Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial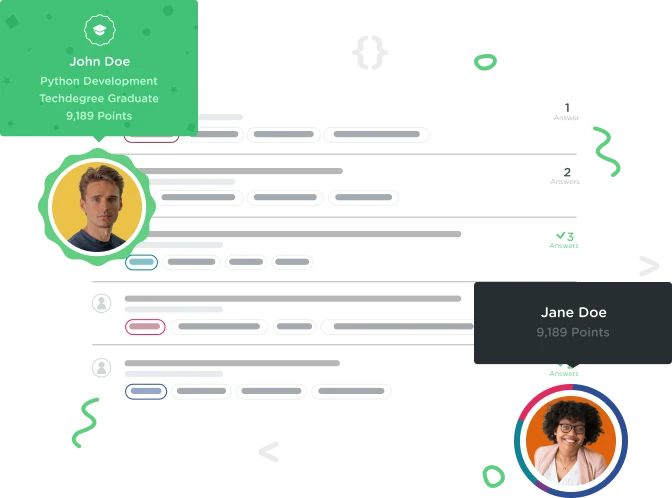
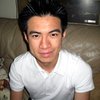
jason chan
31,009 Pointsjavascript - higher order functions
function noisy(f) {
return function(arg) {
console.log("calling with", arg);
var val = f(arg);
console.log("called with", arg, "- got", val);
return val;
};
}
noisy(Boolean)(0);
Can you explain to me what is happening here kind of lost with these higher order functions?
EDIT: Changed the code language markup for better readability. - Dane E. Parchment Jr. (Moderator)
2 Answers
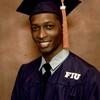
Dane Parchment
Treehouse Moderator 11,075 PointsAlrighty then let's help you out!
A high-order function is at it's most basic is simply a method/function that takes in either multiple functions or returns a method. This is a common function among programming languages, and in most languages is referred to as a lambda expression, which takes its roots from lambda calculus (which I find quite interesting and great to understand if you are a computer scientist/software engineer).
Let's break down the example
Alright so the first part of the script is like any function that you have ever seen before, what it does create a function called noisy, and then sets a parameter that is an arbitrary f.
function noisy(f) {
}
Now let's add the parts that make this more interesting, instead of going your typical route of using a function (code, followed by return statement). We are going to go straight for the return statement and instead of returning a variable or value, we will return a function. This is where the high order part is starting to come in. But wait...the weird part ( I think it's the cool part) hasn't even started yet, in the next step we are going to make this a high order function.
function noisy(f) {
return function(arg) {
}
}
Whoa! Did you catch that! We used f as a function in the body of the noisy's return function! But wait, how is this possible and we didn't specify what f is? Well that is the beauty of a high-order function. We can make f any type of function based on the parameter that we give it. So theoretically we can make the f parameter any type of function and it would accept it. In this case the parameter is Boolean, which when given a value will determine if it is true or false! When we make the function call, we first call the function, in this case noisy, and give it the parameter, in this case Boolean. The next set of curly braces is the function call for the return value of noisy, which as a parameter of 0.
function noisy(f) {
return function(arg) {
console.log("calling with", arg);
var val = f(arg);
console.log("called with", arg, "- got", val);
return val;
};
}
noisy(Boolean)(0);
The output will look like so (ignore the code comments):
/**
calling with 0
called with 0 - got false
**/
If you need any extra help free feel to respond! I will be glad to help, here are some resources to help you out further
- Lambda Calculus I find this very interesting, as a computer science major I have learned a lot about programming through lambda calc can be quite hard to master though.
- High Order Function Some more examples of high-order functions.
- Did you know that high-order functions occur if the real-world too! We just can't see them, a derivative is actually a high-order (lambda) expression, and as such many different physics concepts utilize derivatives and in turn high-order functions! - Random tidbit of info...not important.
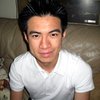
jason chan
31,009 Pointsthanks man, the thing is i never took lambda calculus so I'm very lost.
// higher order function
// this calls out side
var math = function (y) {
return function () {
return console.log(1 + y);
}
}
// first parent calls outside func, second parent calls inside func
math(1)();
var mathTwo = function () {
return function (x) {
return console.log(x + 2)
}
}
mathTwo()(2);
// this is example 3 of higher order functions
var mathShree = function () {
return function () {
return function (x) {
console.log(x)
}
}
}
mathShree()()(1);
But this is what i came up with higher order functions.
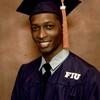
Dane Parchment
Treehouse Moderator 11,075 PointsYeah, I think you got it though! I mentioned lambda calculus mainly so that you can know where the idea for high-order functions came from, and if you were a math geek, then maybe it could help you figure it out a bit easier. You don't actually need to know lambda calculus to figure out how to use high-order functions and it looks like you figured it out.