Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial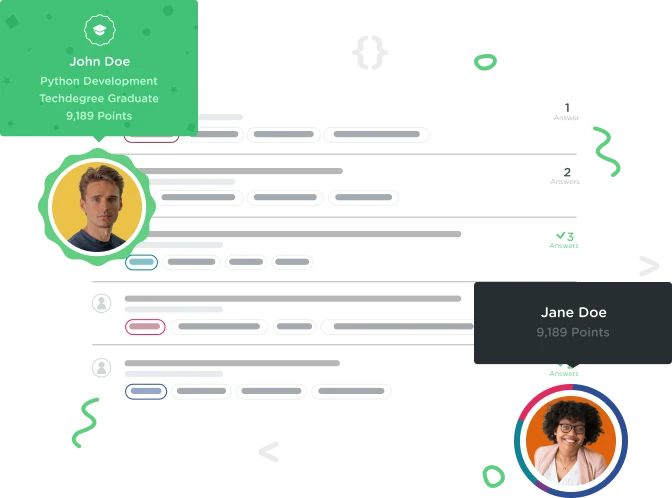

Kathryn Notson
25,941 PointsJavaScript Unit Testing > Behavior Driven Development with Mocha and Chai > A Testing Test!
This code doesn't work which is straight from the Chai assertion library. Clone.js doesn't work correctly, expected test to fail, but it passed.
var expect = require('chai').expect;
describe('clone', function () {
var clone = require('./clone.js');
it('some description string', function () {
expect({foo : 'bar'}).to.deep.equal({foo : 'bar'});
})
})
function clone (objectForCloning) {
return Object.assign({}, objectForCloning)
}
module.exports = clone
5 Answers

Kathryn Notson
25,941 PointsTimothy Schmidt:
Here's one example that I used: "Expect(clone).to.deep.equal({});" clone.js works correctly, specs don’t pass

Timothy Schmidt
4,806 PointsIn the code you provided, you're not actually using the clone function.
var expect = require('chai').expect;
describe('clone', function () {
var clone = require('./clone.js');
it('some description string', function () {
// use clone function to clone an object.
var objClone = clone({ foo : 'bar' });
expect({foo : 'bar'}).to.deep.equal( objClone );
})
})

Kathryn Notson
25,941 PointsTimothy Schmidt:
I don't understand what you said. The clone function in clone.js is exported & the code challenge question uses it.

Timothy Schmidt
4,806 PointsThe question doesn't use it on it's own. You're meant to use the clone function inside your unit test. So, inside your it
function, you need to use clone
and then use expect
to test that the function provided the correct result.

Kathryn Notson
25,941 PointsTimothy Schmidt:
I've tried multiple iterations of "expect(clone).to.deep.equal(objectForCloning);", but nothing passes correctly so I can't complete this code challenge. I've read the Mozilla.org documentation on Object.assign() & also the Chai assertion library. Nothing has hit me to have an "Aha!" moment to realize what the correct answer is. My logic must be faulty.

Timothy Schmidt
4,806 PointsThat's strange. I went to the code challenge before posting to ensure my code worked before posting it. Have you tried using my solution?
If you could comment with one of your examples of using expect(clone).to.deep.equal(objectForCloning);
, maybe I can see what's wrong.

Kathryn Notson
25,941 PointsTimothy Schmidt:
Thanks! That worked. I don't know what I was doing wrong unless I wasn't including enough () within the (clone()). This quiz question drove me nuts for the last 2 1/2 months! I was mulling over what I read in MDN & what the instructor said about ".deep.equal" in the video. I was going to review it all over again today, but you saved me the effort.
Timothy Schmidt
4,806 PointsTimothy Schmidt
4,806 PointsOk, I think I know where the misunderstanding is.
clone
isn't the object you're supposed to test, it's a function that is used to make the clone. You pass an object to theclone
function and the result is what you need to test. So, using the example in your last comment, it would be:expect( clone({}) ).to.deep.equal({});
But, it apparently doesn't work with empty objects, so you need to add a key to the object.
expect( clone({key: "value"}) ).to.deep.equal({key:"value"});
That line passed the exercise for me.