Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial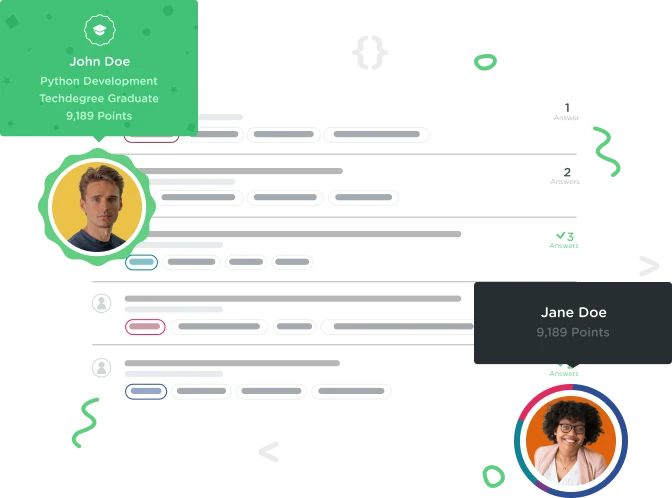

Steve Pierce
Courses Plus Student 1,091 PointsJavaScript - the numbers are not displaying properly for the countdown clock.
The review asks the following:
a) Hector has supplied you with a function named nextJuly4() that returns the date of the next 4th of July. Call the nextJuly4() function using thisDay as the parameter value and store the date returned by the function in the j4Date variable.
b) The countdown clock should count down to 9 p.m. on the 4th of July. Apply the setHours() method to the j4Date variable to change the hours value to 9 p.m. (Express value for 9 p.m. in 24-hour time).
c) Create variables named days, hrs, mins, and secs containing days, hours, minutes, seconds until 9 p.m. on the next 4th of July.
d) Change the text content of the elements with the IDs "dLeft", "hLeft", "mLeft", "sLeft" to the values of the days, hrs, mins, and secs variables rounded down to the next lowest integer.
Verify that the page shows the countdown clock shows the fireworks will begin in 46 days, 11 hours, 28 minutes, and 33 seconds. The countdown clock will not change because the script uses a FIXED date and time for the thisDay variable.
HTML File
<!DOCTYPE html> <html lang="en"> <head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<title>Tulsa's Summer Party</title>
<link href="tny_base.css" rel="stylesheet" />
<link href="tny_layout.css" rel="stylesheet" />
<script src="tny_timer.js" defer></script>
</head>
<body>
<header>
<nav class="horizontal">
<ul>
<li><a href="#">News</a></li>
<li><a href="#">Night Beat</a></li>
<li><a href="#">Tulsa Times</a></li>
<li><a href="#">Calendar</a></li>
<li><a href="#">City Links</a></li>
</ul>
</nav>
<img src="tny_banner2.png" id="logoImg" alt="" />
<h1>Tulsa's<br />Summer Party</h1>
<h2 id="timeHeading">Welcome to Tulsa</h2>
<div id="currentTime"><span>date</span><span>time</span></div>
</header>
<article>
<section>
<h1>Countdown to the Fireworks</h1>
<div id="countdown">
<div><span id="dLeft">58</span><br />Days</div>
<div><span id="hLeft">10</span><br />Hrs</div>
<div><span id="mLeft">14</span><br />Mins</div>
<div><span id="sLeft">48</span><br />Secs</div>
</div>
<p>Celebrate the nation's birthday at <em>Tulsa's Summer
Party</em>, a daylong extravaganza in downtown
Tulsa on the 4th of July. The festivities start at 9 a.m.
with a 5K and 10K walk or race. A great event
for the whole family. Sign up as an individual or
part of a team.
</p>
<p>The <em>Farmer's
Market</em> is also back with farm-fresh produce,
meats, and dairy. There's something for every
pallet.</p>
</section>
<section>
<p>Live music starts at 11 a.m. and continues through the day
with special appearances from <em>Falstaff
Ergo</em>, <em>Crop Circles</em>, <em>Prairie Wind</em> and
<em>James Po</em>.
</p>
<p>At 9 p.m. enjoy the <em>fireworks</em> that have won awards
as the best in the Midwest, designed and presented by
<em>Wizard Works</em>. Arrive early for the best seats!
</p>
<p>After the show, dance the night away to the music of
the <em>Chromotones</em>.
</p>
<p>See you on the 4th!</p>
</section>
<nav class="vertical">
<h1>Party Links</h1>
<ul>
<li><a href="#">5K and 10K Run</a></li>
<li><a href="#">Famer's Market</a></li>
<li><a href="#">Wizard Works</a></li>
<li><a href="#">Falstaff Ergo</a></li>
<li><a href="#">Crop Circles</a></li>
<li><a href="#">James Po</a></li>
<li><a href="#">Tulsa Fireworks</a></li>
<li><a href="#">Prairie Wind</a></li>
</ul>
</nav>
</article>
<footer>
<address>
Tulsa Summer Party ·
340 Main Street, Tulsa, OK 74102 ·
(918) 555-3481
</address>
</footer>
</body>
</html>
JS File
"use strict";
*/ showClock (); setInterval("showClock()", 1000);
function showClock() { var thisDay = new Date("May 19, 2018 09:31:27"); var localDate = thisDay.toLocaleDateString(); var localTime = thisDay.toLocaleTimeString();
/* Display the current date and time */
document.getElementById("currentTime").innerHTML = localDate + "<br />" + localTime;
}
function nextJuly4(thisDay) { var cYear = thisDay.getFullYear() + 1; var j4Date = new Date("July 4, 2018"); j4Date.setFullYear(cYear);
/* Calculate the days until 4th of July */
var days = (cYear - thisDay)/(1000*60*60*24);
/* Calculate the hours left in the current day */
var hrs = (days - Math.floor(days))*24;
/* Calculate the minutes and seconds left in the current hour */
var mins = (hrs - Math.floor(hrs))*60;
var secs = (mins - Math.floor(mins))*60;
/* Display the time left until 4th of July */
document.getElementById("dLeft").textContent = Math.floor(days);
document.getElementById("hLeft").textContent = Math.floor(hrs);
document.getElementById("mLeft").textContent = Math.floor(mins);
document.getElementById("sLeft").textContent = Math.floor(secs);
if ((j4Date - thisDay) < 0) j4Date.setFullYear(cYear + 1); return j4Date; }
1 Answer
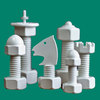
Steven Parker
231,261 PointsHere's a few observations/suggestions:
- I see where you defined "nextJuly4", but it doesn't look like you called it anywhere
- since it seemed missing, I added this line: "
nextJuly4(new Date("May 19, 2018 09:31:27"));
" - the stuff with "cDate" didn't make sense to me, tossed it and just used "j4Date" unmodified
- you forgot the time part of the target date
So after applying my hints, the modified function starts like this:
function nextJuly4(thisDay) {
var j4Date = new Date("July 4, 2018 21:00"); // 9 PM
/* Calculate the days until 4th of July */
var days = (j4Date - thisDay) / (1000 * 60 * 60 * 24);
// ... the remaining parts stay the same ...