Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial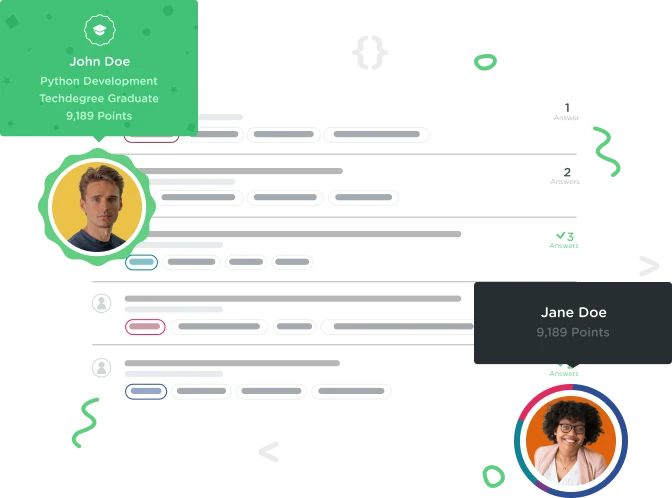
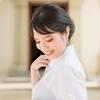
Pimwipha Cheaptumrong
10,977 Points(JavaScript Number) Random number challenge - Two Number Solution.
I wonder why we can't just use:
const randomNumber = Math.floor( Math.random() * highNumber ) + 1;
why it has to be :
const randomNumber = Math.floor( Math.random() * (highestNumber - lowestNumber + 1 ) + lowestNumber;
Btw I am not very good at Math. Please help. Thank you!
4 Answers

Cornelius Hager
5,659 PointsWithout using lowestNumber the number that is generated will always be between 1 and your highNumber.
Example
If you use these numbers for example:
lowestNumber = 10 highestNumber = 15
The code below will give you a random number between 1 and 15. This is not what you want. As the randomNumber could also be a 2 or 4. This number is lower than your provided lowestNumber of 10.
const randomNumber = Math.floor( Math.random() * highNumber ) + 1;
So you need to somehow tell your code from what lowest number it should start generating the random number. If you look at your range 10 - 15. You realise, I actually only need a range of 5 numbers: from 1 - 5, as the numbers from 1 - 10 are unnecessary for the calculation of the number between 10 and 15.
So, how do you calculate this cleverly?
As we just established you actually need the size of the range. 1 - 5 in the case above. How do you get that range? Simply by tacking the highestNumber and subtracting the lowestNumber. 15 - 10 = 5. Nice. That's the range we need.
Let's examine the correct code.
const randomNumber = Math.floor( Math.random() * (highestNumber - lowestNumber + 1 ) + lowestNumber;
The code runs, from the inner most parentheses to the outside.
1.
highestNumber - lowestNumber + 1
Here the range which is actually needed is calculated. See my example 15 - 10 = 5. The + 1 is added so the range starts from 1 and not from 0.
2.
Math.random() * (highestNumber - lowestNumber + 1 )
The range is multiplied with the random number generated by Math.random. (Remember the generated number is between 0-1 (e.i. 0,121213 or 0,99123123)
3.
Math.floor( Math.random() * (highestNumber - lowestNumber + 1 ))
The generated number for your range is being rounded down to a flat integer.
4.
randomNumber = Math.floor( Math.random() * (highestNumber - lowestNumber + 1 ) + lowestNumber;
Now the final magic is happening. Let's get back to our 10 - 15 example. We subtracted 10 from 15. We got 5. We got a random number between 1 and 5. Let's say 3. Now you only need to add back the 10 to the 3 and tada!! you have 13. A random number between 10 and 15.
Took me a while as well to wrap my head around it.

Zimri Leijen
11,835 PointsMath.random() gives a number between 0 and 1.
By giving a high number and a low number (and creatively multiplying) you can create a number in any range.

Chris Goyette
2,910 PointsThanks Cornelius. That link you sent me to w3schools was very helpful.
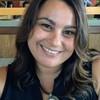
Angela Davis
Full Stack JavaScript Techdegree Student 3,110 PointsVery helpful explanation thank you. I had run my code as shown below and it was working for me, but your version and explanation works so much better and make logical sense.
let randomNum = Math.floor(Math.random() * highNum) +lowNum;
Chris Goyette
2,910 PointsChris Goyette
2,910 PointsBut what if we don't generate a 3 but a 0 instead?
Then the random number will be 10.
If we display the code in console.log() it would say something like "10 is a random number between 10 and 15."
Now that doesn't make much sense to me.
Cornelius Hager
5,659 PointsCornelius Hager
5,659 PointsGood point Chris, I didn't explicitly mention this in my comment as this is thoroughly explained in the videos before this exercise.
In my code that's what the +1 is for. As the Math.random() never returns a flat 1 the result can be 11, 12,13,14. Check the documentation here for more info: https://www.w3schools.com/js/js_random.asp
Abel Gechure
13,608 PointsAbel Gechure
13,608 PointsWow, this was very helpful. Thank you for breaking it down in chunks that can be understood well! Now I get it!!!! Thank you.
Adriaan van Wyk
8,948 PointsAdriaan van Wyk
8,948 PointsCornelius - this was a great explanation. Thanks!
Skyler Harris
7,670 PointsSkyler Harris
7,670 PointsAwesome. Great breakdown, this is what helped me finally understand this random number challenge solution.