Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial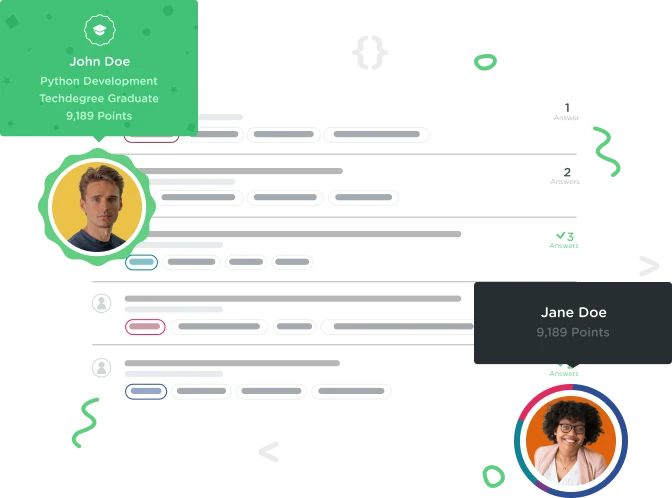

RODRIGO MARTINEZ
5,912 PointsJavascript Project - Simple quiz with radio buttons
Hi everyone. I'm starting to build small projects try to apply what i'm learning in the Full Stack Javascript track. I'm doing the common excercise of buildinz a quiz. The Quiz works. But after i press the button to submit answers, I'd like to get the selected answer in color green if it's correct and red if it is wrong. I applied the style.color change inside of the if statement of the function but it's not working. Could someone please help? Thanks.
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<link rel="stylesheet" href="js quiz.css">
<title>JS Quiz</title>
</head>
<body>
<h1 id="main-h">Desafío: Cuestionario de fútbol</h1>
<h2 id="questions-h">Preguntas</h2>
<p class="questions" id="question1">
1- Quien es el único jugador de futbol argentino que tiene una serie en Netflix?</p>
<input type="radio" name="answer1" value="wrong">Diego Maradona
<input type="radio" name="answer1" value="correct">Carlos Tevez
<input type="radio" name="answer1" value="wrong">Lionel Messi
<hr>
<p class="questions" id="question2">
2- Quien es el máximo goleador histórico de la selección brasileña?</p>
<input type="radio" name="answer2" value="wrong">Romario
<input type="radio" name="answer2" value="wrong">Ronaldo
<input type="radio" name="answer2" value="correct">Pelé
<hr>
<p class="questions" id="question3">
3- De los siguientes jugadores. Quien NO jugó para ambos equipos, Real Madrid y Barcelona</p>
<input type="radio" name="answer3" value="wrong">Luis Enrique
<input type="radio" name="answer3" value="wrong">Figo
<input type="radio" name="answer3" value="correct">Ronaldinho
<hr>
<p class="questions" id="question4">
4- Que jugador de la siguiente lista jugó para Internazionale de Milano y para A.C. Milano</p>
<input type="radio" name="answer4" value="wrong">Cristiano Ronaldo
<input type="radio" name="answer4" value="correct">Zlatan Ibrahimovic
<input type="radio" name="answer4" value="wrong">Samuel Etoo
<hr>
<p class="questions" id="question5">
5- Cual es el quipo que ha ganado más Champions League en la historia?</p>
<input type="radio" name="answer5" value="correct">Real Madrid
<input type="radio" name="answer5" value="wrong">F.C. Barcelona
<input type="radio" name="answer5" value="wrong">A.C. Milano
<br>
<br>
<button class="submit" onclick="return getResults();" type="submit">
Comprueba tus respuestas</button>
<h2 id="results-h">Resultados</h2>
<p id="results"></p>
<script type="text/javascript" src="js quiz.js">
</script>
</body>
</html>
function getResults() {
//CORRECT ANSWERS
var amountCorrect = 0;
// LOOP FOR GOING THROUGH ALL QUESTIONS
for(var i = 1; i <= 5; i++) {
var radiosName = document.getElementsByName('answer'+i);
//LOOP FOR CHECKING ANSWERS INSIDE EACH RADIO
for(var j = 0; j < radiosName.length; j++) {
var radiosValue = radiosName[j];
if(radiosValue.value == "correct" && radiosValue.checked) {
amountCorrect++;
radiosValue.style.color = "green";
}
}
}
document.getElementById('results').innerHTML =
"Respuestas correctas " + amountCorrect;
}
2 Answers
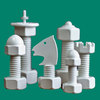
Steven Parker
230,274 PointsFirst, it's not the button you want to color (radio buttons don't respond to styling) but the text associated with it. This can be done by selecting the button's next sibling except that at the moment those are text nodes which don't have "style" properties. So you'd probably want to first put the text in elements (such as "span"s):
<input type="radio" name="answer5" value="correct"><span>Real Madrid</span>
<input type="radio" name="answer5" value="wrong"><span>F.C. Barcelona</span>
<input type="radio" name="answer5" value="wrong"><span>A.C. Milano</span>
Then, you can have the code color the next sibling. And you'll want a few extra cases to handle red for not correct and back to default when not checked:
//LOOP FOR CHECKING ANSWERS INSIDE EACH RADIO
for (var j = 0; j < radiosName.length; j++) {
var radiosValue = radiosName[j];
if (radiosValue.checked) {
if (radiosValue.value == "correct") {
amountCorrect++;
radiosValue.nextSibling.style.color = "green";
} else {
radiosValue.nextSibling.style.color = "red";
}
} else {
radiosValue.nextSibling.style.color = ""; // back to default
}
}

RODRIGO MARTINEZ
5,912 PointsHello Steven. Got it now. I didn't realize i had left the other code there. Thanks a lot!!!
RODRIGO MARTINEZ
5,912 PointsRODRIGO MARTINEZ
5,912 PointsHello Steven. Thanks a lot for taking thetime to help! I've put the text of every radio input in span in the HTML file as you said. And also copied the if statements provided into my function. But for some reason the color change it's not working anyways.
Steven Parker
230,274 PointsSteven Parker
230,274 PointsThe new test code was intended to replace the previous test code, not to be added after it.
And be sure there is nothing between the <input> and <span> elements in the HTML, not even a new line. Otherwise, you might need "nextElementSibling" instead of "nextSibling".