Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial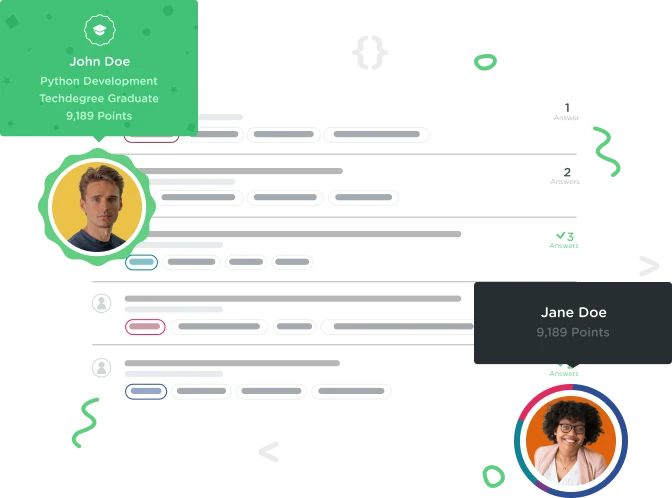
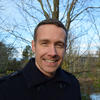
Stephen O'Connor
22,291 PointsJavaScript True/False Question
I am learning JS from scratch - for about the 10th time now - to try and understand fully what is going on, I would like to understand vanilla JS in full before using a framework like jQuery etc.
I'm going through the JS Track and in a video in the loops course there is the following code:
var randomNumber = getRandomNumber(10);
var guess;
var guessCount = 0;
var correctGuess = false;
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
do {
guess = prompt('I am thinking of a number between 1 and 10. What is it?');
guessCount += 1;
if (parseInt(guess) === randomNumber) {
correctGuess = true;
}
} while ( ! correctGuess )
document.write('<h1>You guessed the number!</h1>');
document.write('It took you ' + guessCount + ' tries to guess the number ' + randomNumber);
Dave talks about the do while loop and the test condition 'checks to make sure the test condition is not true'. But from what I can see the test condition is not false, because correctGuess is set to false earlier in the code? So surely '! correctGuess' means not false, which is true, not 'not true'???
I'm a little confused. If someone could help me out and stop my head from aching I'd appreciate it.
Thanks!
11 Answers
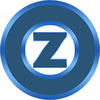
Daniel Gauthier
15,000 PointsHey again Stephen,
When I first read your question, I noticed that in order to understand the logic of what you were trying to say, I had to read the code almost literally.
I can definitely see the logic and why it lead to this confusion, so going to try another approach.
The ! operator doesn't alter the contents of a variable, it simply checks to see if the contents of the variable are true or false. By writing "while ( ! variable )" we're just saying "As long as the contents of the variable passed to you are not true, execute the loop again. Once the variable contains true, stop the loop.
For a visual, as the loop hits the line:
while( ! correctGuess )
The program isn't asking the ! operator to change the contents of correctGuess, it's just asking the ! operator to determine if the value in the variable is true or false. It's not asking the ! operator to make a judgment or any changes to the contents. It just asks that the loop be continued if the contents of correctGuess are false (not true), or to stop the loop if the contents of correctGuess are true.
The confusion stems from misunderstanding how the ! operator works. It will simply determine the value of the variable passed to it and choose a path accordingly. It will not change whether the contents of the variable are true or false.
The idea that the ! operator will convert the value, since that is how logic would work in the literal sense, resulting in the code:
correctGuess = false;
do {
<code>
} while (! correctGuess)
equating to true on the first run is incorrect.
Something would have to change the value of correctGuess in the loop to true to end the loop.
The line "while (! correctGuess)" simply means "While the contents of correctGuess do not equate to true".
In the end, remember that the ! operator can't turn a false into a true, it's just checking to see what the variable contains at the moment it's asked to look.
I hope this helps make it a little more clear, and again, I can totally understand where the confusion is coming from.
Stay awesome!
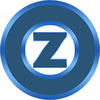
Daniel Gauthier
15,000 PointsHey again Stephen,
I just had another idea about how to explain this, so throwing it up here in hopes one of them helps :D
To really break the problem down to the core, the confusion seems to come from the name of the ! operator, which affects the perception of what one might believe it will do.
Instead of calling it the NOT operator, give it a different name, like the checker operator.
Thinking of it under a different name, it will be easier to understand the concept that the operator will not affect the logic of a statement.
The problem of thinking of it as "Not false has to be true" would not be there if it wasn't for the name of the operator.
