Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial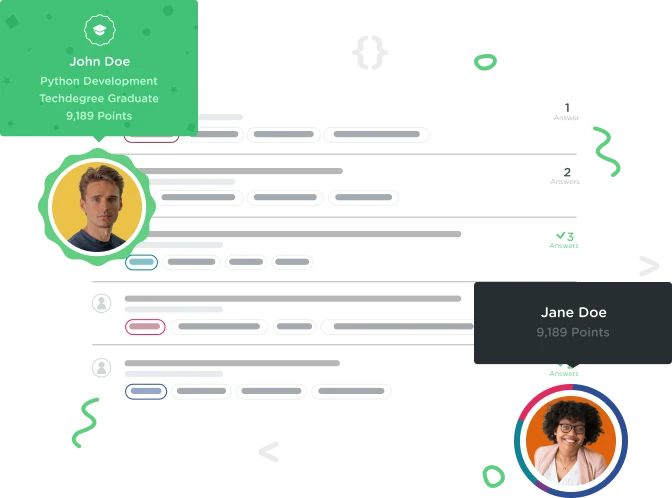

Josh C
Courses Plus Student 503 PointsJavascript: Nested Data exercise solution not working
Hi all
I'm making my way through the Javascript Nested Data and Additional Exploration video, and I cannot figure out why this solution will not work for me. What's strange is that the instructor give this answer in the video, but I cannot get it to work. He uses 2 instances of .map(), but I think the second time it's used, the data is still nested too far down.
If you're curious as to the URL of the video, it can be found here at around the 6:00 mark: https://teamtreehouse.com/library/nested-data-and-additional-exploration
Please review the data and my (not working) solution at the bottom, under the data.
Any thoughts? I would love to hear what the issue is, and how I can resolve it in my code. Thanks!
const users = [
{
name: 'Samir',
age: 27,
favoriteBooks:[
{title: 'The Iliad'},
{title: 'The Brothers Karamazov'}
]
},
{
name: 'Angela',
age: 33,
favoriteBooks:[
{title: 'Tenth of December'},
{title: 'Cloud Atlas'},
{title: 'One Hundred Years of Solitude'}
]
},
{
name: 'Beatrice',
age: 42,
favoriteBooks:[
{title: 'Candide'}
]
}
];
// Result: ['The Iliad', 'The Brothers Karamazov', 'Tenth of December', 'Cloud Atlas', 'One Hundred Years of Solitude', 'Candide'];
var books = users
.map(function(user) {
return user.favoriteBooks;
})
.map(function(book) {
return book.title;
})
.reduce(function(arr, titles) {
return [ ...arr, ...titles ];
}, []);
console.log(books);
1 Answer

andren
28,558 PointsThe issue is that your second map
call is being called on the wrong thing. In the video the second map
is called on the user.favoriteBooks
array within the first map
function, meaning that the second map
is nested within the first map
.
In your code you call the second map
on the result of the first map
, which results in an array of objects being passed to the second map instead of the object itself.
If you fix that issue like this:
var books = users
.map(function(user) {
return (user.favoriteBooks
.map(function(book) {
return book.title;
}));
})
.reduce(function(arr, titles) {
return [...arr, ...titles];
}, []);
Then your code will work.
By the way is there a specific reason why you choose to use traditional functions over arrow functions like the instructor used in the lecture? With code like this where there are a lot of single line functions being used and nested in each other, arrow functions can make the code a lot less verbose and simpler to look at.
Here is the above code rewritten with arrow functions:
var books = users
.map(user => user.favoriteBooks.map(book => book.title))
.reduce((arr, titles) => [...arr, ...titles], []);
Josh C
Courses Plus Student 503 PointsJosh C
Courses Plus Student 503 PointsThank you so much for that answer. I now understand.
I actually don't have a good reason why I'm using traditional functions over arrow functions. I'm prepping for a boot camp that favors regular functions, but I personally really enjoy using arrow functions - they seem much more clear and concise.