Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial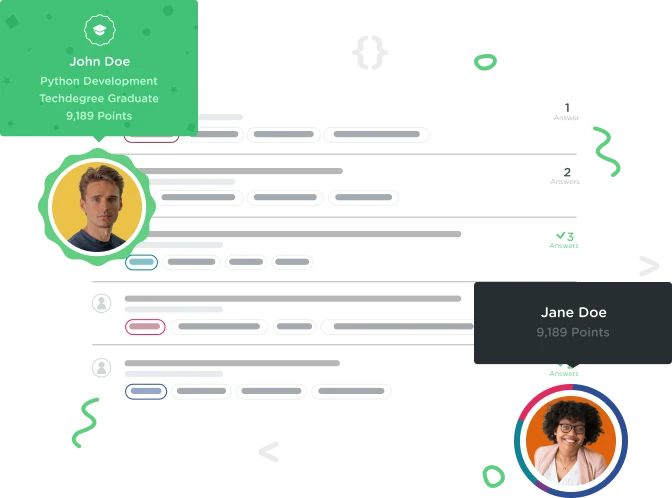

Kathryn Notson
25,941 PointsJavaScript Loops, Arrays, and Objects: Create a do...while Loop
O.K. I've been thrown for a loop on this Code Challenge. (Yes, it's a pun!) What am I missing? The var secret = prompt( "What is the secret password?"); is defined.
var secret = prompt("What is the secret password?");
do {
secret = prompt();
} while ( secret !== "sesame" );
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
4 Answers
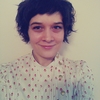
Kristopher Van Sant
Courses Plus Student 18,830 PointsHi Kathryn! If you notice the error you receive with your code, it gives you this hint. "You only need to call the prompt() method once, and only inside the loop. Hint: Declare the secret variable before the loop. "
First it tells you that you only need to call the prompt method once, and ONLY inside the loop. In your code you've called it twice, before the loop and inside. So you want to remove the prompt from outside the loop and place inside like so...
do {
secret = prompt("What is the secret password?");
} while ( secret !== "sesame" );
Finally it tells you declare the secret variable before the loop. When declaring a variable you don't have to instantly assign it a value, you can assign a value later on. In this case we want to declare the variable secret outside of the loop and assign the prompt to it within the loop.
var secret;
do {
secret = prompt("What is the secret password?");
} while ( secret !== "sesame" );
document.write("You know the secret password. Welcome.");
Hope this helps!
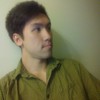
Kenney Hersch
8,167 PointsFor the challenge, it just wants you to call the prompt only in the do while loop.
Your code has you calling the prompt twice, once as you're declaring a variable for the prompt, then again when you start the do while loop.
In this case, declaring the variable secret to 0 (zero) and then having the prompt inside the do while loop ask for the password let's you finish the task.
var secret = 0;
do {
secret = prompt("What is the secret password?");
} while ( secret !== "sesame" );
document.write("You know the secret password. Welcome.");

rdaniels
27,258 PointsWhen the prompt appears, type in anything but "sesame".

Kathryn Notson
25,941 PointsKenney Hersch:
If I define var secret = 0, that's a number value & not a string value. Should I define it as var secret = "sesame"; instead?
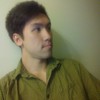
Kenney Hersch
8,167 PointsNo, the challenge wants you to just declare a variable secret so that var secret just 'exists' in your code, so that you can use it in your loop. I like to think of it as a 'placeholder' for that variable.
Once your loop starts, the user will be prompted with a question asking for a password, then it'll take that password and put it into secret, thus overriding the initial 'placeholder' we put in when declaring the variable secret.
Because when using the do while loop, that loop code will run at least once, before figuring out if it needs to run again because of the 'while' part of the code. And it would be redundant if we first asked a user for a password, THEN have to ask again when the do while loop runs.
Kenney Hersch
8,167 PointsKenney Hersch
8,167 PointsI checked with the challenge if the var secret; is accepted as a solution, and i swear it didn't for the sake of passing the challenge. Though I can't confirm it at the moment, since I'm having issues having any challenges passed and get prompted with a connection issue.
I just wanted to clarify and point that part out as opposed to my solution with setting the var secret = 0 solution.
Kristopher Van Sant
Courses Plus Student 18,830 PointsKristopher Van Sant
Courses Plus Student 18,830 PointsHmm that's strange, var secret; works for me. Also, var secret and var secret = 0 are essentially the same thing. When you declare a variable without assigning it a value it technically has the value "undefined" .
Kathryn Notson
25,941 PointsKathryn Notson
25,941 PointsKristopher Van Sant:
Yes, it finally worked! Thanks.
Kristopher Van Sant
Courses Plus Student 18,830 PointsKristopher Van Sant
Courses Plus Student 18,830 PointsAwesome! Glad we could help :)