Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial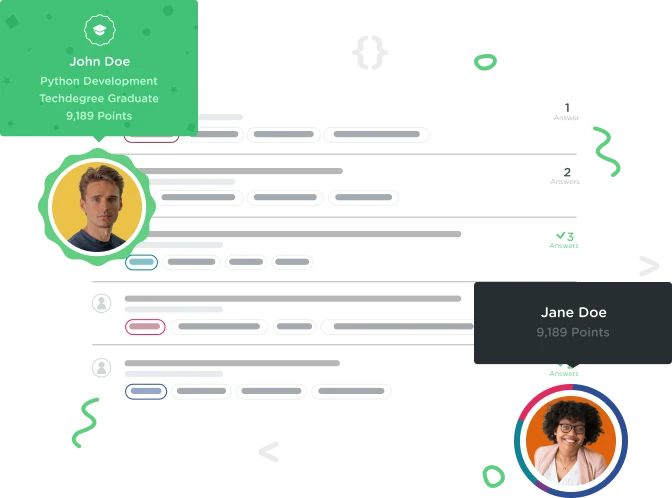

Arnaud Ardans II
5,089 PointsJavascript Question
So a group of us in a Javascript Class are working on this assignment, and we are all trying to work with each other at this point, and several of us have talked with coworkers and other friends that know Javascript, and between the 10 or so of us we haven't come up with a solution. Between all of us we can't get past step 6, and a couple people are currently stuck at steps 4 and 5. I know there may be someone out there that can help us out with this and maybe better explain what we hadn't gotten in the class. As a side note I would love to say that the instructor didn't explain it very well, but in the end she just didn't have the time to because we have a student in our class that asks 3-4 irrelevant questions every couple of minutes that just wastes the classes time and there isn't really a way for us to get him to stop because of ADA related issues. (Not the Dental Association ;-P) I have nothing against the student, but he is wasting our time with all of these irrelevant questions and now we are stumped. :-( And don't think I'm heartless or anything because I've got my own cognitive issues related to receiving high dose chemotherapy and also in the class with ADA assistance options.
But back to the assignment. Anyone got any ideas?
This is the base file given to us for the assignment.
<!DOCTYPE html>
<html>
<head>
<title>Example 4</title>
<!-- INSTRUCTIONS:
1. In the head, create 2 empty arrays.
One should be for past appointments, one should be for future appointments.
2. After the arrays are created, write a new function that takes a single information parameter.
3. Inside the function, split the information parameter by commas and save the result to a new variable.
4. Create a second variable that makes a new object.
Inside the object, assign the first element of the array to the firstName property.
Assign the second element of the array to the lastName property.
Assign the third element of the array to the email property.
Use the fourth element of the array to create a new Date and assign it to the time property.
5. After the object is created, use a regular expression test to see if the firstName property of the
object contains only letters and no spaces.
If it does not, alert the user that the first name is invalid.
If it does, use a second regular expression test to see if the lastName property of the object
contains only letters and no spaces.
If it does not, alert the user that the last name is invalid.
If it does, use a third regular expression test to see if the email property of the object is a valid
email. For the purposes of this exercise, a valid email has an @ in it and a . somewhere after that.
If it does not, alert the user that the email is invalid.
If it does, check to see if the time property of the object is set to before the current date and time.
If it is, add the object to the the past appointments array.
If it is not, add the object to the future appointments array.
6. Call the function using your name, email, and a date in the following format:
"firstname, lastname, email, month date year time"
7. Call the function 2 or 3 more times using other names, emails, dates.
Try to make sure at least one date is before and one date is after the assignment's due date.
8. Alter the ListAppointments function to take a parameter.
Use that parameter in place of the appointments variable inside the function.
9. After the Past Appointments header in the body, call the ListAppointments function on the past
appointments array.
10. After the Future Appointments header in the body, call the ListAppointments function on the future
appointments array.
-->
<script>
function printAppointment(appointment) {
document.write("You have an appointment with: <a href='mailto:" +
appointment.email +"'>" +
appointment.firstName + " " +
appointment.lastName + "</a> at " +
appointment.time + "<br>");
}
function listAppointments() {
for(var i = 0; i < appointments.length;i++) {
printAppointment(appointments[i]);
}
}
</script>
</head>
<body>
<h1>Past Appointments</h1>
<h1>Upcoming Appointments</h1>
</body>
</html>
2 Answers
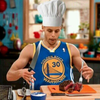
0yzh
17,276 PointsHey Arnaud,
I believe this should do the trick. Let me know if you still need help, happy coding!
<html>
<head>
<title>Example 4</title>
<script>
// Task 1
var pastAppointments = [];
var futureAppointments = [];
// Tasks 2 through 5
function addAppointment(a) {
var splitArr = a.split(', ');
var obj = {
firstName: splitArr[0],
lastName: splitArr[1],
email: splitArr[2],
time: new Date(splitArr[3])
};
var nameCheck = /^[a-zA-Z_\-]+$/;
var emailCheck = /^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?(?:\.[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?)*$/;
if ( nameCheck.test(obj.firstName) !== true ) {
alert('Invalid first name! Please try again');
} else if ( nameCheck.test(obj.lastName) !== true ) {
alert('Invalid last name! Please try again');
} else if ( emailCheck.test(obj.email) !== true ) {
alert('Invalid email! Please try again');
} else if ( obj.time < new Date() !== true ) {
futureAppointments.push(obj);
} else {
pastAppointments.push(obj);
}
}
// Tasks 6 and 7
addAppointment('Joe, Doe, joedoe@gmail.com, 12 16 2015 12:24');
addAppointment('John, Doe, johndoe@gmail.com, 02 16 2016 12:24');
addAppointment('Jane, Doe, janedoe@gmail.com, 04 16 2016 12:24');
function printAppointment(appointment) {
document.write("You have an appointment with: <a href='mailto:" +
appointment.email +"'>" +
appointment.firstName + " " +
appointment.lastName + "</a> at " +
appointment.time + "<br>");
}
// Task 8
function listAppointments(arr) {
for(var i = 0; i < arr.length;i++) {
printAppointment(arr[i]);
}
}
</script>
</head>
<body>
<h1>Past Appointments</h1>
<script>
// Task 9
listAppointments(pastAppointments);
</script>
<h1>Upcoming Appointments</h1>
<script>
// Task 10
listAppointments(futureAppointments);
</script>
</body>
</html>

Arnaud Ardans II
5,089 PointsHey thanks Huy Bui
I think your code is close to what the instructor wanted, but she admitted to writing the instructions pretty late and forgot to proof read it for clarity. Plus she had forgot to instruct and show us a few of the things that would have helped get this code working. So she ended up rewriting the project, and added a few extra steps so we can at least get full credit for this assignment if we complete the new version. At least this one isn't so bad.
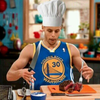
0yzh
17,276 PointsNo problem, The code above does exactly what the instructor wanted originally in the base file she gave you. But if you are saying she changed the instructions for this assignment then it probably won't match what the new instructions are. Good luck!