Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial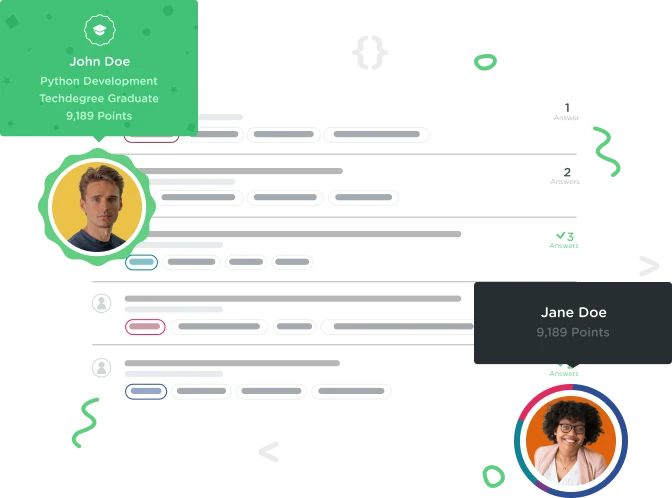
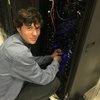
Austin Badolian
635 Pointsjava.util.NoSuchElementException
Getting the error above, looked at other posts about this regarding repl, but couldn't pinpoint my problem. Have a look.
public class PezDispenser {
//initializing integer for state of dispenser that is constant
public static final int MAX_PEZ = 12;
//Storing number of pez in dispenser, not initialized
private int mPezCount;
//The member varaible of class PezDispenser
private String mCharacterName;
//Constructor of class
public PezDispenser(String characterName) {
mCharacterName = characterName;
mPezCount = 0;
}
//Loader for pez method
public void load() {
mPezCount = MAX_PEZ;
{
//Methods always return something. This one returns the character name
public String getCharacterName() {
return mCharacterName;
}
//Check if pez is empty method
public boolean isEmpty() {
return mPezCount == 0;
}
}
2 Answers
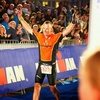
Steve Hunter
57,712 PointsHi Austin,
That's telling the compiler that your method will return something and what type that something is. It's not just setting the data type. You set the type for mCharacterName
in the class with:
private String mCharacterName;
You then want to return that from the getter method. Java already knows that mCharacterName
is of type String
but wasn't aware that your method wanted to return a String
until you added the keyword in the method skeleton:
public String getCharacterName() { // public method that returns a string
return mCharacterName; // the already-declared string being returned
}
I hope that made some sense.
Steve.
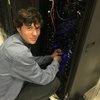
Austin Badolian
635 PointsThanks Steve that clears it up a little. So even though I set the data type when I created the variable. I have to also let the compiler know that a method returns a specific data type?
If that makes sense?
So if I declare a variable named mGetCharacterName, that is a string. I have to also let the method know what it's returning, which is also a string?
And that would apply to any data type?
So if I declared it as String but the method didn't return anything then I would set it as void.
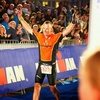
Steve Hunter
57,712 PointsHi Austin,
Yes, your method signature lets the compiler know a few things; whether the method is private, public, protected etc., the name of the method, any parameters (with their type) that the method receives and the data type that it will return (or void if nothing is returned).
Steve.
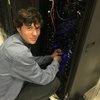
Austin Badolian
635 PointsAwesome, thank you l. That makes perfect sense.
Austin Badolian
635 PointsAustin Badolian
635 PointsAfter some trial and error and trying to run the compiler javac, it gave me the error messages stating that I had not declared the data type. which is a big no no.
It should have been this:
Remember to let the compiler know what your Data Types are. Hope that helps anyone who has any similar issues.