Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial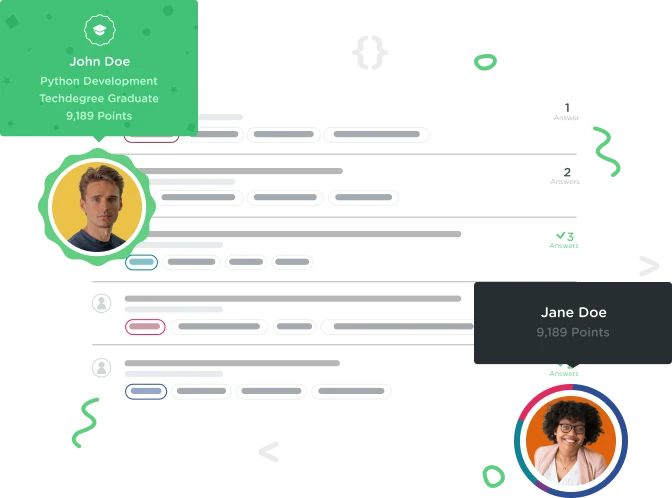

rymatech
4,671 PointsJavaScript: Refactoring a for loop to higher order function?
const numbers = document.getElementsByClassName("numbers");
const styleNumbers = () => {
for (let i = 0; i < numbers.length; i++) {
numbers[i].addEventListener("mouseover", () => {
numbers[i].style.backgroundColor = "#6a6a6a";
});
numbers[i].addEventListener("mouseout", () => {
numbers[i].style.backgroundColor = "#505050";
});
}
};
My attempt below. I've tried using both for each and map but having no success.
const styleNumbers = () => {
numbers.forEach(number => {
console.log(number);
number.addEventListener("mouseover", () => {
number.style.backgroundColor = "#6a6a6a";
});
number.addEventListener("mouseout", () => {
number.style.backgroundColor = "#505050";
});
});
};
1 Answer
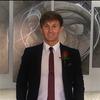
Liam Clarke
19,938 PointsHi Ryan
Your almost there, the problem is foreach loops through an array and "numbers" is not an array, it is a HTMLCollection so the loop is looping through a returned node list (an array object) rather than an array.
Couple ways to fix this:
Solution 1 - Array.from
Array.from() method creates a new, shallow-copied Array instance from an array-like or iterable object.
const styleNumbers = () => {
Array.from(numbers).forEach((number) => {
console.log(number);
number.addEventListener("mouseover", () => {
number.style.backgroundColor = "#6a6a6a";
});
number.addEventListener("mouseout", () => {
number.style.backgroundColor = "#505050";
});
});
};
Solution 2 - spread syntax
spread syntax allows an iterable such as an array expression or string to be expanded in places where zero or more arguments (for function calls) or elements (for array literals) are expected, or an object expression to be expanded in places where zero or more key-value pairs (for object literals) are expected.
const styleNumbers = () => {
[...numbers].forEach((number) => {
console.log(number);
number.addEventListener("mouseover", () => {
number.style.backgroundColor = "#6a6a6a";
});
number.addEventListener("mouseout", () => {
number.style.backgroundColor = "#505050";
});
});
};
Hope this makes sense
Good Luck!