Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial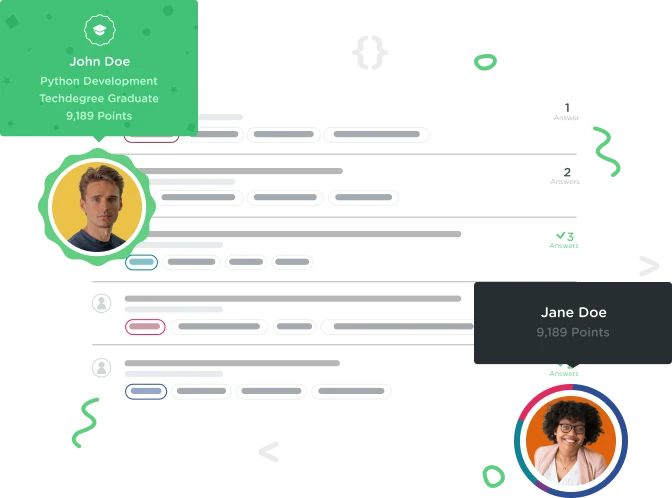

Josiah Bennett
2,271 PointsJavaScript Quiz Challenge
/*
<title>The Ultimate State Capital Quiz</title>
This application quizzes players on their state capital knowledge.
The player is asked a set of 5 questions and are prompted for an answer.
When an answer is given it tests the response to see if it is correct.
The application then lets the player know whether or not they answered correctly.
After the game is over the player's score is tabulated and it determines how they rank.
Author: Josiah Bennett
Website: www.josiahbennett.com
Date: 6/2/2015
*/
//creates variable for counter and sets it to zero to start.
var quizScore = 0;
//Creates answer bank to test responses to.
var questionOneAnswer = 'HARRISBURG';
var questionTwoAnswer = 'COLUMBUS';
var questionThreeAnswer = 'ALBANY';
var questionFourAnswer = 'PROVIDENCE';
var questionFiveAnswer = 'BOSTON';
//Creates variable for holding what the person guesses as the answer.
//Also changes their guess to uppercase to avoid any capitalization errors.
//Tests the guess against the answer and increases score if they get it right.
var questionOneGuess = prompt('Question 1: What is the capital of Pennsylvania?');
if (questionOneGuess.toUpperCase() === questionOneAnswer) {
quizScore = quizScore + 1;
alert('That is correct!');
} else {
alert('Sorry that is incorrect.');
}
var questionTwoGuess = prompt('Question 2: What is the capital of Ohio?');
if (questionTwoGuess.toUpperCase() === questionTwoAnswer) {
quizScore = quizScore + 1;
alert('That is correct!');
} else {
alert('Sorry that is incorrect.');
}
var questionThreeGuess = prompt('Question 3: What is the capital of New York?');
if (questionThreeGuess.toUpperCase() === questionThreeAnswer) {
quizScore= quizScore + 1;
alert('That is correct!');
} else {
alert('Sorry that is incorrect.');
}
var questionFourGuess = prompt('Question 4: What is the capital of Rhode Island?');
if (questionFourGuess.toUpperCase() === questionFourAnswer) {
quizScore = quizScore + 1;
alert('That is correct!');
} else {
alert('Sorry that is incorrect.');
}
var questionFiveGuess = prompt('Question 5: What is the capital of Massachusetts?');
if (questionFiveGuess.toUpperCase() === questionFiveAnswer) {
quizScore = quizScore + 1;
alert('That is correct!');
} else {
alert('Sorry that is incorrect.');
}
//Determines the player's score by testing how many they got correct.
if (quizScore === 5) {
document.write('<p>You win the gold crown with a score of ' + quizScore + ' correct answers.</p>');
} else if (quizScore === 4 || quizScore === 3) {
document.write('<p>You win the silver crown with a score of ' + quizScore + ' correct answers.</p>');
} else if (quizScore === 2 || quizScore === 1) {
document.write('<p>You win the bronze crown with a score of ' + quizScore + ' correct answers.</p>');
} else {
document.write("<p>You haven't won any crown. You answered " + quizScore + " questions correctly.</p>");
}
1 Answer
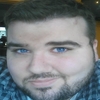
Marcus Parsons
15,719 PointsHey Josiah,
Good job on completing the challenge! An additional step to do after you've learned about arrays and loops is to convert this challenge in to a quiz game that uses less code than this challenge but allows you to add in as many questions/answers as you wish without adding in any additional if/else statements or prompts. It's a fun one to start work on and will increase your expertise with JavaScript. =]
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsHere's a codepen of a version I worked on for someone else to convert there challenge into a DRY solution that accepts more than one answer. http://codepen.io/anon/pen/WvppZX?editors=001