Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial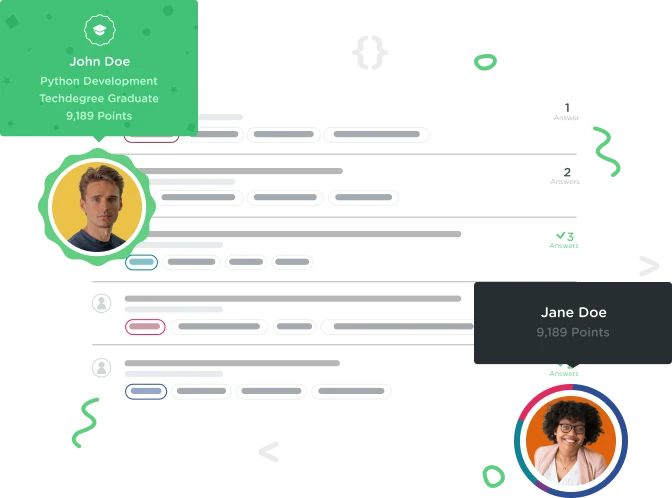

RODRIGO MARTINEZ
5,912 PointsJavascript Unit Converter with 2 dropdowns menus.
Hello everyone. I'm new to javascript. Trying to build, for practice, a simple unit converter kind of like the one that google uses. I'd like to have an input box for entering the number value. After that, two <select> lists in the HTML files so i can choose in first <select> the unit i convert from; and on the second <select> the unit i convert to. Maybe is an obvious one but i have not been able to find an answer to this problem. I'll post the last code/approach i tried.
HTML
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title></title>
<script src="simpleweightconversortest.js"></script>
</head>
<body>
<form>
<!-- Select 1-->
<label>From:</label>
<select name="converts" id="Selection">
<option>Chose Option</option>
<option value="kilograms1">Kilograms</option>
<option value="grams1">Grams</option>
<option value="miligrams1">Miligrams</option>
</select>
<!-- Select 2-->
<label>To:</label>
<select name="converts2" id="Selection2">
<option>Chose Option</option>
<option value="kilograms2">Kilograms</option>
<option value="grams2">Grams</option>
<option value="miligrams2">Miligrams</option>
</select>
<!--INPUT-->
<br>
<br>
<br>Value
<input type="number" id="value" placeholder="Insert value">
<!--RESULT -->
<br>Conversion
<input type="text" id="convertedOuput">
<br>
<br>
<input type="Button" onclick="Conversion()"
value="convert">
</form>
</body>
</html>
JAVASCRIPT
var val = document.getElementById("value").value;
var convertedOuput =document.getElementById("convertedOuput");
var selectFrom = document.getElementById("Selection");
var selectTo = document.getElementById("Selection2");
var madeSelection_1 = selecFrom[selectFrom.selectedIndex].value;
var madeSelection_2 = selectTo[selectTo.selectedIndex].value;
function Conversion() {
if (madeSelection_1 == "kilograms1" && madeSelection_2 == "kilograms2") {
var result = val * 1;
document.getElementById('convertedOuput').innerHTML = result;
}
}
1 Answer
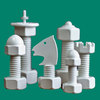
Steven Parker
231,007 PointsYou're close, but:
- you need to sample the control values inside the function (instead of before they are set)
- you don't need to index the select, its "value" is the value of the selected option
- inputs are not allowed to have content, so set the "value" of the input control instead
- you might want to display something when the conditions aren't set for calculating
- since you already referenced the input control, use "convertedOuput" instead of repeating
getElementById
function Conversion() {
var val = document.getElementById("value").value;
var madeSelection_1 = selectFrom.value;
var madeSelection_2 = selectTo.value;
if (madeSelection_1 == "kilograms1" && madeSelection_2 == "kilograms2") {
var result = val * 1;
convertedOuput.value = result;
} else
convertedOuput.value = "Make selections first!";
}
RODRIGO MARTINEZ
5,912 PointsRODRIGO MARTINEZ
5,912 PointsHello Steven. Thank you so much for taking the time to help me. I just tried out the code you gave me and i'm still not beeing able to make it work. When i click the button, it does nothing. I completely understand what you wrote. And it's very logic. Now i just don't get why it's not working. Am I missing something out in the HTML file? Very confused about it.
Thanks again, Rodrigo
RODRIGO MARTINEZ
5,912 PointsRODRIGO MARTINEZ
5,912 PointsI'm getting this message in the console
simpleweightconversortest.js:11 Uncaught TypeError: Cannot read property 'value' of null at Conversion (simpleweightconversortest.js:11) at HTMLInputElement.onclick (INDEX.html:41) Conversion @ simpleweightconversortest.js:11 onclick @ INDEX.html:41
RODRIGO MARTINEZ
5,912 PointsRODRIGO MARTINEZ
5,912 PointsSolved it. Thanks a lot. I'll share later my final code later. It works but seems like a lot of code. And I don't know if there's a way i could clean it up a bit. Thanks again.