Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial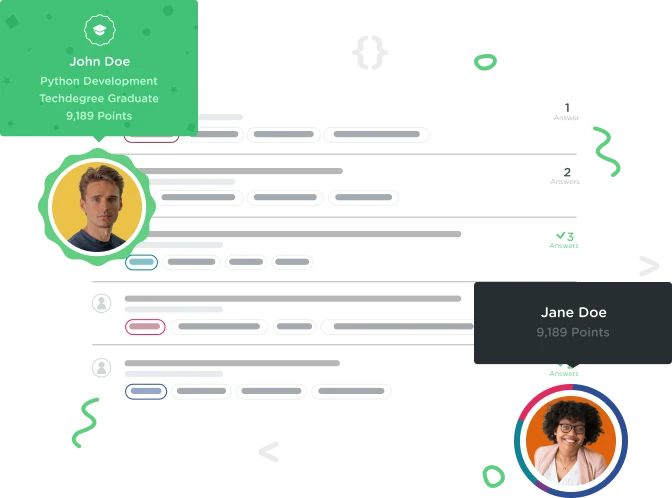
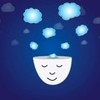
Douglas Watts
5,464 PointsJavascript Objects
This Challenge is way beyond me, does anyone understand and could you please explain:
var genericGreet = function(name, mood) {
name = name || "you";
mood = mood || "good";
return "Hello " + name + ", my name is " + this.name +
" and I am in a " + mood + " mood!";
}
var andrew = {
name: "Andrew"
}
var args1 = ["Michael", "awesome", ":)"];
var greeting = genericGreet;
thanks.
3 Answers
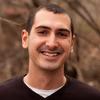
tihomirvelev
14,109 PointsWell...let me try to explain it with my broken English. :)
The answer is:
var greeting = genericGreet.apply(andrew, args1);
The basic task is to replace the variables name , mood and this.name in the var genericGreet in the first line of the code.
To do that you can use 2 methods - call()
or apply()
.
The only basic difference between the two methods is that with apply()
you can pass in an array of arguments as an argument but with call() you have to write manually every single argument.
Since in the challenge you have to use the array args1
, then you must use apply()
.
apply()
takes up two arguments - the first is the context and the second is the array.
The context is simply what will be replaced each time when there is this.name
or any other type of that. So if there is this.something
it will be replaced with the context.
So in the challenge you are passing the object andrew as a context. That way, in the function of the top of the code, this.name
will be replaced with andrew.name
. And since andrew.name = "Andrew"
, his name will be displayed in that place.
The second argumet is the array args1
. The first element is "Michael", the second is "awesome" and the third is ":)".
You can see that the top page function takes up two arguments - name and mood.
Therefore name = Michael and mood = awesome. The third element in the array is just to fool you. It's not gonna actually be used in the function because the function takes only two arguments.
That's the basic explanation and I hope it helps. At first it might be confusing but watch Jim's video a few more times and you will understand it: http://teamtreehouse.com/library/javascript-foundations/objects/call-and-apply
Good luck!

Derick Moncado
23,259 PointsThanks man! Really helpful!
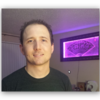
Brian van Vlymen
12,637 Pointswow it was excellent explain! I would like to take other challenge using call to see the diff between apply and call method.
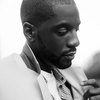
Michael Hicks
24,124 PointsFantastic explanation! Thank you tihomirvelev.
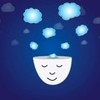
Douglas Watts
5,464 PointsSorry, i forgot to include the challenge text, so i will start over...Could someone please help with this Challenge:
Set the 'greeting' variable, on line 29, by using the 'genericGreet' function in the context of 'andrew' with the array of arguments, 'args1'.
var genericGreet = function(name, mood) {
name = name || "you";
mood = mood || "good";
return "Hello " + name + ", my name is " + this.name +
" and I am in a " + mood + " mood!";
}
var andrew = {
name: "Andrew"
}
var args1 = ["Michael", "awesome", ":)"];
var greeting = genericGreet;
thanks.
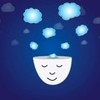
Douglas Watts
5,464 PointsThanks, your English was perfect and i will go over the video a couple more times.
tihomirvelev
14,109 Pointstihomirvelev
14,109 PointsHello,
If you type the text/question of the challenge it will be much easier to help. :)