Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial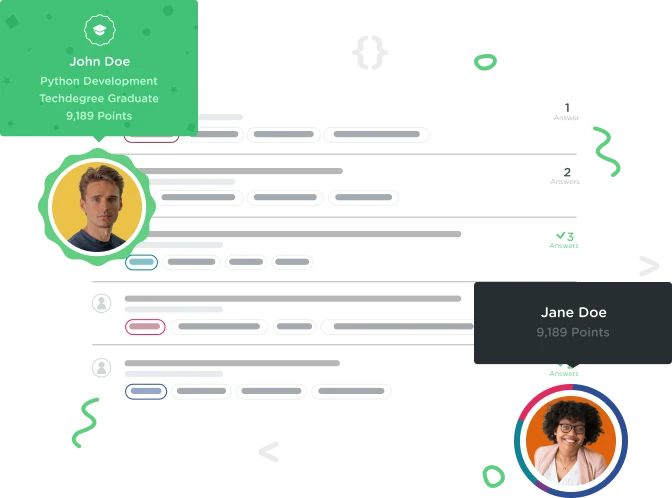
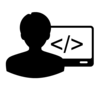
Abe Layee
8,378 PointsJavaScript Global Variable
I am current working on my JavaScript skill. However, I came across some bug or whatever it may be called. When I had a global varialbe, the setTimeout() function wasn't running every 1 second until I placed the global variable within the function itself. Can anyone please tell why the setTimeout wasn't working? Last but not least, my diem variable is not changing to PM or AM.
This code is fine with the global variable being inside the function
var diem = "AM";
function DisplayDateAndTime(){
var currentTime = new Date();
var hour = currentTime.getHours();
var minutes = currentTime.getMinutes();
var seconds = currentTime.getSeconds();
if (hour === 0) {
hour = 12;
} else if(hour > 12) {
hour = hour - 12;
diem = "PM";
}
if (hour < 10) {
hour = "0" + hour;
}
if (minutes < 10) {
minutes = "0" + minutes;
}
if (seconds < 10) {
seconds = "0" + seconds;
}
var Eltime = document.getElementById('time');
Eltime.textContent = hour + ":" + minutes + ":" + seconds + "" + diem;
Eltime.innerHTML = hour + ":" + minutes + ":" + seconds;
setTimeout(DisplayDateAndTime,1000)
}
DisplayDateAndTime();
This the global variable part. The setTimeout is not working. I don't know why.
var diem = "AM"; // global variable
var currentTime = new Date(); // global variable
function DisplayDateAndTime(){
var hour = currentTime.getHours();
var minutes = currentTime.getMinutes();
var seconds = currentTime.getSeconds();
if (hour === 0) {
hour = 12;
} else if(hour > 12) {
hour = hour - 12;
diem = "PM";
}
if (hour < 10) {
hour = "0" + hour;
}
if (minutes < 10) {
minutes = "0" + minutes;
}
if (seconds < 10) {
seconds = "0" + seconds;
}
var Eltime = document.getElementById('time');
Eltime.textContent = hour + ":" + minutes + ":" + seconds + "" + diem;
Eltime.innerHTML = hour + ":" + minutes + ":" + seconds;
setTimeout(DisplayDateAndTime,1000)
}
DisplayDateAndTime();
6 Answers
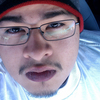
Chyno Deluxe
16,936 PointsThe reason is simple.
When you set the variable currentTime as a global variable, more specifically outside the scope of the DisplayDateAndTime function. You are only asking for the currentTime once. Therefore, all the variables inside the DisplayDateAndTime function, (hours, minutes, seconds), have only the initial time when the script currentTime was called.
However, when you place the currentTime variable INSIDE the function, when the setTimeOut runs it is making another call for the currentTime every second. This gives the function a new time to run the rest of the code and display the current time.
I hope this helps.

Dan Oswalt
23,438 PointsGlobal variables are constants in Javascript, they can't be changed, they are immutable. They are generally to be avoided (some would say always) because you won't get the behavior you expect.
In your example, both of the global variables you have are things that are highly mutable, so you don't want them as constants. So there's not a good reason to declare them outside of the scope of your function.
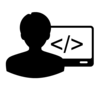
Abe Layee
8,378 PointsI added the innerHTML for ie. Thank you bro
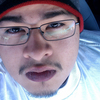
Chyno Deluxe
16,936 PointsAnytime! Just add the + deim to your innerHTML then. Glad I could help.
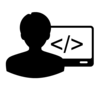
Abe Layee
8,378 PointsChyno Deluxe , personal question, how you got good at js? I am trying to focus on raw js before I do Jquery.
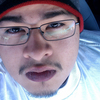
Chyno Deluxe
16,936 PointsI'm still learning. I've only been studying JavaScript for roughly 6 months so I'm not an expert by any means but I can tell you that you are making a smart decision starting with plain javascript before jQuery. That is the same path I took and It's helpful.
But honestly, I learned alot from the Full-Stack JavaScript course but I was still unsure of alot at the end so I took a look at youtube videos.
Here are a few playlist from LearnCode.Academy
Watching the playlist above gave me alot of Mind-Blown moments and got me familiar with alot of what I know now. The best way to learn is to put time in and practice with small projects. I've made two small projects using plain JS that you can checkout. To-Do List and JS Form Validator
I hope this helps you too.
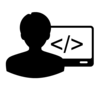
Abe Layee
8,378 PointsAlright, ty again bro:D
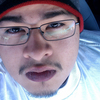
Chyno Deluxe
16,936 PointsAnytime. If you ever need help again feel free to tag me. If I can help, I will =)
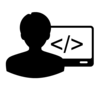
Abe Layee
8,378 PointsAlright bro
Abe Layee
8,378 PointsAbe Layee
8,378 PointsThat makes sense now but why is the diem var not working. I don't see Am or Pm in my clock.
Chyno Deluxe
16,936 PointsChyno Deluxe
16,936 PointsAhh! Whats happening is you are overwriting your time in your script. Just remove the .innerHTML and it should work. Your innerHTML overrides the .textContent so because it doesn't include the + diem. It doesn't output the PM/AM.