Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial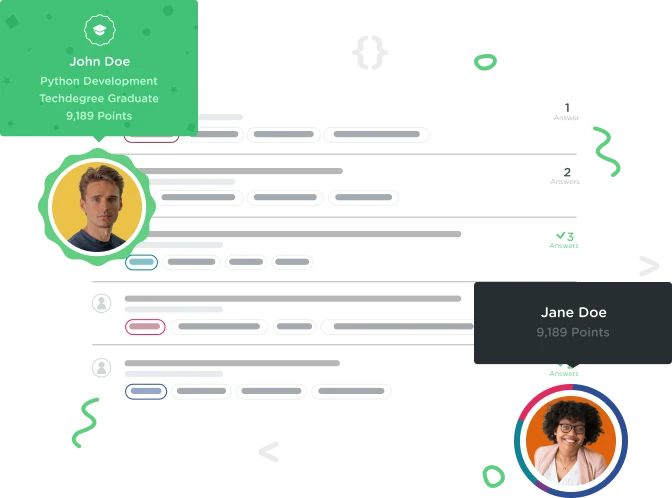
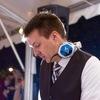
Darrell Osborne
23,146 PointsJavaScript .sort() Method - How does the comparison actually work?
I understand what the .sort() method does, and how to implement it. I also understand what the comparison function does when used with it. But I would like to better understand the steps that the comparison function goes through.
For example let's say we have an array with the values of [6, 10, 4, 12, 3], and I run .sort(function(a, b){return a-b}). As I understand it "a" will initially be assigned the first value of 6, and "b" the second value of 10. And then after it compares "a" to "b", it stays the same. The it reassigns "b" to value of 4, and compares again.
My question is: when "b" is smaller than "a" does it then reorder all of the index numbers staring with 4 being [0]? And then reorder again with 3 and so forth as it cycles through the array?
Question 2: after it has compared 6 to all other array values, does "a" then get reassigned to 10 and the same process continues?
1 Answer

Jason Anello
Courses Plus Student 94,610 PointsHi Darrell,
Great question!
Your question is really about implementation details. What gets passed in for "a" and "b" is going to depend on which sorting algorithm is used by the sort method. The ECMAScript specification doesn't define any particular sorting algorithm that should be used. The developers of each browser get to decide which algorithms to use.
I don't think an exact answer can really be given here but I can try walking you through a particular sorting algorithm so that you can better understand what gets passed in for "a" and "b".
Here's a list of various sorting algorithms if you want to dig deeper into this. https://en.wikipedia.org/wiki/Sorting_algorithm
Here's the main page for bubble sort which I'll use as the example. https://en.wikipedia.org/wiki/Bubble_sort
It's generally considered an inefficient sort and probably wouldn't be used in any browser but it's easier to understand and useful for learning. It gets its name from the fact that the highest element will "bubble up" to the top after the first pass. Then the next highest element and so on. It will always compare adjacent elements and check if they are in the right order, swapping if necessary. That page has an animation which can help you visualize it. You'll see that after the first pass through the list, the 8 has bubbled up to the top.
I'll use your array as an example and go through the first pass only to keep this from getting too long. It's the same idea for the second pass and so on.
At the start:
[6, 10, 4, 12, 3]
The sort method would start by passing in 6 and 10 to the compare function and it would return -4 which lets the sort method know that 6 should come before 10. No swap takes place since they are already in the correct order with respect to each other.
Then the next 2 adjacent elements, 10 and 4, will be passed to the compare function. This time the compare function will return 6, a positive value, and the sort method knows they are in the wrong order and will swap them.
[6, 4, 10, 12, 3]
Now the 3rd and 4th elements will be compared, the 10 and 12. No swap takes place. Then the 12 and 3 would be passed to the compare function and a swap would take place. One complete pass has been made at this point.
After the first pass through the array, it looks like this:
[6, 4, 10, 3, 12]
As you can see, the 12 "bubbled up" to the top and is now in the correct position. If you trace through a 2nd pass yourself, you'll find that the 10 bubbles up to the second highest spot. It will take several more passes to fully sort this array. Hence the inefficiency of bubble sort.
Hopefully this gives you a better understanding of what gets passed in for "a" and "b" and how the sorting could happen. Keep in mind that this example would be different if a different sorting algorithm had been chosen.
Let me know if anything is still unclear.
Darrell Osborne
23,146 PointsDarrell Osborne
23,146 PointsThis is exactly what I was looking for!
I started to realize after posting the question that the browser probably has it's own method of how to implement the sort, but this gives a much clearer understanding of the steps it goes most likely goes through. MDN lists the sort as a "comparison", if a function isn't otherwise passed through.
Thanks, Jason!