Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial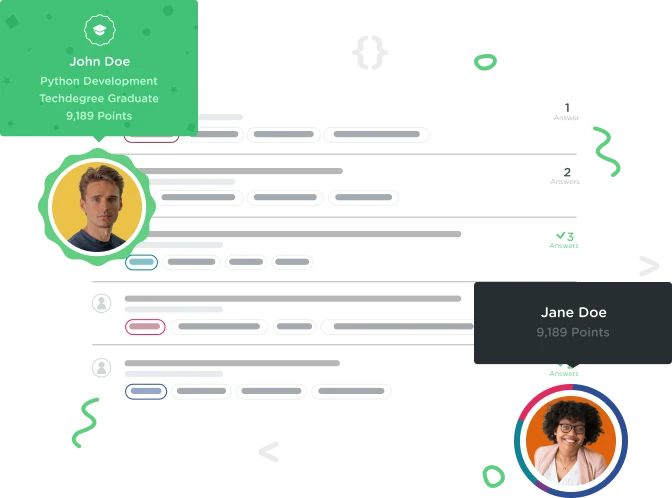
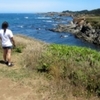
Nancy Melucci
Courses Plus Student 35,157 PointsJavaScript Two Buttons Two onClick Functions
I need to add a button that deletes list times from base code that adds items to a to-do list.
At this point, I make the second button I add do anything but the same function that the "add task" button does.
I am using a "alert" message to test the code.
I am posting the code for the html, css and js. There is a minor problem with the css but that is not a concern now. I have to figure out how to get the Delete button to perform a different function than the Add button.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>To-Do List</title>
<!--[if lt IE 9]>
<script src="http://html5shiv.googlecode.com/svn/trunk/html5.js"></script>
<![endif]-->
<link rel="stylesheet" href="css/form.css">
</head>
<body>
<!-- Script 6.5 - task.html -->
<form action="#" method="post" id="theForm">
<fieldset><legend>Enter an Item To Be Done</legend>
<div><label for="task">Task</label><input type="text" name="task" id="task" required></div>
<button onClick = "addTask()"> <input type="submit" value="Add It!" id="submit"></button>
<button onClick = "removeTask()"><input type="submit" value="Remove It!!" id="delete"></button>
<div id="output"></div>
</fieldset>
</form>
<script src="js/tasks.js"></script>
</body>
</html>
The JavaScript
// This function does all the work.
// It is immediately-invoked.
(function(){
// Variable that stores the tasks:
var tasks = [];
// Function called when the form is submitted.
// Function adds a task to the global array.
function addTask() {
'use strict';
var task = document.getElementById('task');
var output = document.getElementById('output');
var message = '';
if (task.value) {
tasks.push(task.value);
message = '<h2>To-Do</h2><ol>';
for (var i = 0, count = tasks.length; i < count; i++) {
message += '<li>' + tasks[i] + '</li>';
}
message += '</ol>';
output.innerHTML = message;
} // End of task.value IF.
// Return false to prevent submission:
return false;
}
// End of addTask() function.
function removeTask(){
alert("Button Clicked!");
}
// Initial setup:
function init() {
'use strict';
document.getElementById('theForm').onsubmit = addTask;
} // End of init() function.
window.onload = init;
})();
The CSS in case you need it.
body{
font-family:"Lucida Grande", "Lucida Sans Unicode", Verdana, Arial, Helvetica, sans-serif;
font-size:12px;
color: #1b1d26;
background:#F1F2D8;
}
p, h1, form, button{border:0; margin:0; padding:0;}
.spacer{clear:both; height:1px;}
/* ----------- My Form ----------- */
form{
margin:0 auto;
width:400px;
padding:14px;
background-color: #ffffff;
border:solid 2px #425955;
}
/* ----------- stylized ----------- */
h1 {
font-size:14px;
font-weight:bold;
margin-bottom:8px;
}
h2 {
clear: both;
}
p{
font-size:11px;
color:#666666;
margin-bottom:20px;
border-bottom:solid 1px #BFBD9F;
padding-bottom:10px;
}
label{
display:block;
font-weight:bold;
text-align:right;
width:140px;
float:left;
}
.small{
color:#666666;
display:block;
font-size:11px;
font-weight:normal;
text-align:right;
width:140px;
}
select{
float:left;
font-size:12px;
padding:4px 2px;
border:solid 1px #BFBD9F;
width:200px;
margin:2px 0 20px 10px;
}
input{
float:left;
font-size:12px;
padding:4px 2px;
border:solid 1px #BFBD9F;
width:200px;
margin:2px 0 20px 10px;
}
#submit{
clear:both;
margin-left:150px;
width:125px;
height:31px;
background:#F1F2D8;
text-align:center;
line-height:20px;
color:#000000;
font-size:12px;
font-weight:bold;
}
#delete{
clear:both;
margin-left:150px;
width:125px;
height:31px;
background:#F1F2D8;
text-align:center;
line-height:20px;
color:#000000;
font-size:12px;
font-weight:bold;
}
#output {
clear:both;
margin-bottom: 10px;
color: blue;
}
2 Answers
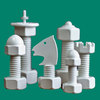
Steven Parker
230,274 PointsYou might want to redefine your buttons another way.
Since a "submit" input is already a button, and you want the other button to not be related to submit:
<input type="submit" value="Add It!" id="submit">
<button type="button" onclick="removeTask()" id="delete">Remove It!!</button>
Then, in the JavaScript you need to move the definition of removeTask outside of the IIFE (immediately invoked function expression) because otherwise it is contained in that function's scope and not accessible by the HTML code.
Alternately, you could establish the handler for the delete button in the init function similarly to how you currently establish the submit handler.
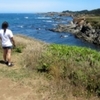
Nancy Melucci
Courses Plus Student 35,157 PointsThanks. I don't have a lot of experience with JS I found a version of the same code without the automatically invoked function. So I am continuing with that....I got the alert button to work.