If you think of the line "while ( ! correctGuess )" as "While the checker operator is passed a value of false from the correctGuess variable, execute the loop, and if the checker operator is passed a value of true, end the loop", it will get rid of the confusion brought on by the name.
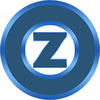
Daniel Gauthier
15,000 PointsHey Stephen,
From the way you explain getting to your point of confusion, it's easy to see how anyone could get confused. I had to second guess myself for a second looking at it from that angle :D
Basically, the do/while loop says:
"Do everything between the braces, while correctGuess does not equate to true."
Since we initialize it as false, we have to wait for the player to guess the correct number before the if statement changes the value to true.
What keeps the loop running is the fact that correctGuess equates to false (not true), and the ! operator is simply waiting for the correctGuess variable to return a value of true.
Hope this helps!
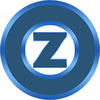
Daniel Gauthier
15,000 PointsHey again Stephen,
I actually thought of an easier way to explain this as I hit post, so I'll just throw this here also:
The code:
while ( correctGuess )
basically means "While correctGuess is true".
Which means that the code:
while ( ! correctGuess)
means "While correctGuess is not true".
Good luck!

Peter Furler
31,647 PointsYou initialize correctGuess with the following value: correctGuess = false;
It is used in the loop as follows: while ( !correctGuess) Which is is equivalent to this: while ( !false)
Though because you are using the negation operator (!), this will reverse the Boolean value and instead make it true. So the expression within the while loop condition will be evaluated as follows: while (true)
It will only stop looping when the correct guess is made as it will be true, therefore the negation operation will make it false.
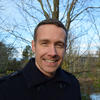
Stephen O'Connor
22,291 PointsHello.
Thanks for your answers, however, I am still confused, maybe it's the wording Dave uses in the video, or maybe I just don't get it... Some of the JS logic does leave my head hurting at times.
From my understanding conditions (of any kind) rely on a true or false value, and here we explicitly set 'correctGuess' to false. So 'while(!correctGuess)' in my head means 'while(!false)' which is true? Using the not operator has reversed the boolean value, from false to true, right?
If that's correct then I don't understand how the following can be the case.
while ( correctGuess )
basically means "While correctGuess is true".
Which means that the code:
while ( ! correctGuess)
means "While correctGuess is not true".
Here you say 'while(correctGuess) basically means 'While correctGuess is true'. How can that be if we have already set correctGuess to be false earlier in the code? And why does Dave say 'checks to make sure the test condition is not true'. I guess I am 'not getting' the 'not operator' ha. It's too early in the morning for this kind of headache!
If Peter, Daniel, Dave or anyone can help clarify this further for me you'll make me a very happy man.
Thanks!
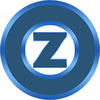
Daniel Gauthier
15,000 PointsHey again Stephen,
The bump you seem to be hitting is:
"Here you say 'while(correctGuess) basically means 'While correctGuess is true'. "
Which isn't the line that appears in the code. I added that for context.
After initializing the variable to false, we're actually using the line:
while ( ! correctGuess)
which means "While correctGuess is not true".
Since the line "while ( ! correctGuess)" evaluates to false until the user guesses the correct number, the loop continues to iterate.
Hope that clears things up!
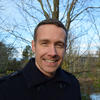
Stephen O'Connor
22,291 PointsHi Daniel,
Thanks for the response. So does the value in correctGuess within the loop condition not matter? Because I am still seeing it as not false. In my head I see the following:
var correctGuess = false; So, while(!correctGuess) is the same as while(!false), which is true.
But you are saying:
while ( ! correctGuess) which means "While correctGuess is not true".
Does that make sense? (there is some irony in that statement ha).
Thanks.
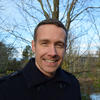
Stephen O'Connor
22,291 PointsThanks Daniel! I now understand. I was indeed misunderstanding the not operator, I thought it changed the value of the variable, but now understand that it actually does not do that. Which makes the code make sense, which makes me happy!
Thanks again.
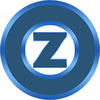
Daniel Gauthier
15,000 PointsAwesome, good luck with the next section :D
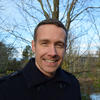
Stephen O'Connor
22,291 PointsThanks. The weird thing is I have done almost the full 'Full Stack JS' track, so I should know this by now.
I was reading about OOP in JS the other day and a few things were still confusing me so decided to go back and do the basics again. One day it will all click, one day ... haha.