Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial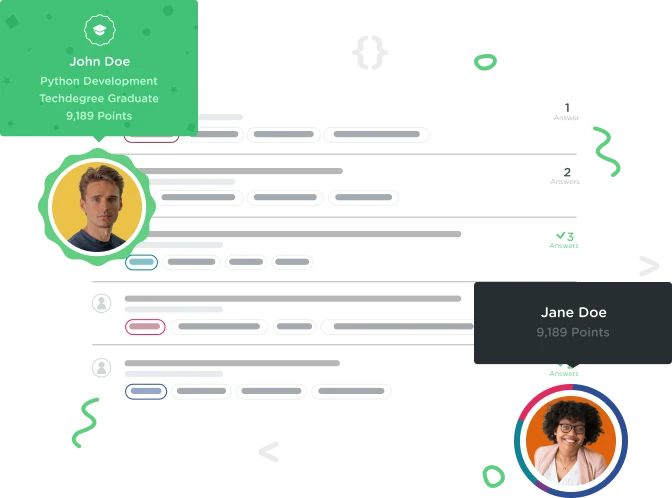

Riley Bolen
26,711 PointsJavaScript Tic-Tac-Toe
In an effort to practice JQuery I am trying to make a simple tic-tac-toe game using an unordered list as with 9 li's aranged in a 3x3 grid, and changing the background color of these li's instead of the x's and o's. I have figured out how to change the background color of a single li on click but I want to run a loop that alternates back and forth between the two following blocks of code:
$(listItem).click(function() { $(this).css("background-color", "red");
});
$(listItem).click(function() { $(this).css("background-color", "blue");
});
The idea is to be able to click on one li and have it turn red, then click on another and have it turn blue, then the loop starts over and the next one turns red and so on. Ive been fooling around with this all night but I cant figure it out, so I was hoping that someone could figure it out and show me how to do it! Thanks, Riley.
1 Answer

Jason Anello
Courses Plus Student 94,610 PointsHi Riley,
I think you'd want to have a variable that keeps track of whose turn it is. And that will tell you which color the background should be when an li is clicked on.
Another thing to consider is that the click handler should be detached from each li once it's clicked on because you should not be able to click on the same li again.
The .one() method would be good for this. It sets a one time event handler. After you click each li, the handler will be detached and they will no longer respond to clicks.
I would recommend taking a different approach on the css. In my opinion, it's easier to set up what styles you need in the css using class selectors. Then in the javascript all you're doing is adding and removing those classes. Right now you're only setting a background color. But if you expand on this then you'll likely have more styles to set.
Here's the javascript to do what I think you want:
var player = "x";
$('li').one("click", function() {
$(this).addClass(player);
player = player == "x" ? "o" : "x";
});
It sets up one time click handlers for all the li's. I purposely chose the value of the player variable to match the class names in the css so that when you need to add the class it's going to be the value of the player variable.
Then it's a matter of switching the player variable. If it's currently 'x' then make it 'o', otherwise make it 'x'. I used the ternary operator for that but you could do it with an if/else.
// the ternary operator is a shortened version of this if/else code
if (player == "x") {
player = "o";
} else {
player = "x";
}
Here's the css:
.x {
background: red;
}
.o {
background: blue;
}
Let me know if you have any questions about this.
Here's a codepen demo where I went a little further with it if you're interested. https://codepen.io/anon/pen/VKraxO
I added a reset button and x's and o's using the ::before pseudo element. I got the symbols from here: http://www.copypastecharacter.com/
Riley Bolen
26,711 PointsRiley Bolen
26,711 PointsThanks for the great answer! I've never seen those ternary operators before, but they are going to be very handy in the future. Now that the basic functionality is there, i'm going to try to improve it on my own first, but if I get stuck that codepen demo you made will definitely be helpful! Riley