Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial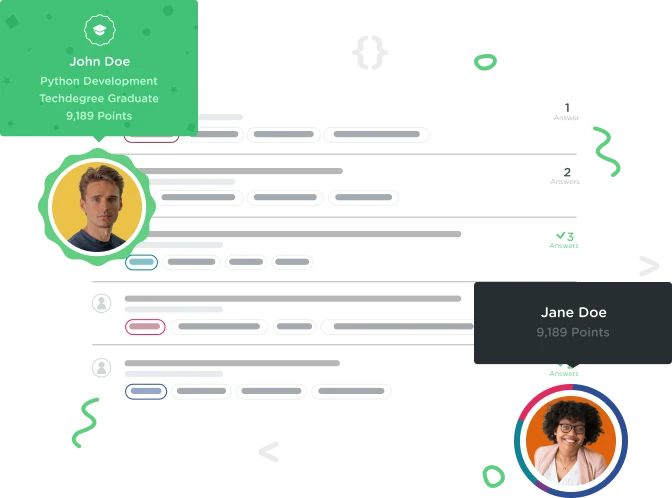

Erik L
Full Stack JavaScript Techdegree Graduate 19,470 PointsJavascript techdegree 1st Project
hello I am currently working on the first javascript project, "random quote generator", I have a few questions:
-How can I make the background color change to any random color after the user clicks on the button displayed on the page each the user clicks?
-Also how can I make it so that the webpage automatically changes quotes after a certain amount of times has passed , like every 3 seconds.
I did some research around the internet and I came across the (set interval) method, I tried to use it on the html file, however I am not entirely sure I used it correctly, could someone point me in the right direction on how to approach this challenge? Below is my code for html and javascript:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Random Quotes</title>
<link href='https://fonts.googleapis.com/css?family=Playfair+Display:400,400italic,700,700italic' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/normalize.css">
<link rel="stylesheet" href="css/styles.css">
</head>
<body>
<div class="container">
<div id="quote-box">
<p class="quote">Every great developer you know got there by solving problems they were unqualified to solve until they actually did it.</p>
<p class="source">Patrick McKenzie<span class="citation">Twitter</span><span class="year">2016</span></p>
</div>
<button id="loadQuote">Show another quote</button>
</div>
<script src="js/script.js"></script>
<script>
setInterval(function() {
var randomColor = Math.round( Math.random() * 255 );
var element = document.getElementById("loadQuote");
},2000);
</script>
</body>
</html>
// FSJS - Random Quote Generator
/* I created an array[] of quote objects{} and I named it (quotes)
*/
var quotes = [
{ quote: "The biggest risk is not taking any risk... In a world that's changing really quickly, the only strategy that is guaranteed to fail is not taking risks.",
source: 'Mark Zuckerberg',
citation: "Facebook's Mark Zuckerberg -- Insights For Entrepreneurs",
year: '2011' ,
occupation: '--Former Programmer/Businessman',
},
{
quote: 'Not everything that can be counted counts, and not everything that counts can be counted.',
source: 'William Bruce Cameron ',
citation: 'Informal Sociology: A Casual Introduction to Sociological Thinking',
year: '1963' ,
occupation:'--Book Author',
},
{
quote: 'Life is 10% what happens to you and 90% how you react to it.',
source: 'Charles R. Swindoll',
citation: 'https://www.brainyquote.com/quotes/charles_r_swindoll_388332',
year: '2011' ,
occupation: '--Pastor/Author',
},
{
quote: 'Our greatest weakness lies in giving up. The most certain way to succeed is always to try just one more time',
source: 'Thomas A. Edison',
citation: 'https://www.brainyquote.com/quotes/thomas_a_edison_149049',
year: '1931' ,
occupation: '--Inventor/Businessman',
},
{
quote: "I'd rather attempt to do something great and fail than to attempt to do nothing and succeed.",
source: 'Robert H. Schuller',
citation: 'https://www.brainyquote.com/quotes/robert_h_schuller_155998',
year: '2015' ,
occupation: '--Pastor/Motivational Speaker'
}
];
/*
choose and then return a random quote
object from the array
*/
function getRandomQuote(array) {
var quoteIndex = Math.floor( Math.random() * (quotes.length));
for (var i = 0; i < array.length; i++) {
var randomQuote = array[quoteIndex];
}
return randomQuote;
}
// I created a second function and gave it (printQuote) as its name.
function printQuote() {
var message = ""; // This is the message variable with empty strings
var result = getRandomQuote(quotes);
message = "<p class='quote'>" + result.quote + "</p>";
message += "<p class='source'>" + result.source;
message += "<span class='citation'>" + result.citation + "</span>";
message += "<span class='year'>" + result.year + "</span>";
message += "<span class='occupation'>" + result.occupation + "</span>"
message += "</p>";
document.getElementById('quote-box').innerHTML = message;
}
printQuote();
// This event listener will respond to "Show another quote" button clicks
// when user clicks anywhere on the button, the "printQuote" function is called
document.getElementById('loadQuote').addEventListener("click", printQuote, false);
2 Answers

Erik Nuber
20,629 PointsTo change color when you push a button randomly, you need to consider what you are going to use for color. For example, rgb(red, green, blue); which takes a value from 0-255 for the red green and blue within the brackets. So to achieve what you are looking for without writing it for you, you need to get three random numbers, plug them into the rgb() and use javascript to then set the background color. I would be. happy to write it out for you but, hopefully having the concept you need to achieve this, you will be able to do it on your own.
You are also on the correct path with setInterval() to do anything you want after a set period of time.
setInterval(function(){
alert('hello world');
}, 1000);
The above will run a function every 1 second. You can also pass in a named function.
function namedFunction(0 {
alert('hello world');
}
setInterval(namedFunction, 1000);
This will do the same thing.
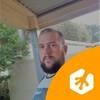
Daniel Languiller
Full Stack JavaScript Techdegree Graduate 33,396 PointsHi Erik, welcome to the tech degree :). Did you receive an invitation to the tech degree slack channel? I highly recommend you join and post questions about those projects over there in the slack. If you missed the email inviting you, please go ahead and send an email here: techdegree@teamtreehouse.com