Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial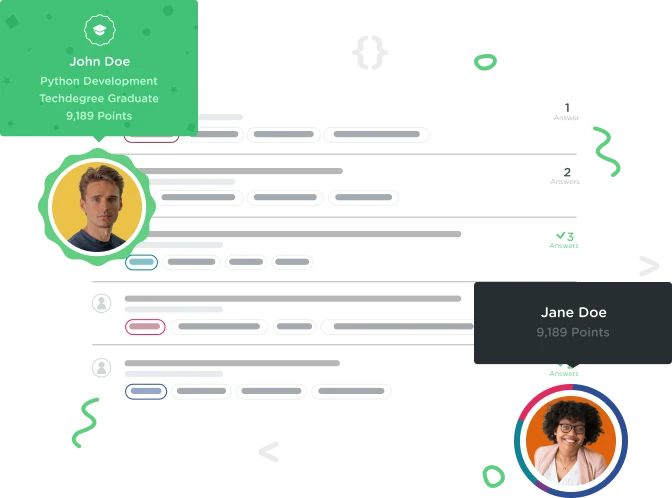

Jeff Xu
10,291 PointsJavascript's Array.sort() question
Hi, please consider the follow code:
var myArray = [1,2,3,4,5,6]; //define an array of numbers from 1 to 6 inclusive
myArray.sort(function() {return 1;}) //returns [6,5,4,3,2,1] since a positive number means the pair in the bubble sort algorithm get reversed since when a-b, a has to be bigger than b for the expression to evaluate to a positive number
//but then why does...
myArray.sort(parseInt(8)); //not reverse everything in the array like when we call a function and return a positive number? Thanks! I think maybe I just don't understand return. It's just suppose to put out a value to feed into the .sort() method right? so if we feed a positive int directly into it, when is it not the same as returning a positive int?
1 Answer

Josh Haywood
5,621 PointsYes, I think you might be confused by how 'return' works. Return is used to stop the evaluation of a function and return the value to the caller of the function. Since you do not declare a sortFunction, you are unable to return a value to sort() to determine sort order. So to keep going with your example:
myArray.sort(function(){return parseInt('8');}); returns myArray in reverse order as expected; however, myArray.sort(return parseInt('8')); does not, because only a function can return a value to the caller.
This was confusing to me at first too, but reading the explanation of the specs on the MDN website really helped me understand why this works the way it does, rather than blindly accepting it as 'just the way it works'.
Jeff Xu
10,291 PointsJeff Xu
10,291 Pointshttps://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/return
Thanks for the suggestion to look up the article! I see that return is kind of like break, except the function returns a value upon existing the loop. I m still a little confused as to why we need a function to return a value as opposed to just suggesting a positive number ourselves to feed into the .sort() method, which I understand to be kind of like a predefined function of the window. So it's like window.sort or something like like that.
Josh Haywood
5,621 PointsJosh Haywood
5,621 PointsYes, sort() is part of the array prototype, so you could think of it as a predefined function to act on arrays, but because it is part of the prototype, it has a very specific definition. Per the specs for Array.prototype.sort(), the sort function accepts only one argument which must be a function that returns a value to pass to the internal sort() algorithm. After looking into it a little further, it seems like the example I had above
might not even technically meet the specs because the above compareFunction doesn't accept two arguments for comparing the values in the array, but it does seem to work anyhow.
I'm a lot like you in that I really like to know the why and how for a lot of this stuff, but at some point the why and how becomes "because the really smart people writing the specs decided that's the best way". Maybe one day we can be the smart guys and make the specs how we like it :)
Jeff Xu
10,291 PointsJeff Xu
10,291 PointsSweet! Ya, the way I visualize coding is that each language have these little tiny pieces of logic "lego blocks" that we have to assemble them into more useful parts like functions and eventually into hopefully something useful. Each language is meant to tackle a different issues in slightly different way. For example, Ruby was meant to be more English like, etc. And since there's soo many unique "lego blocks" for each language and so many ways they are meant to interact with each other, a lot of programming comes down to Googling documents and code examples of how other people tackled a similar problem. But on a high level, we sort of intuitively know what a language should be capable of because we use things they create every day. But it's like we have to take a concept and progressively break it down into small and more specific tasks that small pieces of logic statements can accomplish while not getting too bummed out about missing semi colons and forgetting to add an "s" in a function name, and the hours of debugging that naturally comes with them.