Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial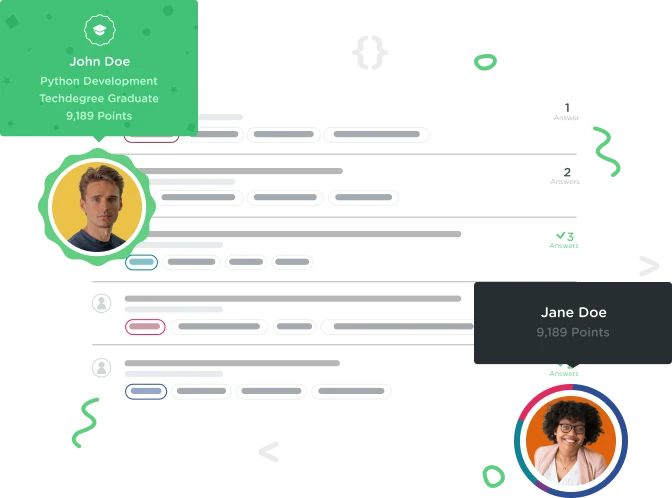
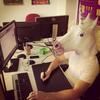
Nir Benita
Courses Plus Student 3,905 PointsJavascript recursion
Hey guys,
I am having a bit of trouble wrapping my head around Recursion in JS.
Could anyone point me to a good resource?
Thanks!
4 Answers

Tim Woodbury
Courses Plus Student 1,534 PointsThere's a section on this in Douglass Crockford's "Javascript: The Good Parts" - recommended reading for all JS developers in my opinion. You might also find this post useful: http://javascript.about.com/library/blrecursive.htm
In practical terms, recursion is the process of breaking an algorithm into a set of trivial operations and allowing the function call stack to substitute for a similar data structure which would otherwise be required by the iterative solution.
Clear as mud?

James Barnett
39,199 Points
Simon Winkler
2,477 Points…

Simon Winkler
2,477 Pointsi think the basic structure of a recursive function call is not that complicated
var x = 0;
function recursiveCall ( ) {
if ( x < 5 ) {
x += 1;
console.log(x);
recursiveCall();
} else {
return;
}
}
recursiveCall();
the key is that a recursive function calls itself, as long as a certain condition is true.
http://jsfiddle.net/simonwinkler/WNXaL/
i am a beginner, so don't rely on this example too much, but you can see how a i count up to five, using a recursive function call.