Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial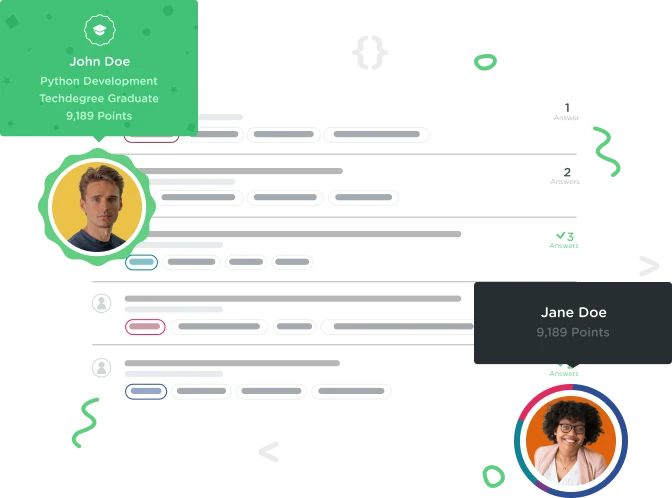

Daryl Carr
18,318 PointsJavaScript Validation
Hi all
I was just wondering, what is the most recent/best practice way to validate forms with JavaScript. Should we use the inbuilt HTML5 validation and use JavaScript to complement? Is there any good recent guides on how to do so?
For example I have built the below HTML & JavaScript. Is this right or should I be doing it a different way?
A final question, when the submit button is clicked, if there is an alert, all the values in the inputs are deleted, is there a way to stop this from happening? Also any tips, advice on the below is welcome too!
Thanks in advance!
Daryl
HTML
<fieldset>
<label for="email">Email:</label>
<input type="email" id="email" name="user_email" required>
<label for="password">Password:(Over 6 letters, at least 1 number)</label>
<input type="password" id="password">
<label for="confirm-password">Confirm Password:</label>
<input type="password" id="confirm-password" name="user_password">
</fieldset>
JavaScript
var submitButton = document.getElementById("submit");
var email = document.getElementById("email");
var pword = document.getElementById("password");
var confirmPword = document.getElementById("confirm-password");
//-------------------FUNCTIONS------------------
function validateEmail(email) {
var re = /^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/;
return re.test(email);
}
function pwordValidation(pword) {
var hasNumber = /\d/;
return hasNumber.test(pword);
}
function validateForm() {
if ( !email.value ) {
alert("Enter email.");
email.focus();
} else if ( !validateEmail(email.value) ) {
alert("Invalid email entered.");
email.focus();
} else if (pword.value.length < 6) {
alert("Password too short. Password must be 6+ characters.");
pword.focus();
} else if ( !pwordValidation(pword.value) ) {
alert("Password does not include a number. Password must include at least 1 number.");
pword.focus();
} else if (pword.value !== confirmPword.value) {
alert("Passwords do not match");
pword.focus();
}
}
//-------------------WIRING-------------------
submitButton.addEventListener("click", validateForm);
2 Answers

Matt F.
9,518 PointsHi Daryl,
What you are doing works. Using the HTML validation alongside JavaScript validation before submitting the form data to the server is good for user experience.
Keep in mind, however, that the validation on the server is going to be more important (for security reasons). Generally the front end handles all of the common cases, and then the server handles all of the known cases - in case someone is POSTing directly to the server.
Most of my experience validating forms has come from using the methods recommend in Angular and React - so if you end up using a library/framework, it is good to look at the respective best practices.
In regards to your second question - I do not think that you should ever rely on the form clearing itself - instead it should redirect or clear once validation (as well as the server responding with success). I believe that preventing the submit button's default actions will solve this for you, but I am not certain.
Give this a shot:
submitButton.addEventListener("click", function(event){
event.preventDefault();
validateForm();
});
If that solves the issue, then you will need to write a function that clears the form values - which you call after validation or POSTing succeeds.

Daryl Carr
18,318 PointsHey Matt, thanks for replying!
I think in my haste I phrased the second question badly or I simply do not understand what your code is doing haha!
What I was trying to say was that in the case that one of the inputs is invalid, at the moment an alert is thrown, when the user clicks ok on the alert, all the information in the form is deleted. I just wanted to prevent that from happening so that the user can edit the incorrect input without losing all of their information in the other inputs until all the inputs are validated.
Is this what your code is referring to?
Thanks alot!
Daryl

Matt F.
9,518 PointsHi Daryl,
I believe that the preventDefault should clear that up - can you add the rest of your HTML so I can test it and see?

Matt F.
9,518 PointsAlso, here is some solid documentation on preventDefault
https://developer.mozilla.org/en-US/docs/Web/API/Event/preventDefault

Daryl Carr
18,318 PointsHey, I used your code and it's working just how I wanted! I finally re-read it the 20th and it made sense to me!
I'll keep working on the whole thing and post it up later to see what you think/ any suggestions if thats ok with you?
Thanks again for the code ;)

Matt F.
9,518 PointsGlad that it worked!
Always glad to help if something else comes up.