Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial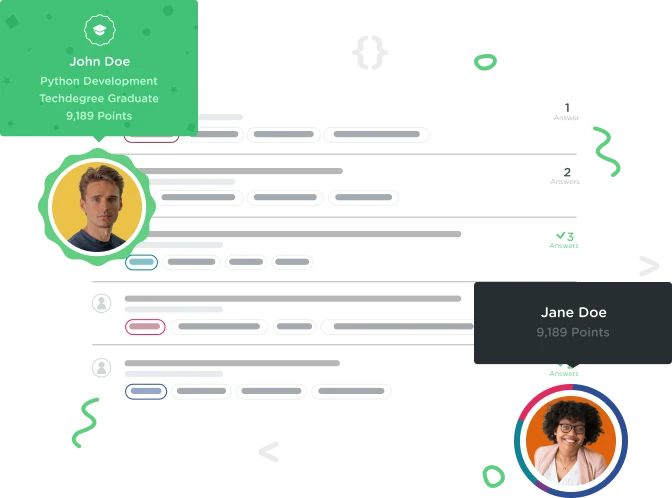
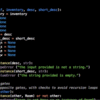
Hammad Ahmed
Full Stack JavaScript Techdegree Student 11,910 PointsJavaScript Objects
I'm getting confused about JavaScript Objects. In the course by Dave McFarland, he explained objects as group of variables. But in a book I read the same thing was mentioned but It was called Associative Arrays. And there is a lot of other explanation for JS Objects.
2 Answers
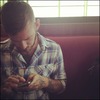
Erik McClintock
45,783 PointsHammad,
I recently answered another post on the forums here looking for a little more information on objects, properties, and methods, which you can view here. Some of the information in there may help to visualize what objects are.
For clarity, though, Dave's explanation in this course is (obviously) correct, as far as breaking down what objects are in simple terms, if a little confusing. But if you take a look at an example object, you can start to see how it makes a little more sense:
var person = {
}
Here, we have a JavaScript object called "person". This object in and of itself does nothing for us in its current state. It is merely a blue print for the concept of a "person". Once we will this object with a collection of variables, however, it becomes more important and useful. Let's say we want to create a specific person, named Alicia. We can do this easily in our object:
var person = {
name: "Alicia"
}
Great, now our "person" object is of some use to us! If at some point in our code, we want to know the name of our person, we can refer to their property called "name", like so:
console.log(person.name);
//or
console.log(person['name']);
You may have noticed the square brackets there - that is another way you can refer to properties of an object, similar to how you would refer to an index or a key in an array. Multidimensional arrays are just arrays that are filled with other arrays, but the thing to realize here is: arrays are actually a type of object in JavaScript! If you run "typeof" on an array, it will return "Object". Just like objects, arrays are collections of values. The main difference here is that JavaScript doesn't support what are called "associative arrays", where you can store key and value pairs...at least, it doesn't support them as an array. Arrays can just hold a collection of values, while Objects can hold keys and values that you can look up and refer to. JavaScript objects are effectively associative arrays from other languages. JavaScript objects use named indexes, while JavaScript arrays use numeric indexes. Thus, you want to use the right tool for the right project/instance, and if you need to store values inside of individually named keys, an object is the way to go.
So, let's say we need more information about our person, because just knowing their name doesn't do much for us. We want to know their age, their occupation, and maybe we even want them to be able to say hello and tell us some information about themselves!
var person = {
name: "Alicia",
age: 25,
occupation: "Web Designer",
introduceYourself: function() {
console.log("Hello, my name is " + this.name + ", I am " + this.age + " years old, and I am a " + this.occupation + ". Nice to meet you!");
}
}
Now we have a more useful object, because it holds more properties and even a method! We can now do more interesting things with it and find more information using it, as opposed to before. Additionally, we can make better, more efficient use of this object than if we simply had a) a bunch of random variables, or b) an array with only values. Now we can look up some specific information from our person object, or we could even loop over and print out everything that's inside if we wanted! Now, this person object could be useful to us, but we also have the option of making what's called a "constructor", which would basically create a blue print of this blue print for us, at which point, we could reuse that constructor to create as many different people as we wanted! This might look a bit confusing, but just take it in to know that it exists, and that it is something to look forward to with the power of objects:
// this is our object constructor, for an object type that we're calling "Person"
function Person(name, age, occupation) {
this.name = name;
this.age = age;
this.occupation = occupation;
}
// this adds the method "introduceYourself" to any Person objects that we create using our constructor
Person.prototype.introduceYourself = function() {
return "Hello, my name is " + this.name + ", I am " + this.age + " years old, and I am a " + this.occupation + ". Nice to meet you!";
}
// this is instantiating a new variable called "erik", which we are saying is a new Person object, and we are passing in the correct/necessary parameters to give this object all the information that it needs to exist and be useful according to our blue print!
var erik = new Person("Erik", 27, "Web Developer");
// thus, the "erik" variable is now an object that looks like this:
var erik = {
name: "Erik",
age: 27,
occupation: "Web Developer",
introduceYourself: function() {
return "Hello, my name is " + this.name + ", I am " + this.age + " years old, and I am a " + this.occupation + ". Nice to meet you!";
}
}
// all because we used that constructor! We could go on to make as many different Person objects as we wanted
// because "erik" is a Person object, and we gave all Person objects access to the "introduceYourself" method, now that we've instantiated "erik" AS a Person object, we can call that method on it!
console.log(erik.introduceYourself());
// erik.introductYourself would then return the string found in that method, and would fill in the missing spots with information from the erik object
Through all of that, you can hopefully see a little more clearly what Dave meant when he said that objects were just collections of variables, because they are! Without those variables (think of "name: 'Erik'" as being any other JavaScript variable outside of the object, like "var name = 'Erik'") inside those objects, they would be empty shells.
It may all be a bit confusing now, but trust me, with time and practice, it will all start to come together. Objects and arrays were the biggest hurdle for me when learning object oriented programming, but after using them time and again, the picture is getting much more clear.
Hopefully you've found some of this information useful, but the best course of action is to just keep on keeping on with your studies and practice, and it will come to you! Try and learn from a variety of other resources as you've been doing, and see things from different perspectives. You may find that one way of thinking about these abstract terms makes more sense to you than another, and by finding that one way of looking at it, everything might click.
Happy coding!
Erik
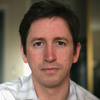
jamescool
44,592 PointsI'm not sure what book you were reading, but it's easier for me to conceive of a JavaScript Object (as opposed to a multidimensional array) as being a group of variables because it's a collection of properties and values. Despite the fact that variables are not themselves referred to as "properties", they would be if they were features of an Object. An array--or a multidimensional array, for that matter--has values which are tied not to properties, but to indices. In this way, Objects and multidimensional arrays are different.
Could you possibly direct us to the passage in the book you mention?
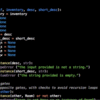
Hammad Ahmed
Full Stack JavaScript Techdegree Student 11,910 PointsI read Learning PHP, MySQL and JavaScript by Robin Nixon.
Hammad Ahmed
Full Stack JavaScript Techdegree Student 11,910 PointsHammad Ahmed
Full Stack JavaScript Techdegree Student 11,910 PointsThanks Erik for your great explanation, I helped a lot and Happy Coding!