Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial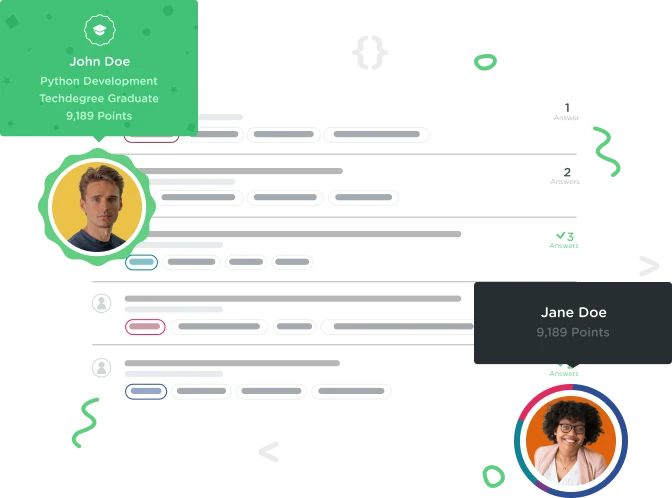

William J. Terrell
17,403 PointsJavascript Variable with {}
It doesn't have anything to do with any of the tutorials (that I know of at least), but I was just wondering if anyone could tell me what the following syntax means:
var dict = {};
Is this just an empty JavaScript Object? There is some more code that follows it, some examples are:
dict.age = { 'active': false, 'title': 'Age', 'intValue': 0 };
dict.livingStatus = { 'active': false, 'title': 'Living arrangement', 'intValue': 0, 'textValue': '' };
dict.maritalStatus = { 'active': false, 'title': 'Marital Status', '0': 'No', '1': 'Yes', 'intValue': '' };
dict.numberOfChildren = { 'active': false, 'title': 'Children', '0': 'No', '1': 'Yes', 'intValue': '' };
I'm just trying to figure out how the code works and better my understanding. :)
Thanks!
2 Answers

Joe Cochran
18,249 PointsYou are absolutely correct. the line:
var dict = {};
creates a new empty javascript object. Then, in the second block of code, different keys are being set to contain javascript objects themselves! This could be reformatted as:
var dict = {
'age': {
'active': false,
'title': 'Age',
'intValue': 0
},
'livingStatus': {
'active': false,
'title': 'Living arrangement',
'intValue': 0, 'textValue': ''
},
'maritalStatus': {
'active': false,
'title': 'Marital Status',
'0': 'No',
'1': 'Yes',
'intValue': ''
},
'numberOfChildren': {
'active': false,
'title': 'Children',
'0': 'No',
'1': 'Yes',
'intValue': ''
}
};
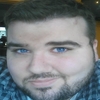
Marcus Parsons
15,719 PointsHey William,
That initialization just creates an empty JavaScript object as you said. Once you create the object, you can attach anything that you want to it so long as follows the rules for setting properties of an object and doesn't violate protected keywords.
So, in that 2nd set of commands, you are setting these initial object properties and just adding more properties to each one. And when you go to call one of those values that you need, there are multiple ways to do so:
//Let's say we want the intValue for the age reference of dict
dict.age.intValue;
//Or:
dict.age["intValue"];
//Or:
dict["age"].intValue;
//Or:
dict["age"]["intValue"];
The key thing to remember, however, is that when you are making calls to these properties in the object, if your property has spaces or characters that are unsupported by a typical variable name, you need to use the [""] method of calling that property.
For example:
//Let's say we set the housingType property and then set a sub property of "House Type" to "house"
dict.housingType = {"House Type" : "House" };
//And we want to call that:
dict.housingType.House Type //gives an error
dict.housingType."House Type" //also gives an error
//However, we can call those like this:
dict.housingType["House Type"];
//Or:
dict["housingType"]["House Type"];
Does that make sense?