Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial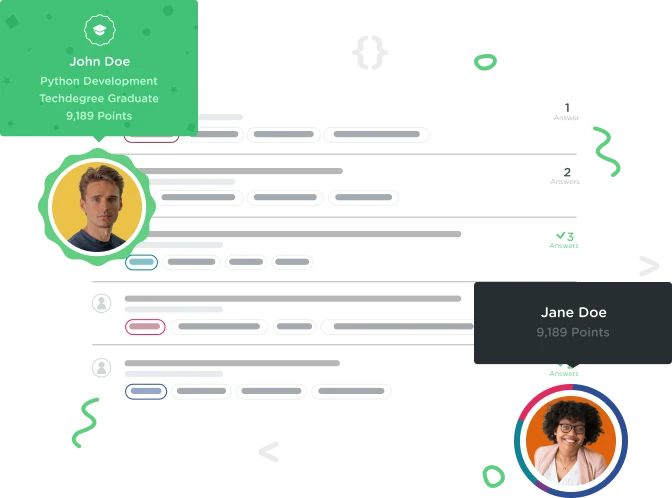

steven clark
5,009 PointsJavascript "OR" Operator
Hello!
I just finished the javascript "functions" code challenge which asks the following:
"Create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in."
My first attempt was this:
function arrayCounter (array) {
if(typeof array === "undefined" || "string" || "number") {
return 0;
}
else {
return array.length;
}
}
I am wondering why the above code did not work? If the typeof array is === to undefined, or , string, or number, why would this code not run?
I eventually solved it using the following code below, however I am still trying to figure out how the above code wouldn't run properly.
function arrayCounter (array) {
if(typeof array === "undefined" || typeof array === "string" || typeof array === "number") {
return 0;
}
else {
return array.length;
}
}
2 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsThis part of your code works partially, but it doesn't work as you expect it to:
if(typeof array === "undefined" || "string" || "number") {
return 0;
}
To understand what's happening here, you must understand how the logical or operator in JavaScript works. Take this example:
firstExpression || secondExpression;
This piece of code will return firstExpression
if it can be coerced into boolean true
. If not, it will return secondExpression
. It doesn't matter if the second expression is true
or false
, it still gets returned. It's the if
statement that decides which code is run based on the truthiness of the returned value, or rather the returned value being compared to something else through the equality operators (==
, ===
).
If you chain several expressions with logical or operators, as soon as you hit the first one that can be coerced into boolean true
, that one will get returned (and other expressions are not even evaluated anymore — this is called short-circuit evaluation). If none of them can get coerced to true
, the last expression will be returned.
In the case of your original if
statement, it's basically equivalent to this:
if ( (typeof array === "undefined") || "string" || "number") {
return 0;
}
If the type of the array
variable is "undefined"
, the operators are short-circuited, and the code inside the if
statement is run. Otherwise, the operators move on to the next expression, and that expression is "string"
. All non-empty strings are truthy values, so the operators are short-circuited there, and the code inside the if
statement gets executed even if it shouldn't be running, because it's not evaluating the truthiness of the typeof
comparison, but rather the truthiness of a string.
That's why you have to write the code like this:
if (typeof array === "undefined" || typeof array === "string" || typeof array === "number") {
return 0;
}
Now each of the typeof
expressions may or may not return a boolean true
, and those expressions are then evaluated through the logical or operators. In case one of them is true
, the code in the if
statement is run. If all of them are false, the last falsy value is returned and the code in the if
statement is ignored.

Aaron Graham
18,033 PointsEach 'OR' statement is evaluated for truthiness separately. In your first attempt, "string" and "number" don't express any kind of condition that needs to be met. In other words, the 'ORs' don't chain, if that makes sense. Each 'OR' stands as its own statement.