Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial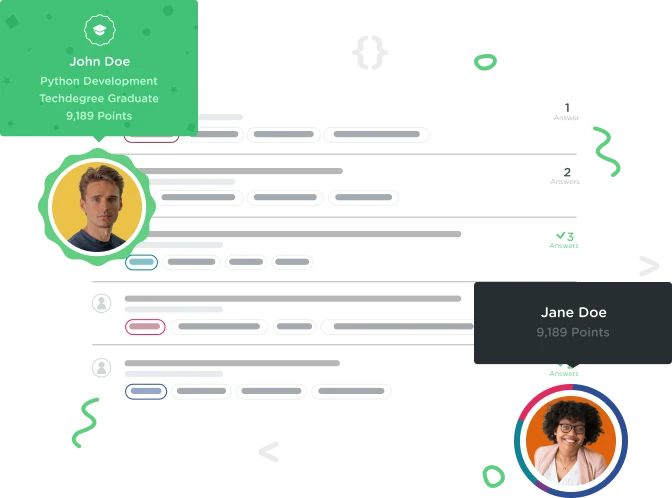
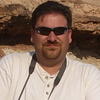
Harold Stewart
9,322 PointsJavascript unit testing challenge
I must be misunderstanding the goal. I thought it was to write a test case that catched an expected error message. But when checking my work, I get the message " We tried your spec with a version of subtraction.js that DOESN'T work correctly, expecting the test to fail. But it passed! Better try again!". I though passing the test was to goal. Can someone help me understand to he goal of this challenge?
var expect = require('chai').expect
describe('subtraction', function () {
var subtraction = require('../WHEREVER')
it('only works with numbers', function () {
expect(subtraction("A","B").to.throw("subtraction only works with numbers!");
})
})
function subtraction (number1, number2) {
if (typeof number1 !== 'number' || typeof number2 !== 'number') {
throw Error('subtraction only works with numbers!')
}
return number1 - number2
}
2 Answers
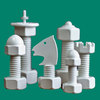
Steven Parker
241,809 PointsWhen used with "to.throw", the expect function takes another function as a parameter, not the result of calling one.
Also, you're a missing a close parenthesis before .to.throw
.
But the main issue is the argument passed to expect. You could build a "handler" function and pass that as was done in the video example, or you could curry the arguments and pass the function using bind:
expect(subtraction.bind(null,"A","B")).to.throw("subtraction only works with numbers!");
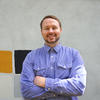
Tom Geraghty
24,174 Points@Steven, I always appreciate your answers and reminding us of argument currying; I would not have been able to solve this challenge based on the lecture alone without your reminder that expect wants a function and not the result of the call of that function as it's parameter.
I also wanted to put up my answer that, I believe, demonstrates the same techniques used in the video lecture without confusing people who aren't as familiar with .bind() and currying parameters.
describe('subtraction', function () {
var subtraction = require('../WHEREVER') // anyone else find this confusing? I'd rather Treehouse just hid this or made it refer to the actual imported file's location (which I understand is difficult in Workspaces, but you guys are pros figure it out!)
it('only works with numbers', function () {
var handler = function(a,b) { subtraction(a,b) }
expect(handler).to.throw(Error)
expect(handler).to.throw('subtraction only works with numbers!')
})
})
This will pass the test and matches with Guil's code from the videos/source project files.
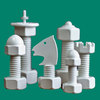
Steven Parker
241,809 PointsThat was actually my first suggestion (though I didn't illustrate it), but don't you still need to call "subtraction" with defined non-numeric arguments for the test to work?
Sam Donald
36,305 PointsSam Donald
36,305 PointsTo be clear
expect
will take the result of a called function.But in this case with
throw
, it requires the use of a callback function.