 PointsThank you Bryan. Very helpful!!